Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial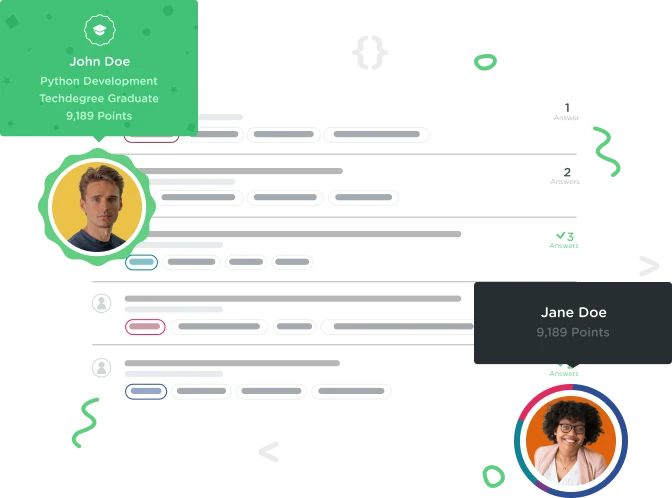

Arsalan Ahadi
7,626 PointsNeed help with the extra credit Challenge
Please Help!...I can't figure out how to fix my If statement at the end to print out " 'Input' Not Found" ,It automatically evaluates to true despite the condition and shows " 'Input' Not Found" even if the condition is false. Also the screen is not clearing up when I put a new input in the prompt.
var students = [
{
name: "Arsalan",
track: "Front End Development",
achievements: 300,
points:4000
},
{
name: "Arsalan",
track: "Wordpress",
achievements: 500,
points:3000
},
{
name: "Shane",
track: "Front End Development",
achievements:300,
points: 5000
},
{
name: "Paul",
track: "Full Stack JavaScript",
achievements:30,
points:100
},
{
name: "Dillen",
track: "Android development",
achievements: 10,
points: 50
},
];
Main Code below
var student;
var message = "";
var search;
function print(message){
var outPutDiv= document.getElementById("output");
outPutDiv.innerHTML += message;
}
//Returns the report of specific student
function getReport(student){
var report ="<h2>Student: "+student.name+"</h2>";
report +="<p>Track: "+student.track+"</p>";
report +="<p>Achievement: "+student.achievements+"</p>";
report +="<p>Points: "+student.points+"</p>";
return report;
}
//Returns the report of all students
function allStudentsReport(){
for(var i = 0; i < students.length; i++){
student = students[i];
message +="<h2>Student: "+student.name+"</h2>";
message +="<p>Track: "+student.track+"</p>";
message +="<p>Achievement: "+student.achievements+"</p>";
message +="<p>Points: "+student.points+"</p>";
}
return message;
}
do{
search = prompt("Please enter the name of the student (Type 'quit' to end the search and 'list' to show the whole record");
//If the input is equal to "quit" then exit the loop
if(search === null || search.toLowerCase() === "quit"){
break;
}
search = search.toLowerCase();
//Iterates through the Array
for(var i = 0; i < students.length; i++){
student = students[i];
/*
1.Checks the user input and prints the specified student's record
2.If the input is equal to "list" then it prints the whole record of the students
*/
if (search === student.name.toLowerCase()) {
message = getReport(student);
print(message);
}else if(search === "list"){
print(allStudentsReport());
}
}
//Checks if the user Input is not equal to any key and prints "Not Found"
if(student.name.toLowerCase !== search){
print(search + " Not found");
}
message = "";
}while(true)
2 Answers

Aaron Pedersen
5,856 PointsHi Arsalan.
Your screen is not clearing with each new input in the prompt because of the following code:
outPutDiv.innerHTML += message;
It should instead be
outPutDiv.innerHTML = message;
without the + operator. As it is, you are adding a string to the var outPutDiv every time the function is called without erasing what is in that variable from the first function call, hence the screen not clearing. However, if you change this line of code, it will let manifest other problems that are a result of other issues with the code and how it loops, which pertains to your first question about the last 'if' statement always evaluating to true.
That 'if' statement will not evaluate to true if you enter the last name in your list. The reason is because the 'student' variable is always going to be set on the last record of your student list because the 'for' loop ends on the last record. Try entering "Dillen" in the prompt and you will see that 'Dillen Not found' does not print. There are multiple ways to fix this, one simple way is to change the conditional statement inside the 'if' statement to (message === "") so that the last couple lines of code are
if(message === ""){
print(search + " Not found");
}
message = "";
thus checking whether the message variable is still an empty string, which would indicate no student was found and the user did not type 'list' or 'quit' in the prompt.
Loop logic is also getting applied when a user enters 'list' in the prompt. The whole student list prints out with every iteration of the for loop because of the placement of the code. The 'else if' statement that checks if the user typed 'list' should be outside of the 'for' loop, whether before or after the loop doesn't make a difference in terms of functionality. It can look like the following, which is the same as you have it except not an 'else if' statement:
if(search === "list"){
print(allStudentsReport());
}
Another manifestation of code error from changing the print function is multiple entries no longer displaying. After making the above changes, when a user types 'Arsalan' in the prompt only the second entry will show. This is easily fixed as well. When the program iterates through the 'for' loop looking for a match between the student name and the user prompt entry it will want to print a message every time it finds a match. The first match it finds will set the message variable equal to one student's info and send it to be printed, but the loop will continue iterating through the list of students, usually not even entering the 'if' statement because the condition will be false. However, when it is true for the second time because of two list entries using the same student name, the 'message' variable will still contain the information from the first student because it hasn't been set back to an empty string (that doesn't happen until after the 'for' loop is finished iterating). So, we can simply change
message = getReport(student);
to
message += getReport(student);
With this change, instead of replacing the information from the first student in the 'message' variable with the information from the second student, the program will add the second student's info to the message variable (In both cases, the matched student info was sent to the 'getReport' function for formatting and then returned to the 'message' variable). This updated 'message' variable will now be sent to the 'print' function, which in the process will clear any previous information sent to the 'print' function because of the change we made earlier.
Here is the code block with the changes made:
var student;
var message = "";
var search;
function print(message){
var outPutDiv= document.getElementById("output");
outPutDiv.innerHTML = message;
}
//Returns the report of specific student
function getReport(student){
var report ="<h2>Student: "+student.name+"</h2>";
report +="<p>Track: "+student.track+"</p>";
report +="<p>Achievement: "+student.achievements+"</p>";
report +="<p>Points: "+student.points+"</p>";
return report;
}
//Returns the report of all students
function allStudentsReport(){
for(var i = 0; i < students.length; i++){
student = students[i];
message +="<h2>Student: "+student.name+"</h2>";
message +="<p>Track: "+student.track+"</p>";
message +="<p>Achievement: "+student.achievements+"</p>";
message +="<p>Points: "+student.points+"</p>";
}
return message;
}
do{
search = prompt("Please enter the name of the student (Type 'quit' to end the search and 'list' to show the whole record");
//If the input is equal to "quit" then exit the loop
if(search === null || search.toLowerCase() === "quit"){
break;
}
search = search.toLowerCase();
//If the input is equal to "list" then it prints the whole record of the students
if(search === "list"){
print(allStudentsReport());
}
//Iterates through the Array
for(var i = 0; i < students.length; i++){
student = students[i];
//Checks the user input and prints the specified student's record
if (search === student.name.toLowerCase()) {
message += getReport(student);
print(message);
}
}
//Checks if the user Input is not equal to any key and prints "Not Found"
if(message === ""){
print(search + " Not found");
}
message ="";
}while(true)
I hope this helps.
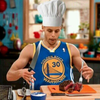
0yzh
17,276 PointsHi Arsalan,
On your last if statement, you have:
if(student.name.toLowerCase !== search){
print(search + " Not found");
}
You need to add parenthesis at the end of the toLowerCase method, like this:
if(student.name.toLowerCase() !== search){ // <--- Added ()
print(search + " Not found");
}
Arsalan Ahadi
7,626 PointsArsalan Ahadi
7,626 PointsThanks for the in depth explanation, it helped me a lot.