Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial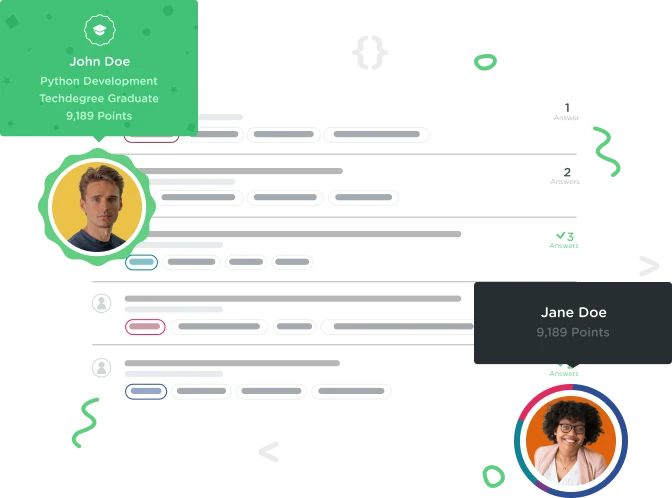

Jing Zhou
2,162 PointsNeed Help with this Word count problem! Been working on this challenge for hours
Been working with this challenge for hours. Just can't pass this part. The system said It can't get the expected outcome and be sure you're lowercasing the string and splitting on all whitespace!" Please advise! Thank you.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
dictionary={}
word_read = []
string_list = string.lower().split()
for word in string_list:
if word in word_read:
dictionary [word] +=1
else:
dictionary[word] = 1
return dictionary
2 Answers
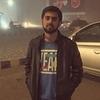
zainsra7
3,237 PointsI have 0 knowledge of python language as my base is mainly Java and I'm learning Javascript/FullStack here on Treehouse.
But still I can figure your code out and solve this problem as it's a basic one, you need to count each word in the list and then return the dictionary which contains key and value pairs where key refer to each word in the string and value means how many times it gets repeated in the string.
What I understood from your solution is that,
- You create an empty dictionary.
- You create an empty list of words
- You split the string on white spaces and covert them to lowerCase and store each individual word in string_list.
You then check for each word in string_list and if it's present in your current word list or not. If it's present then you increment the count of it in dictionary, otherwise you put 1 as the count of that specific word in dictionary.
But here are you missing one crucial point
You're not updating word_list at all and at each point you're basically checking each word from string_list in an empty word_list.
I tried to run your code in online compiler and it gives output as every individual word being 1, i.e
{'am': 1, 'sam': 1, 'it': 1, 'not': 1, 'i': 1, 'do': 1, 'like': 1}
I tried to solve it by inserting only a single line of code after else statement :
word_read += word
Final Code:
def word_count(string):
dictionary={}
word_read = []
string_list = string.lower().split()
for word in string_list:
if word in word_read:
dictionary [word] +=1
else:
word_read += word
dictionary[word] = 1
return dictionary
print(word_count("I do not like it Sam I Am"));
and it gives following output on online compiler :
{'am': 1, 'sam': 1, 'it': 1, 'not': 1, 'i': 2, 'do': 1, 'like': 1}
I hope it answers your question, Happy Coding !

Kaisa Hai
44 PointsYou are close enough. You're just forgetting to add the read word to the word_read list. Do that after the else block and you'll be fine. Here's the corrected code,
def word_count(string):
dictionary = {}
word_read = []
string_list = string.lower().split()
for word in string_list:
if word in word_read:
dictionary[word] +=1
else:
dictionary[word] = 1
word_read.append(word)
return dictionary
Jing Zhou
2,162 PointsJing Zhou
2,162 PointsHi,
Thank you for your answer! It is very comprehensive and easy to understand. I copied your final code and deleted the last line but it still didn't pass the challenge. It is so strange.