Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial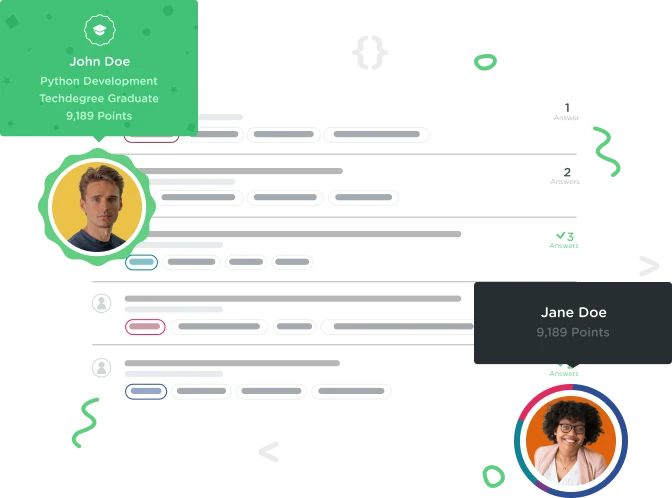

Anthony Lopez
963 PointsNeed to reverse a list and count every even index!
Here is the question:
You're on fire! Last one and it is, of course, the hardest.
Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse.
For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1].
You can do it!
Is there a reason why the bottom function is not passing? If you were to run range(20) through my function you would get back 19, 17, 15 etc. all the way down to 1, which are the correct even index's.
Not sure why it's saying that it didn't get the right values.
Thanks!
def first_4(lists):
create_list = list(lists)
solved = create_list[:4]
return solved
def first_and_last_4(new_list):
complex_list = list(new_list)
solved1 = complex_list[:4]
solved2 = complex_list[-4:]
return solved1 + solved2
def odds(iterable):
odds_list = list(iterable)
solved3 = odds_list[1::2]
return solved3
def reverse_evens(last):
last_list = list(last)
solved4 = last_list[::-2]
return solved4
1 Answer
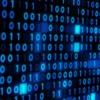
Alexander Davison
65,469 Points[::-2]
doesn't always return reverse evens. Sometimes, it will return reverse odds!
For example:
[1, 2, 3][::-2] # This will return [2], which isn't correct :/
[1, 2, 3, 4, 5][::-2] # This will return [4, 2] :/
# etc...
Also, you are coercing the input iterable into a list specifically. Iterables can be of many types, not just list
!
So, instead of [::-2], try making it a two-step process, first you get the evens, then you reverse it:
def reverse_evens(iter):
evens = iter[::2]
return evens[::-1]
Or, if you want to be super fancy, and merge it into two lines:
def reverse_evens(iter):
return iter[::2][::-1]

Anthony Lopez
963 PointsAh I see! That makes some sense Alexander thank you.

Anthony Lopez
963 PointsHey Alexander,
I actually have a question about this one. Your solution works using the two step process, but if I tried to reverse it first and then get the evens it doesn't work.
For example: def reverse_evens(iter): evens = iter[::-1] return evens[::2]
Shouldn't this yield the same thing in the end? I just changed which order I executed the steps.
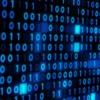
Alexander Davison
65,469 PointsReversing first then getting the evens is different than the other way around.
For example...
(Pretend I'm in the Python Shell)
>>> my_list = [1, 2, 3, 4]
>>> my_list[::2][::-1] # Correct way
[3, 1]
>>> my_list[::-1][::2] # Incorrect way
[4, 2]
As you can see, they produce different results. The challenge expects you to first get the evens, then reverse it.

Anthony Lopez
963 PointsOh ok that makes sense. Thanks Andrew! Appreciate the help!
When I read it, the way I understood it was that it was asking for the value in the even position, not the value that is an even.
Tristan Magpantay
Full Stack JavaScript Techdegree Student 14,032 PointsTristan Magpantay
Full Stack JavaScript Techdegree Student 14,032 PointsI've already seen this question this week. I wounder if it could be a bug that should be reported to the support team