Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial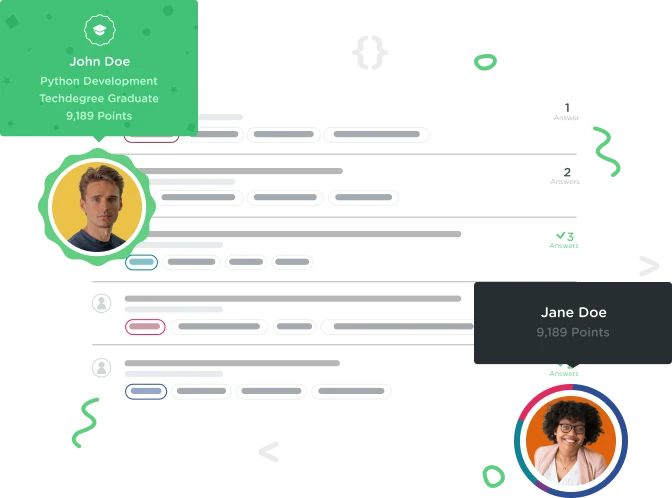

Arek Bochenski
11,893 PointsNo subtracting. Is this a valid solution?
Hi Guys,
Dave's solution is to subtract the bottom number from the top number, do the math, then add the top number to the result.
I did it a bit differently. I seem to be getting desired results, but is this a valid solution?
var startDie = prompt('What is your starting number?');
var endDie = prompt('What is your ending number?');
var strRolls = prompt('How many rolls?');
var n1die = parseInt(startDie);
var n2die = parseInt(endDie);
var rolls = parseInt(strRolls);
var total = 0;
for (var x = 1; x<=rolls; x++) {
var roll = Math.floor (Math.random() * n2die) + n1die;
document.write ('<p>You rolled ' + roll + '</p>');
total+= roll;
};
document.write('The sum of these rolls is: ' + total);
Thank you.
2 Answers
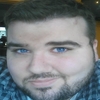
Marcus Parsons
15,719 PointsHey Arek,
That is a nicely done program, but unfortunately, the range it produces isn't quite right. Your program doesn't produce a range between a given minimum and maximum. It produces a range that is between "n1die" and ((n2die - 1) + n1die). You'll see exactly what I mean if you plug in a small range such as 5 and 8 and roll several rolls. It will produce values outside of that range.
A look at the minimum value: Math.random() can reach a minimum of 0, and so that means your inside floor statement can go to 0 and then the outside will be added, making the minimum be: 0 + n1die which equals "n1die".
A look at the maximum value: Math.random() can reach a maximum of approximately 0.9999... so your inside is going to be (n2die * 0.9999) which will result in (n2die - 1) because of the floor statement. And then the outside is added making your maximum be: ((n2die - 1) + n1die).
In order to get a proper range, you have to use the formula:
var roll = Math.floor (Math.random() * (n2die - n1die + 1)) + n1die;

Arek Bochenski
11,893 PointsMarcus,
Thanks very much for your detailed answer. I only tested my code with numbers varying from 1-10, 1-4 etc. and it seemed fine.
After meditating on your answer and trying it out with a higher starting number I understand it now.
Thank you!
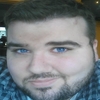
Marcus Parsons
15,719 PointsYou're very welcome. The reason why you were seeing correct code comes from that "-1" within the maximum of your code and the fact that you started with 1. They cancelled each other out so that the range would work so long as the starting number is 1. Another interesting case is starting with 0, which results in a maximum of "n2die - 1". So, if you roll any X amount of times the numbers 0 and 10, you will never get 10 in any of your rolls. And if you start with some number and do the 2nd roll to be 0, you'll always get the first number for every roll. And then from 2 onwards, the mathematics I show you above work.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsBtw, to test that my mathematics are correct on your minimum and maximum values, plug in a number such as 5 for your first number and 8 for your second number. Do as many rolls as you'd like, such as 1000 or even 5000. Then, take a look at all of the random values displayed. You'll see that you never get lower than the bottom number of 5, and you'll never get higher than (8+5-1) = 12. All of the numbers rolled will not be between 5 and 8 but between 5 and 12, inclusive.