Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial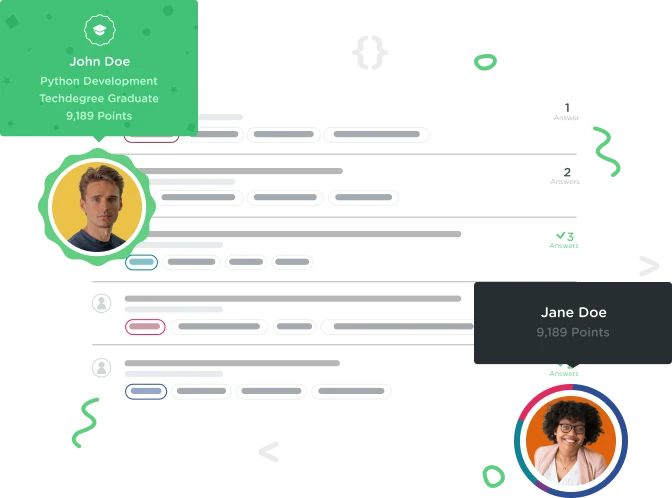

Puneet Mahal
Courses Plus Student 983 PointsNot able to retrieve weather data from forecast.io
As the title says, I'm unable to retrieve data from the site and I can't figure out why. Any help would be greatly appreciated
Here is my main activity code:
package com.example.puneetmahal.stormy;
import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log;
import java.io.IOException;
import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//put api key that you got from URL here, break is down into the following components
String apiKey = "7ec60e1b37c24a1742a89ad7213654b3";
double latitude =37.826;
double longitude = -122.423;
String forecastURL = "https://api.forecast.io/forecast" + apiKey + "/" + latitude + "." + longitude;
//main client object
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder() //Build request with the url
.url(forecastURL) //Pass in the URL
.build(); //actually builds it
//Need a call obj, need to put this request inside a call object.
Call call = client.newCall(request); // we have an execute method from the call class
//and it returns a response object. Lets put that in a var
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
if (response.isSuccessful()) {
Log.v(TAG, response.body().string()); //around 7 min mark on Making an HTTP Get request.
}
} catch (IOException e) {
Log.e(TAG, "Exception caught ", e);
}
}
});
}
}
And here is the logcat with filter "show only selected application":
04-20 19:53:29.454 2366-2366/com.example.puneetmahal.stormy I/art: Not late-enabling -Xcheck:jni (already on) 04-20 19:53:29.510 2366-2366/com.example.puneetmahal.stormy E/art: Failed to send JDWP packet APNM to debugger (-1 of 87): Broken pipe 04-20 19:53:29.511 2366-2372/com.example.puneetmahal.stormy I/art: Debugger is no longer active 04-20 19:53:29.515 2366-2366/com.example.puneetmahal.stormy W/System: ClassLoader referenced unknown path: /data/app/com.example.puneetmahal.stormy-1/lib/x86 04-20 19:53:29.556 2366-2381/com.example.puneetmahal.stormy D/OpenGLRenderer: Use EGL_SWAP_BEHAVIOR_PRESERVED: true 04-20 19:53:29.596 2366-2381/com.example.puneetmahal.stormy I/OpenGLRenderer: Initialized EGL, version 1.4 04-20 19:53:29.631 2366-2381/com.example.puneetmahal.stormy W/EGL_emulation: eglSurfaceAttrib not implemented 04-20 19:53:29.631 2366-2381/com.example.puneetmahal.stormy W/OpenGLRenderer: Failed to set EGL_SWAP_BEHAVIOR on surface 0xaaa07720, error=EGL_SUCCESS
5 Answers

aakarshrestha
6,509 PointsYour String forecastURL setup is not correct. You have: String forecastURL = "https://api.forecast.io/forecast" + apiKey + "/" + latitude + "." + longitude;
Change it to: String forecastURL = "https://api.forecast.io/forecast" + apiKey + "/" + latitude + "," + longitude;
Hope it helps!
Happy coding!

Puneet Mahal
Courses Plus Student 983 PointsSo I tried using genymotion as well, here is the logcat from that emulator:
04-21 23:41:45.589 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: Late-enabling CheckJNI 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to find class referenced in signature (Landroid/view/SearchEvent;) 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.view.Window$Callback.onSearchRequested, referenced from method android.support.v7.view.WindowCallbackWrapper.onSearchRequested 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve interface method 15123: Landroid/view/Window$Callback;.onSearchRequested (Landroid/view/SearchEvent;)Z 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x72 at 0x0002 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.view.Window$Callback.onWindowStartingActionMode, referenced from method android.support.v7.view.WindowCallbackWrapper.onWindowStartingActionMode 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve interface method 15127: Landroid/view/Window$Callback;.onWindowStartingActionMode (Landroid/view/ActionMode$Callback;I)Landroid/view/ActionMode; 04-21 23:41:45.601 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x72 at 0x0002 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.view.ViewGroup.onRtlPropertiesChanged, referenced from method android.support.v7.widget.Toolbar.onRtlPropertiesChanged 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve virtual method 15022: Landroid/view/ViewGroup;.onRtlPropertiesChanged (I)V 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x6f at 0x0007 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.content.res.TypedArray.getChangingConfigurations, referenced from method android.support.v7.widget.TintTypedArray.getChangingConfigurations 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve virtual method 421: Landroid/content/res/TypedArray;.getChangingConfigurations ()I 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x6e at 0x0002 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.content.res.TypedArray.getType, referenced from method android.support.v7.widget.TintTypedArray.getType 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve virtual method 443: Landroid/content/res/TypedArray;.getType (I)I 04-21 23:41:45.613 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x6e at 0x0002 04-21 23:41:45.645 1209-1212/com.example.puneetmahal.stormy D/dalvikvm: GC_CONCURRENT freed 201K, 3% free 10926K/11207K, paused 10ms+1ms, total 20ms 04-21 23:41:45.645 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.content.res.Resources.getDrawable, referenced from method android.support.v7.widget.ResourcesWrapper.getDrawable 04-21 23:41:45.645 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve virtual method 384: Landroid/content/res/Resources;.getDrawable (ILandroid/content/res/Resources$Theme;)Landroid/graphics/drawable/Drawable; 04-21 23:41:45.645 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x6e at 0x0002 04-21 23:41:45.645 1209-1209/com.example.puneetmahal.stormy I/dalvikvm: Could not find method android.content.res.Resources.getDrawableForDensity, referenced from method android.support.v7.widget.ResourcesWrapper.getDrawableForDensity 04-21 23:41:45.645 1209-1209/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve virtual method 386: Landroid/content/res/Resources;.getDrawableForDensity (IILandroid/content/res/Resources$Theme;)Landroid/graphics/drawable/Drawable; 04-21 23:41:45.645 1209-1209/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x6e at 0x0002 04-21 23:41:45.705 1209-1209/com.example.puneetmahal.stormy D/libEGL: loaded /system/lib/egl/libEGL_genymotion.so 04-21 23:41:45.713 1209-1209/com.example.puneetmahal.stormy D/libEGL: loaded /system/lib/egl/libGLESv1_CM_genymotion.so 04-21 23:41:45.713 1209-1209/com.example.puneetmahal.stormy D/libEGL: loaded /system/lib/egl/libGLESv2_genymotion.so 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to find class referenced in signature (Ljava/nio/file/Path;) 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to find class referenced in signature ([Ljava/nio/file/OpenOption;) 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy I/dalvikvm: Could not find method java.nio.file.Files.newOutputStream, referenced from method okio.Okio.sink 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve static method 16257: Ljava/nio/file/Files;.newOutputStream (Ljava/nio/file/Path;[Ljava/nio/file/OpenOption;)Ljava/io/OutputStream; 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x71 at 0x000a 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to find class referenced in signature (Ljava/nio/file/Path;) 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to find class referenced in signature ([Ljava/nio/file/OpenOption;) 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy I/dalvikvm: Could not find method java.nio.file.Files.newInputStream, referenced from method okio.Okio.source 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy W/dalvikvm: VFY: unable to resolve static method 16256: Ljava/nio/file/Files;.newInputStream (Ljava/nio/file/Path;[Ljava/nio/file/OpenOption;)Ljava/io/InputStream; 04-21 23:41:45.729 1209-1223/com.example.puneetmahal.stormy D/dalvikvm: VFY: replacing opcode 0x71 at 0x000a 04-21 23:41:45.733 1209-1209/com.example.puneetmahal.stormy W/EGL_genymotion: eglSurfaceAttrib not implemented 04-21 23:41:45.757 1209-1209/com.example.puneetmahal.stormy D/OpenGLRenderer: Enabling debug mode 0 04-21 23:41:45.793 1209-1212/com.example.puneetmahal.stormy D/dalvikvm: GC_CONCURRENT freed 244K, 4% free 11079K/11463K, paused 10ms+0ms, total 12ms 04-21 23:41:45.805 1209-1209/com.example.puneetmahal.stormy D/OpenGLRenderer: TextureCache::get: create texture(0xb8792388): name, size, mSize = 2, 4096, 4096
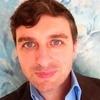
Arsene Lavaux
4,342 PointsSame issue... Are we the only ones?

aakarshrestha
6,509 PointsWrite down your forecastURL string value?
It should work: String forecastURL = "https://api.forecast.io/forecast" + apiKey + "/" + latitude + "," + longitude;
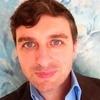
Arsene Lavaux
4,342 PointsThe string is fine, I actually get a JSON back. On debugger it looks like the problem is in parsing the JSON data into my model for some reasons. Thanks anyway!

aakarshrestha
6,509 PointsGo to Build/Rebuild Project or Clean Project.
Let me know.

Puneet Mahal
Courses Plus Student 983 PointsNo difference
Puneet Mahal
Courses Plus Student 983 PointsPuneet Mahal
Courses Plus Student 983 PointsStill getting the same error