Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial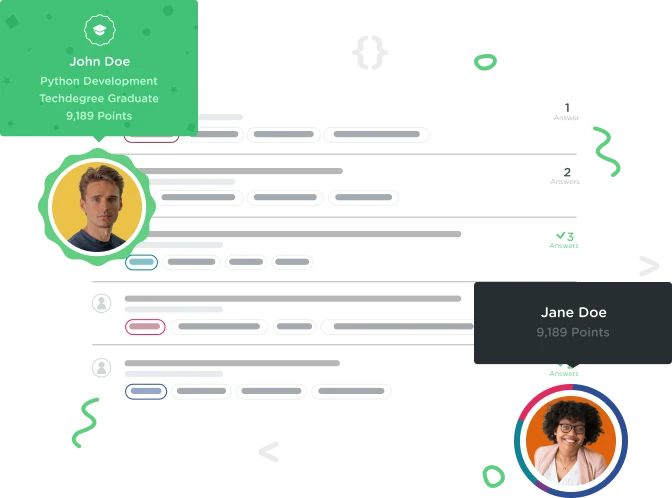

Caleb Pitts
967 PointsNot correct
What am I doing wrong here?
def loopy(items):
# Code goes here
for items in loopy:
if(items == 'STOP'):
break
else:
print(items)
loopy("Hello", "Bye", "STOP", "a")
2 Answers
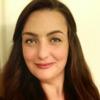
Jennifer Nordell
Treehouse TeacherHi there Caleb Pitts! You've got the right idea and even the right syntax! It's the naming of your variables that's throwing the whole thing off. Also, you don't need to call the loopy function yourself. Treehouse is going to do that.
I'm going to show you my code and then explain what yours is currently doing:
def loopy(items):
# Code goes here
for item in items:
if(item == 'STOP'):
break
else:
print(item)
You have the function loopy defined. And it's accepting an iterable named items. But when you start your for loop you say for every items in loopy. And loopy isn't an iterable, it's a function. Your iterable is items. So this is how I did it. For every item in the items iterable, if the item is equal to STOP then break. Otherwise print the item.
Hope this makes sense and is somewhat helpful!
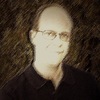
Jason Anders
Treehouse Moderator 145,860 PointsHey Caleb,
You're more or less on the right track, but there are a few things.
First is the
for loop
. You need to assign a temporary variable to the loop and iterate over the items passed into the function. You can't actually iterate through the function itself. The general convention is to use the singular version of the variable name being pass in.With that in mind, the variable checking for "stop" needs to be updated to reflect the new temporary variable.
And then, the
print
statement in the else clause will also need updating.Lastly, the challenge did not ask you to call the function, so that whole line needs to be deleted.
Below is the corrected code for your reference. I used "item" with no "s" for the temporary variable being used in the loop. I hope it gives you that "ah - ha" moment.
def loopy(items):
# Code goes here
for item in items:
if(item == 'STOP'):
break
else:
print(item)
Keep Coding! :)

Caleb Pitts
967 PointsThank you!
Caleb Pitts
967 PointsCaleb Pitts
967 PointsThank you!