Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial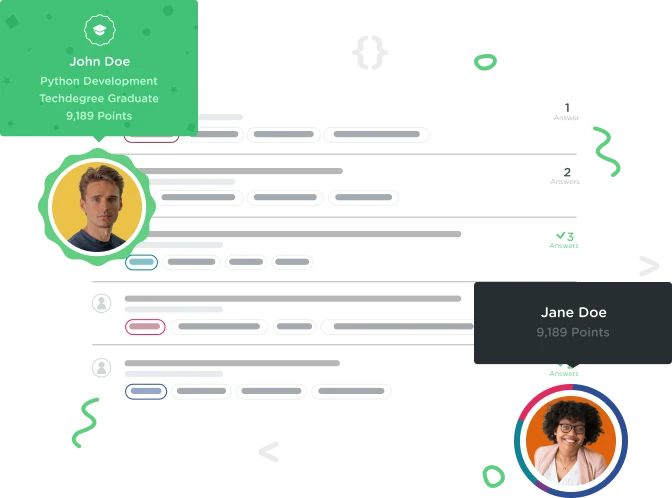

David Ly
1,858 PointsNot fully displaying questions and answers that were answered correct and wrong
I'm not sure what is wrong with my code and my head hurts :( Can someone help me?
var questionsCorrect = 0;
var question;
var answer;
var response;
var correct = [];
var wrong = [];
var html;
var questions = [
["How many stars does the US flag have?", "50"],
["what is 4 x 4?", "16"],
["What is a legendary fly creature that breathes fire?", "dragon"],
["what has 8 legs and a red hour glass on its belly?", "black widow"],
["In the movie oceans 11 what cocktail is Brad pitt eating?", "shrimp"]
];
//This function is created to create an ordered list to store the questions answered right and wrong.
function buildList(arr){
// this creates and ordered list 1., 2., 3., etc. If I used "ul" instead it would be bullet points instead of numbers
var listHTML = "<ol>";
for(var i = 0; i < arr.length; i += 1){
// uses a for loop to create 1 html list item "li" tag containging whath is stored in the array in the specified index position
//close ol tag return string
listHTML += "<li>" + arr[i] + "</li>";
return listHTML;
}
listHTML += "</ol>";
return listHTML;
}
function print(message) {
//use currently instead of document.write what it does is locate the ID name in this case "output" and print it in that area
var outputDiv = document.getElementById("output");
outputDiv.innerHTML = message;
}
for ( var i = 0; i < questions.length; i += 1){
//the question is located at questions
question = questions[i][0];
//the answers are located next to the questions in the array
answer = questions [i][1];
//the reponse will be a prompt for the question then it is stored back in the response
response = (prompt(question));
if (response === answer){
questionsCorrect += 1;
//use the push method to add question at the end of array
correct.push(question);
}else{
wrong.push(question);
}
}
if (questionsCorrect === 5){
print("Congratulations you got " + questionsCorrect + " questions correct. You earned a Gold star");
}else if (questionsCorrect >= 3){
print("Congratulations you got " + questionsCorrect + " questions correct. You earned a Silver star");
}else if (questionsCorrect >= 1){
print("Congratulations you got " + questionsCorrect + " questions correct. You earned a Bronze star");
}else{
print("Sorry you got " + questionsCorrect + " questions correct. Womp Womp....");
}
html += "<h2> You got these questions correct:</h2>";
html += buildList(correct);
html += "<h2> You got these questions wrong<h2>";
html += buildList(wrong);
print(html);
2 Answers

Erik Nuber
20,629 Pointsjust a suggestion, as someone could get all questions right or all questions wrong, you might want to test that. Not necessary but, as you are returning a <ul></ul> if nothing is there, you might want to skip this step if there is nothing in a given array.
if (correct.length > 0) {
html += "<h2> You got these questions correct:</h2>";
html += buildList(correct);
}
if (wrong.length > 0) {
html += "<h2> You got these questions wrong<h2>";
html += buildList(wrong);
}
print(html)
Your issue is in the buildList function
you have a return listHTML within the loop so it isn't working properly.
function buildList(arr){
console.log(arr);
var listHTML = "<ul>";
for(var i = 0; i < arr.length; i++){
listHTML += "<li>" + arr[i] + "</li>";
// return listHTML; remove this line and it will work fine.
}
listHTML += "</ul>";
return listHTML;
}

Andrew McPheron
14,290 PointsI second what Erik said about trying to return your listHTML too soon. Get rid of the return line here:
listHTML += "<li>" + arr[i] + "</li>";
return listHTML;
There are a few other issues. Your medal awarding unit doesn't appear because it's being overwritten by the new print command. A super easy fix for this is to change the print function to use += instead of =, like so:
outputDiv.innerHTML += message;
Finally, you'll still have that pesky 'undefined' string because your html variable at the top is undefined when you add stuff to it. An empty string will clear that right up, just define the variable as:
var html = '';
Hope all that helps!