Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial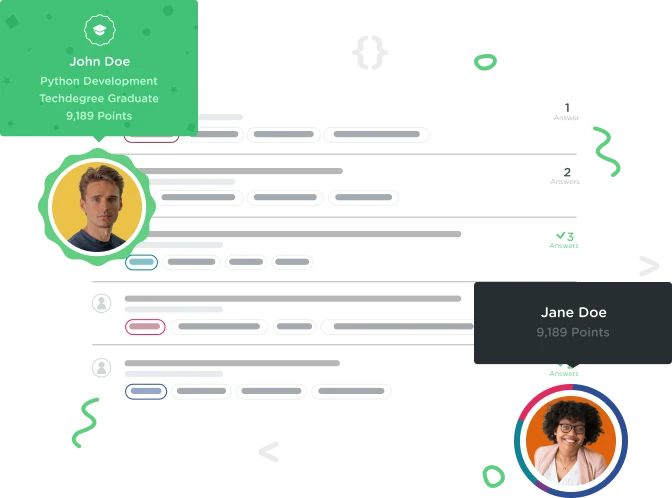

Alex Diaz
4,930 PointsNot getting correct output
Could anyone please help me with finishing this challenge, I really don't know what else to do. Thank you!
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(topics):
results = []
for course, course_topics in COURSES.items():
results.append(topics.intersection(course))
# course_topics is the set which you can use for this question
return results
2 Answers
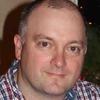
jonlunsford
15,480 PointsAlex:
I'm sure there are a number of ways to complete this task and it looks like you are on the right path. The problem is that each time through the loop your code is appending to the results[] list you set up. So if you passed in a set with one element like: topics = {"Python"}, the code would produce this result.
[set(), set(), {"Python"}, set()]
NOTE that the ordering may be slightly different each time you run the code because dictionaries store their elements unordered.
Because you are appending to the list for each course in the dictionary (Python Basics, Java Basics...) you get four values in your list. The set() values above represent empty sets that are returned from your loop where "Python" did not match any value in the course_topics set.
What you need to do is add a conditional to only append when a non-empty set is returned. For instance.
def covers(topics):
results = []
for course, course_topics in COURSES.items():
match_set = topics.intersection(course_topics)
# only append to results[] if the returned set is not empty
if len(match_set):
results.append(course)
return results

Umesh Ravji
42,386 PointsHi Alex, you are looking to see which courses contain any of the topics (using intersection), which is great. However, you have to add the course to the results list and not the topics in the sets. The code below can be shortened, but I think it's easier to understand as is.
def covers(topics):
results = []
for course, course_topics in COURSES.items():
overlap = topics.intersection(course_topics)
if overlap:
results.append(course)
return results