Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial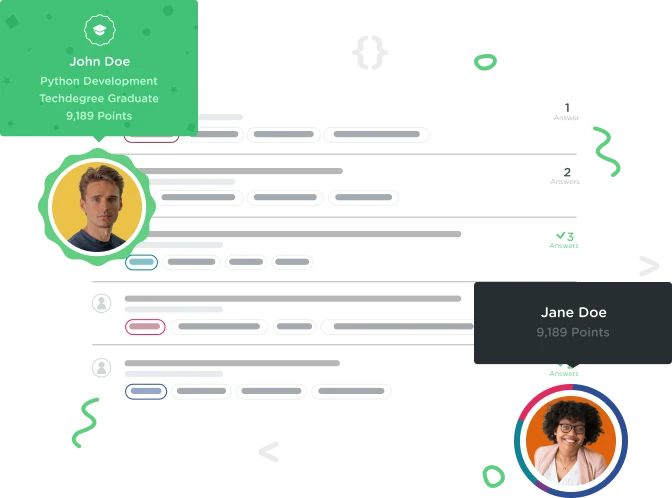

Nick Shannon
10,535 PointsNot quite sure where to start... this is what I made but I know it's not correct. Any help would be appreciated.
Great work! OK, you're doing great so I'll keep increasing the difficulty.
For this step, make another new function named courses that will, again, take the dictionary of teachers and courses.
courses, though, should return a single list of all of the available courses in the dictionary. No teachers, just course names!
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
treehouse_teachers = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def num_teachers(treehouse_teachers):
return len(treehouse_teachers)
def num_courses(my_dict):
count = 0
for teacher in my_dict:
count += len(my_dict[teacher])
return count
def courses(treehouse_teachers):
courses = []
for values in treehouse_teachers.values():
courses.append(treehouse_teachers.values())
1 Answer
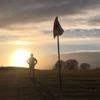
Stuart Wright
41,120 PointsYou're actually not too far off, you have the right idea but then it goes a bit wrong at this line:
courses.append(treehouse_teachers.values())
The first thing to note is that instead of accessing 'treehouse_teachers.values()' inside your loop, you should just access 'values', which is the list of courses by the current teacher in the loop. The next thing to note is that by using the append method, you'll end up with a list of lists. You might want to check out the extend method instead, as it extends the current list with the new elements, without any nesting.
Oh, and you'll want to remember to return the courses variable :)
Christian A. Castro
30,501 PointsChristian A. Castro
30,501 PointsStuart Wright something like this courses.extend([teachers, courses]), am I right? I'm sorry for my ignorance.. but I still kinda lost
Stuart Wright
41,120 PointsStuart Wright
41,120 PointsHi Christian - courses.extend(values) is what I had in mind. Because on each iteration of your loop, the variable values is a list of courses, which you will add to your main list using the extend method.