Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial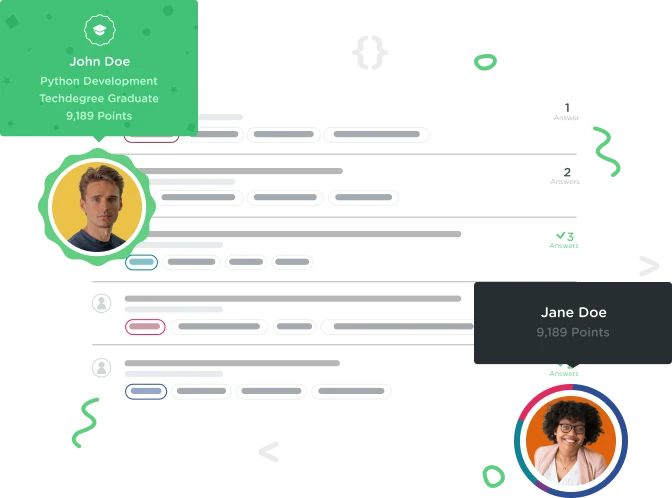

Clayton McDaniels
5,788 PointsNot sure how to approach the "Disemvowel" challenge
Not sure how to approach the "Disemvowel" challenge
def disemvowel(word):
vowels = ['a', 'A', 'e', 'E', 'i', 'I', 'o', 'O', 'u', 'U']
for letter in word:
if letter in vowels:
vowels.remove(word)
return word
3 Answers
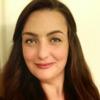
Jennifer Nordell
Treehouse TeacherHi there! I'm going to start by giving a hint. This might sound silly, but run this code in a workspace or on your local machine and send in the word "Treehouse". I think you'll find the results interesting. The problem is that the indexing of the iterable you're looking at changes when you mutate it. Short answer: don't mutate the thing you're iterating over. Maybe iterate over a copy?
That being said, there are other solutions that don't require the remove
function.
Hope this helps, but let me know if you're still stuck!
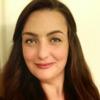
Jennifer Nordell
Treehouse TeacherHi there! I inadvertently ran code that another student had left in a previous question about the same challenge. They were also using the remove
function. Your code in its present state will not compile. I apologize for the confusion.
First and foremost, the remove
function is not available on a string, only on a list. You will first need to turn word
into a list if you want to use the remove
function. Secondly, you have this line:
vowels.remove(word)
#but you meant
word.remove(letter)
Third, you should not mutate the iterable you're looping over but rather make a copy and iterate over that as mentioned in my previous statement.
Finally, because you've turned this into a list you will need to convert the list back to a string by using the join
function, as the challenge expects a string to be returned.
Hope this helps!

Clayton McDaniels
5,788 Pointsdef disemvowel(word):
vowels = ['a', 'A', 'e', 'E', 'i', 'I', 'o', 'O', 'u', 'U']
word_list = list(word)
for letter in word:
if letter in vowels:
word_list.remove(letter)
word = ''.join(word_list)
return word

Clayton McDaniels
5,788 PointsMy code above worked.
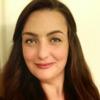
Jennifer Nordell
Treehouse TeacherYup! That's it! Well done.
Clayton McDaniels
5,788 PointsClayton McDaniels
5,788 PointsI'm still stuck.