Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial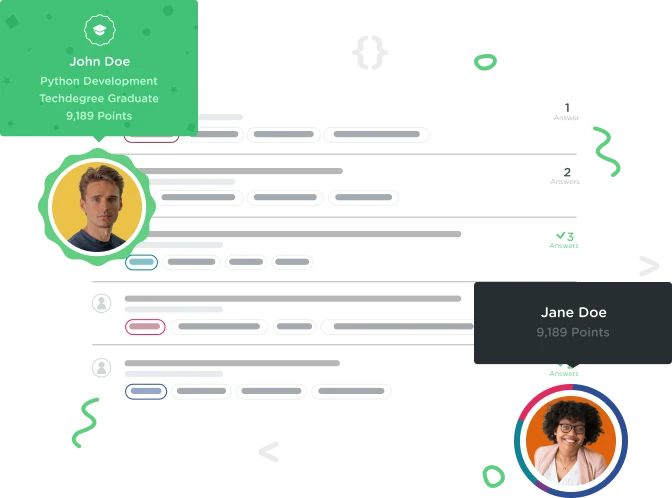

varun ganglani
4,665 PointsNot sure why my moves don't change the player position
not sure where I went wrong in the code but the player position doesn't change and the invalid move prompt comes up
1 Answer

varun ganglani
4,665 Pointsimport os import random
draw grid
pick random location for player
pick random location for monster
pick random location for the door
draw player on grid
take input for movement
move player, unless invalid move (past edges of grid)
check for win/loss
clear screen and redraw grid
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0), (0, 1), (1, 1), (2, 1), (3, 1), (4, 1), (0, 2), (1, 2), (2, 2), (3, 2), (4, 2), (0, 3), (1, 3), (2, 3), (3, 3), (4, 3), (0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
def clear_screen(): os.system('cls' if os.name == 'nt' else 'clear')
def get_locations(): return random.sample(CELLS, 3)
def move_player(player, move): x, y = player if move == 'LEFT': x -= 1 if move == 'RIGHT': x += 1 if move == 'UP': y -= 1 if move == 'DOWN': y += 1 return player
def get_moves(player): moves = ['UP', 'DOWN', 'LEFT', 'RIGHT'] x, y = player if x == 0: moves.remove("LEFT") if x == 4: moves.remove("RIGHT") if y == 0: moves.remove("UP") if y == 4: moves.remove("DOWN") return moves
monster, door, player = get_locations()
while True: valid_moves = get_moves(player) clear_screen() print("Welcome to the dungeon!") print("You're currently in room {}".format(player)) print("You can move {}".format(", ".join(valid_moves))) print("Enter 'Q' to quit")
move = input("> ").lower()
if move == 'q':
break
elif move in valid_moves:
player = move_player(player, move)
else:
print("\n** You ran into a wall! **\n")
continue
# good move? change player position
# bad move? dont change anything
# on the door? win
# on monster? lose
# otherwise, loop