Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial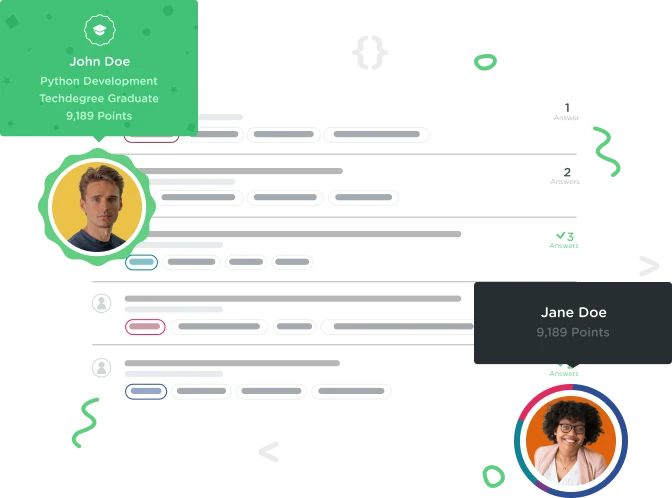
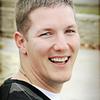
Brian Hankins
5,735 PointsNot understanding the function buildList(arr) portion.
So, I followed the instructors notes and everything printed out like it should. However, I'm really not understanding this portion of the code:
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i +=1) {
listHTML += '<li>' + arr[ i ] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
I understand that he's building up the html using ordered list and list items but...my confusion is why does he use 'arr' as the argument (hope my programming lingo is right) and then use arr[ i ]? Is [ i ] going towards the quiz array I created or something else?
Here is my full code below:
var quiz = [
['1', 'What is the biggest state in the US?', 'TEXAS'],
['2', 'What city is the Sears Tower located in?', 'CHICAGO'],
['3', 'Where is the worst state to live in the US?', 'ILLINOIS']
];
var answerCorrect = 0;
var answerIncorrect = 0;
var answer;
var html;
var correct = [];
var wrong = [];
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i +=1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
for (i = 0; i < quiz.length; i += 1) {
answer = prompt('Your question is... ' + quiz[i][1] );
answer = answer.toUpperCase();
if ( answer === quiz[i][2] ) {
answerCorrect += 1;
correct.push(quiz[i][1]);
} else {
answerIncorrect += 1;
wrong.push(quiz[i][1]);
}
}
html = "You got " + answerCorrect + " question(s) right.";
html += '<h2>You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
2 Answers
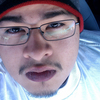
Chyno Deluxe
16,936 Pointsthe arr variable name IS the variable itself. Think of it as a placeholder. Any value that is passed into the buildList function then becomes the value of arr.
the values are being given here.
html += buildList(correct); //<-- List of correct answers
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong); //<-- List of wrong answers
as for arr[i]. arr becomes correct[i] and wrong[i] as buildList() is taking the arrays and cycles through them.
so If i was to answer two correctly and 1 incorrectly then it would look something like this.
["What is the biggest state in the US?", "What city is the Sears Tower located in?"] //correct array
["Where is the worst state to live in the US?"] //wrong array
in order to cycle through an array you would call an index of the array like so:
correct[0] // "What is the biggest state in the US?"
correct[1] // "What city is Sears Tower located in?"
wrong[0] //"Where is the worst state to live in the US?"
I hope this helps.
let me know if you still have questions
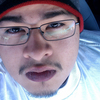
Chyno Deluxe
16,936 PointsWhat is happening is the buildList function is taking in an array as its argument. it is then cycling through all the items in the array (arr[i]) and building the html for the list of questions answered correctly..then the list of incorrectly answered questions.
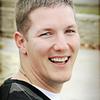
Brian Hankins
5,735 PointsOkay, I understand that now. But, where does the arr value come from? It's not assigned to any variable or in anywhere else. And secondly, where does the 'i' come from in [i]? What if there were multiple array's declared?
Maybe, I'm missing the whole picture by these questions?