Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial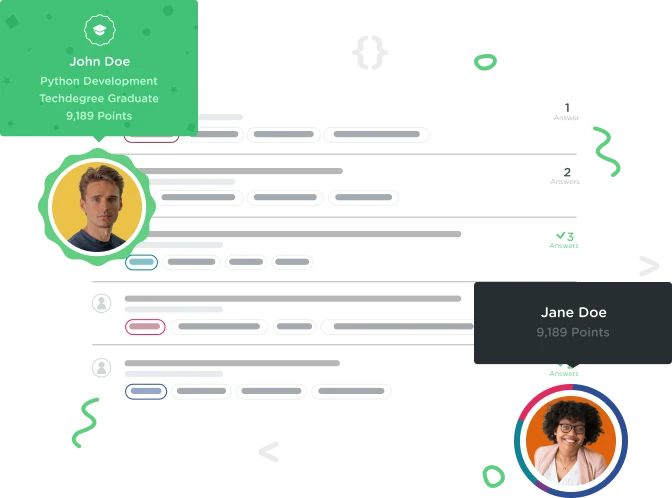

Steven Buchko
4,387 PointsNull Pointer Exception on Log In
java.lang.NullPointerException: Attempt to invoke virtual method 'com.parse.ParseHttpClient com.parse.ParsePlugins.restClient()' on a null object reference
I receive this error if I attempt to Log In without first visiting the Sign Up Activity. I tried enabling the local data store and initializing Parse in the Log In Activity (currently I do it in the Sign Up activity), but that seemed to cause other issues.
LogInActivity
package com.stevenbuchko.here;
import android.app.AlertDialog; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView;
import com.parse.LogInCallback; import com.parse.Parse; import com.parse.ParseException; import com.parse.ParseUser;
public class LogInActivity extends AppCompatActivity {
protected EditText mUsername;
protected EditText mPassword;
protected Button mLoginButton;
protected TextView mSignUpTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_log_in);
mUsername = (EditText) findViewById(R.id.usernameField);
mPassword = (EditText) findViewById(R.id.passwordField);
mLoginButton = (Button) findViewById(R.id.logInBtn);
mSignUpTextView = (TextView) findViewById(R.id.signUpText);
mSignUpTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(LogInActivity.this, SignUpActivity.class);
startActivity(intent);
}
});
mLoginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
username = username.trim();
password = password.trim();
if (username.isEmpty() || password.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(LogInActivity.this);
builder.setMessage(R.string.login_error_message)
.setPositiveButton(android.R.string.ok, null)
.setTitle(R.string.login_error_title);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
// Login
setProgressBarIndeterminate(true);
ParseUser.logInInBackground(username, password, new LogInCallback() {
@Override
public void done(ParseUser parseUser, ParseException e) {
setProgressBarIndeterminate(false);
if (e == null) {
// Success!
Intent intent = new Intent(LogInActivity.this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(LogInActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.login_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_log_in, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
SignUpActivity
package com.stevenbuchko.here;
import android.app.AlertDialog; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.EditText;
import com.parse.Parse; import com.parse.ParseException; import com.parse.ParseUser; import com.parse.SignUpCallback;
public class SignUpActivity extends AppCompatActivity {
protected EditText mUsername;
protected EditText mPassword;
protected EditText mEmail;
protected Button mSignUpButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sign_up);
mUsername = (EditText) findViewById(R.id.usernameField);
mPassword = (EditText) findViewById(R.id.passwordField);
mEmail = (EditText) findViewById(R.id.emailField);
mSignUpButton = (Button) findViewById(R.id.signUpBtn);
Parse.enableLocalDatastore(this);
Parse.initialize(this);
mSignUpButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
String email = mEmail.getText().toString();
username = username.trim();
password = password.trim();
email =email.trim();
if (username.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(R.string.sign_up_username_error_message)
.setTitle(R.string.sign_up_error_title)
.setPositiveButton(android.R.string.ok , null);
AlertDialog dialog = builder.create();
dialog.show();
} else if (password.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(R.string.sign_up_password_error_message)
.setTitle(R.string.sign_up_error_title)
.setPositiveButton(android.R.string.ok , null);
AlertDialog dialog = builder.create();
dialog.show();
} else if (email.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(R.string.sign_up_email_error_message)
.setTitle(R.string.sign_up_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
} else {
// create the user
setProgressBarIndeterminateVisibility(true);
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
newUser.setEmail(email);
newUser.signUpInBackground(new SignUpCallback() {
@Override
public void done(ParseException e) {
setProgressBarIndeterminateVisibility(false);
if (e == null) {
// success!
Intent intent = new Intent(SignUpActivity.this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.sign_up_error_title)
.setPositiveButton(android.R.string.ok , null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_sign_up, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}