Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial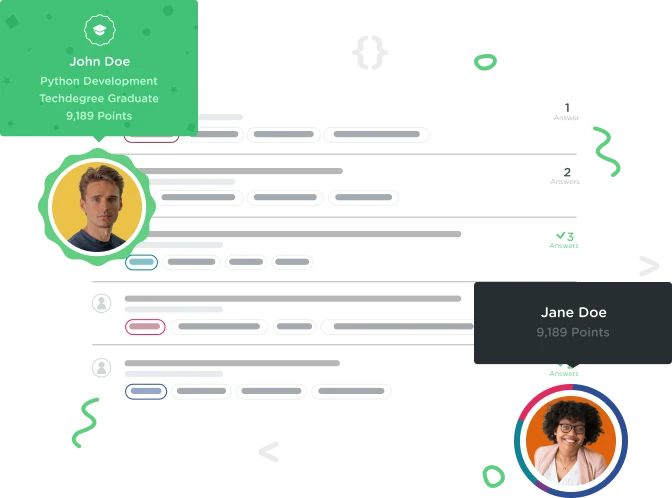
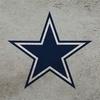
Anmol Gulati
13,991 PointsNumber Game App with Python
When I try catch the exception using the except block it gives me the following error when I try to add a non integer number :
Guess a number between 1 and 10: l
Traceback (most recent call last):
File "number_game1.py", line 9, in game
guess = int(input("Guess a number between 1 and 10: "))
ValueError: invalid literal for int() with base 10: 'l'
Traceback (most recent call last):
File "number_game1.py", line 30, in <module>
game()
File "number_game1.py", line 11, in game
print("Hey, {} is not a number huhh!!".format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
Here is my code:
import random
def game():
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("Hey, {} is not a number huhh!!".format(guess))
else:
if guess == secret_num:
print("You got it buddy.My number was {}.".format(guess))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
guesses.append(guess)
else:
print("You ran out of choices.My number was {}.".format(secret_num))
play_again = input("Do you want to play again? Y/n :")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()
2 Answers
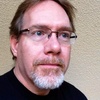
Chris Freeman
Treehouse Moderator 68,426 PointsIf the input can not be converted to an int
then the error is raised before guess
is assigned. One fix is to save guess input before converting:
try:
guess = input("Guess a number between 1 and 10: ")
guess = int(guess)
except ValueError:
print("Hey, {} is not a number huhh!!".format(guess))

shelley goel
3,547 PointsI would like to point out there is error in try-except block in Video . Because of combining int and input in one line, guess is not assigned if a ValueError occurs at first guess, thus the print statement in except will give UnboundLocalError.
I understand modifying Video is not easy and not useful for such small error. But it might help students if a note is made in the video description.
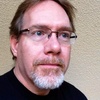
Chris Freeman
Treehouse Moderator 68,426 PointsYour are correct Shelley. The Number Game video @ 1:45
introduces a possible unbound error in the except
block.
Tagging Kenneth Love to for comment and possible Teacher's Notes update. (Actually, for the linked video, there are no teacher's notes.)
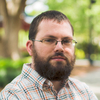
Kenneth Love
Treehouse Guest TeacherGood catch! The error message should probably just tell them they had a bad guess and not what the guess was. I'll leave a new note in the Teacher's Notes.
Anmol Gulati
13,991 PointsAnmol Gulati
13,991 PointsWorked. Thanks a lot.