Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial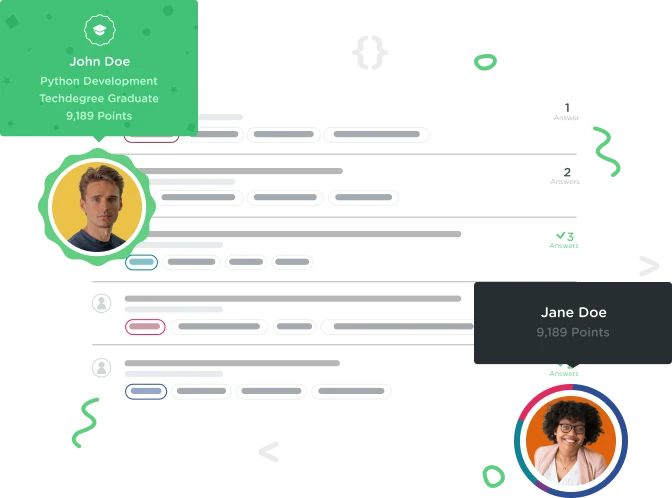
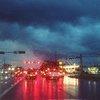
nathaniel marquez
Full Stack JavaScript Techdegree Student 2,145 Pointsnumber generator, jshint and youtube
I have taken a few days to consider what I am supposed to do in the
"RANDOM GENERATOR CHALLENGE" ... and I got something completely different.
Below is the code I wrote.
"use strict";
var valOne = window.prompt(); var valTwo = window.prompt();
window.document.write((Math.random(parseInt(valOne)) + (Math.random(parseInt(valTwo)))));
also when the two numbers are added together I not only get the number 1 from time to time but the number generated passes the number one.
I rewrote the code as follows.
"use strict";
var valOne = window.prompt("Pick a number from 1 to 6."); var valTwo = window.prompt("Just need one more number from 1 to 6");
var subVal = (parseInt(valOne) + parseInt(valTwo));
window.document.write(Math.floor(subVal) +1);
I get a random number but im not understanding how to limit the numbers between 1 - 6.
Also I tried to go for the gold in the challenge by adding the two numbers together to get the one generated number.
Thanks for any and all input cause im fixin to start drinking over this. :)
ps when I use the "use strict"; I get an error in JSHINT suggesting a function should be used when attempting "use strict"; rule.
1 Answer
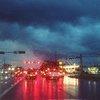
nathaniel marquez
Full Stack JavaScript Techdegree Student 2,145 PointsI AM LITERALLY ... LAUGHING OUT LOUD ...
I know just enough to really get turned around ... I was trying to google examples and even explanations for the maths. Thanks for the Example :)

Simon Coates
28,694 Pointsit's a little more intimidating on a single line, but if you break it down and look at the component parts, it makes more sense. But it's good practice for debugging, which tends to involve outputting (or using an inspection tool) to work through an algorithm bit by bit, confirming the values at each stage.
Simon Coates
28,694 PointsSimon Coates
28,694 PointsYou seem to have gotten confused enough to be attempting to solve a completely unrelated problem. In the first fragment, you're passing a parameter to the Math.random function. It doesn't expect one and doesn't know how to use in a passed in value. In the second fragment, you're flooring a value that you created by combining two int. Flooring an integer is redundant. The method to creating a random value in a range is to a) use low and high values to determine a range b) use Math.random to generate values over that range c) use the low value as a displacement. If struggling with the maths, break it (either your code, or the video's version) down into small steps and use console.log to watch what is happening with your values. eg.
a lot of people are perplexed with the maths. Personally, I find it helps to visualize the value on a graph - apparently my brain came with Microsoft excel (expressions of sympathy are assumed). And there is always the drink option.