Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial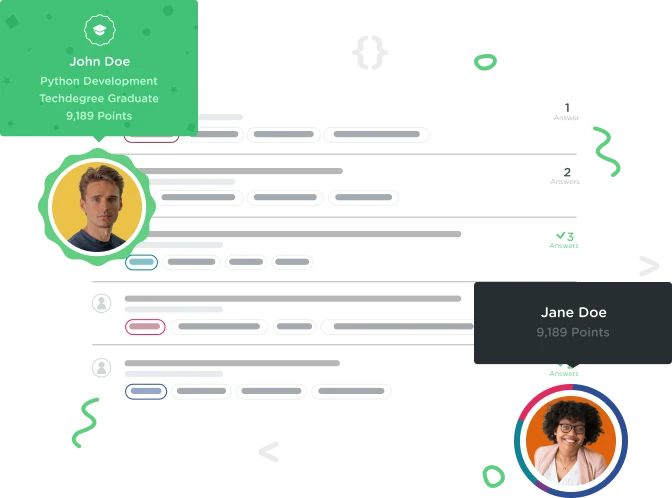

Hanifah W
395 Pointsnumbersgame.py trying to print statement if user inputs the same #? Help
Hi,
I am trying to extend the number game a bit to where if a user inputs a number twice it gives them a message. When I run it the script ignores this command.
import random
def game():
# generate a random number between 1 -100
secret_num=random.randint(1,100)
while True:
# catch someone if the submit a non intergers
try:
guess = int(input('Guess a number> '))
except ValueError:
print('{} That isn\'t a number'.format(guess))
# catch someone if they submit the same number
already_guesses = []
already_guesses.append(guess)
if guess == already_guesses:
print("You already guessed that number, try again")
# help with guesses and anounce if it is correct number!
else:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
number_guesses = len(guess) - already_guesses
print("It took you {} guesses".format(number_guesses))
break
elif guess == already_guesses:
print("You already guess that number, try again")
elif guess < secret_num:
print("You\'re getting hot, its a higher number")
else:
print("You\'re getting cold, try a lower number")
else:
print("You didn't guess it, my number was {}".format(secret_num))
play_again = input("Do you wanna play again? y/n")
if play_again != 'n':
game()
else:
print("Bah Bye!")
game()
[MOD: added ```python markdown formatting -cf]
8 Answers
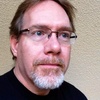
Chris Freeman
Treehouse Moderator 68,454 PointsThis issue is you are reinitializing the already_guesses
list to empty each loop. Also, when checking if a guess
is in already_guesses
use the in
keyword instead of the double-equals ==
:
# catch someone if they submit the same number
# already_guesses = [] # <-- move this statement outside and prior to the while-loop
already_guesses.append(guess)
if guess in already_guesses: # <-- changed double-equal to in
print("You already guessed that number, try again")

Hanifah W
395 PointsIt didn't quite work. It then started to give same input error for every thing I inputed. I'm still trying to get it to work, but now I am getting an indentation error on that nested else: within the second to last if statement. second to last Else:
else:
guess in already_guesses
print("You already guessed that number, try again")
-----------------
import random
def game():
# generate a random number between 1 -100 and initiate repeat guest list
secret_num=random.randint(1,100)
already_guesses = []
while True:
# catch someone if the submit a non intergers
try:
guess = int(input('Guess a number> '))
except ValueError:
print('{} That isn\'t a number'.format(guess))
# catch someone if they submit the same number
# help with guesses and anounce if it is correct number!
else:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
number_guesses = len(guess) - already_guesses
print("It took you {} guesses".format(number_guesses))
break
elif guess == already_guesses:
print("You already guess that number, try again")
elif guess < secret_num:
print("You\'re getting hot, its a higher number")
elif print("You\'re getting cold, try a lower number"):
else:
guess in already_guesses
print("You already guessed that number, try again")
else:
print("You didn't guess it, my number was {}".format(secret_num))
play_again = input("Do you wanna play again? y/n")
if play_again != 'n':
game()
else:
print("Bah Bye!")
game()
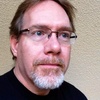
Chris Freeman
Treehouse Moderator 68,454 PointsI noticed you had mixed TABs and SPACEs. Try to stay will all SPACEs in your code.

Hanifah W
395 PointsThank you for your help by the way.

Hanifah W
395 Pointsok. I will reformat everything to try again.

Hanifah W
395 PointsI am trying to show patience. It kept giving me indentation errors until respaced everything. NowI am getting a syntax error on something that seems pretty straight forward.
Line 28 or "elif: print("You\'re getting cold, try a lower num"
The arrow is point to the colon next to the elif.
---------------------------
import random
def game():
# generate a random number between 1 -100 and initiate repeat guest list
secret_num=random.randint(1,100)
already_guesses = []
while True:
# catch someone if the submit a non integers
try:
guess = int(input('Guess a number> '))
except ValueError:
print('{} That isn\'t a number'.format(guess))
# help with guesses and anounce if it is correct number!
# catch someone if they submit the same number
else:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
number_guesses = len(guess) - already_guesses
print("It took you {} guesses".format(number_guesses))
break
elif guess == already_guesses:
print("You already guess that number, try again")
elif guess < secret_num:
print("You\'re getting hot, its a higher number")
elif:
print("You\'re getting cold, try a lower number")
else:
if guess in already_guesses:
print("You already guessed that number, try again")
else:
print("You didn't guess it, my number was {}".format(secret_num))
play_again = input("Do you wanna play again? y/n")
if play_again != 'n':
game()
else:
print("Bah Bye!")
game()
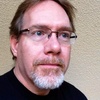
Chris Freeman
Treehouse Moderator 68,454 PointsWhen using elif
a condition must come before the colon, otherwise what is the "if" referring to?
Also, You can block format code in forum posts by surround the code with:
```python
```
with a blank line before and after the markdown.

Hanifah W
395 PointsOk, no more errors but I just cant seem to get it to fire off the command to catch repeated numbers. Here is the final code I have. Really appreciate this help.
import random
def game():
# generate a random number between 1 -100 and initiate repeat guest list
secret_num=random.randint(1,100)
already_guesses = []
while True:
# catch someone if the submit a non intergers
try:
guess = int(input('Guess a number> '))
except ValueError:
print('{} That isn\'t a number'.format(guess))
# help with guesses and anounce if it is correct number!
# catch someone if they submit the same number
else:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
number_guesses = len(guess) - already_guesses
print("It took you {} guesses".format(number_guesses))
break
elif guess == already_guesses:
print("You already guess that number, try again")
elif guess < secret_num:
print("You\'re getting hot, its a higher number")
elif guess > secret_num:
print("You\'re getting cold, try a lower number")
else:
if guess in already_guesses:
print("You already guessed that number, try again")
else:
print("You didn't guess it, my number was {}".format(secret_num))
play_again = input("Do you wanna play again? y/n")
if play_again != 'n':
game()
else:
print("Bah Bye!")
game()
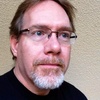
Chris Freeman
Treehouse Moderator 68,454 PointsIn an if/elif/else
statement, the first condition that matches will execute that code block an no other. It is likely one of the other branches is evaluating True
first. Move the if guess in already_guesses
earlier:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
number_guesses = len(guess) - already_guesses
print("It took you {} guesses".format(number_guesses))
break
# elif guess == already_guesses: # <-- redundant not needed
# print("You already guess that number, try again")
elif guess in already_guesses:
print("You already guessed that number, try again")
elif guess < secret_num:
print("You\'re getting hot, its a higher number")
elif guess > secret_num:
print("You\'re getting cold, try a lower number")
Also, I don't see a statement where you actually append the guess to already_guesses
. Where did this line go?
already_guesses.append(guess)

Hanifah W
395 PointsI'm not sure if you are still up, but ... No more errors . this script is not however doing two things.
Once the user guesses the number it should account for how many attempts a user made to guess the number - how many time he/she repeated a number.
It should give the message "YOu already guessed that number, try again when they input the same number twice or more than once.
It late here but I really need to figure this out withint eh next hour. I'm determined. I hope you can help.
import random
def game():
# generate a random number between 1 -100 and initiate repeat guest list
secret_num=random.randint(1,10)
already_guesses = []
number_guesses = 0
final_count = number_guesses - len(already_guesses)
while True:
# catch someone if the submit a non intergers
try:
guess = int(input('Guess a number> '))
except ValueError:
print('{} That isn\'t a number'.format(guess))
number_guesses += 1
# help with guesses and announce if it is correct number!
# catch someone if they submit the same number
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
print("It took you {} guesses".format(final_count))
break
elif guess < secret_num:
print("You\'re getting hot, Try a higher number")
elif guess > secret_num:
print("You\'re getting cold, try a lower number")
elif guess in already_guesses:
print("You already guessed that number, try again")
else:
already_guesses.append(guess)
else:
play_again = input("Do you wanna play again? y/n")
if play_again != 'n':
game()
else:
print("Bah Bye!")
game()
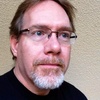
Chris Freeman
Treehouse Moderator 68,454 PointsReview your flow! Notice that if a non-number is entered, the exception block is executed, then the code continues, first by incrementing number_guesses
. Perhaps think about adding a small loop around the whole try
statement to wait until a valid input is seen.
Also notice that since guess
is either == or < or > the secret number the last elif
and else
will never execute:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
print("It took you {} guesses".format(final_count))
break
elif guess < secret_num:
print("You\'re getting hot, Try a higher number")
elif guess > secret_num:
print("You\'re getting cold, try a lower number")
elif guess in already_guesses: # <-- will never reach here
print("You already guessed that number, try again")
else: # <-- will never reach here
already_guesses.append(guess)
It's late for me as well. I'm off for the night. Good luck. Post back on your progress.
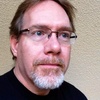
Chris Freeman
Treehouse Moderator 68,454 PointsReview your flow! Notice that if a non-number is entered, the exception block is executed, then the code continues, first by incrementing number_guesses
. Perhaps think about adding a small loop around the whole try
statement to wait until a valid input is seen.
Also notice that since guess
is either == or < or > the secret number the last elif
and else
will never execute:
if guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
print("It took you {} guesses".format(final_count))
break
elif guess < secret_num:
print("You\'re getting hot, Try a higher number")
elif guess > secret_num:
print("You\'re getting cold, try a lower number")
elif guess in already_guesses: # <-- will never reach here
print("You already guessed that number, try again")
else: # <-- will never reach here
already_guesses.append(guess)
It's late for me as well. I'm off for the night. Good luck. Post back on your progress.

Hanifah W
395 Pointsso I've been working on this and decided to simplify a bit but am still feeling the pain regarding from control flow issues, indentation and now an elif syntax error. I"m trying ot understand how they all can affect one another. But what is most important to me is locking down this control flow issue. So here is where I am as of date. I am on several forums at this point, reading documentation and even emailing my wife's dad for help. Anyway, I appreciate any further insight that can be given.
A review:
I am trying to create a program that executes the following:
secret_num == generate a random # between 1-10
ask user to guess this number between 1-10
if guess == secret_num congratulate them and provide the number of guesses number_guesses it took (minus any duplicate guesses)
account for duplicate guesses 5. let them know if guess is too high or low
I am having typical new programmer problems with syntax, indentation and control flow. Right now I'm getting a syntax on the elif guess == secret num.
Otherwise I cannot get this code to account for duplicates to save my life. I would appreciate any help. Thank you .
import random
def game():
# generate a random number between 1 -100 and initiate repeat guess list
secret_num=random.randint(1,10)
# initiate duplicate list and number of guesses
already_guesses = []
number_guesses = 0
while True:
# ask for user guess
guess = int(input('Guess a number from 1-10> '))
# help with guesses and announce if it is correct number!
# catch someone if they submit the same number
if guess in already_guesses:
print ("You already guessed that number, try again")
already_guesses.append(guess)
number_guesses += 1
elif guess == secret_num:
print("whooohoo! you guessed it, my number is {}".format(secret_num))
print("It took you {} guesses".format(number_guesses - len([already_guesses])))
break
elif guess < secret_num:
print("You\'re getting hot, try a higher number")
else:
guess > secret_num
print("You\'re cold, try a lower number")
# ask if they want to play again
else:
play_again = input("Do you wanna play again? y/n")
if play_again != 'n':
game()
else:
print("Bah Bye!")
game()
This is the latest syntax error I'm encountering:
/Library/Frameworks/Python.framework/Versions/3.5/bin/python3.5 /Users/hanifahwalidah/PycharmProjects/hackbright/.idea/hacknumbers
File "/Users/hanifahwalidah/PycharmProjects/hackbright/.idea/hacknumbers", line 18
elif guess == secret_num:
^
SyntaxError: invalid syntax
Process finished with exit code 1
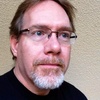
Chris Freeman
Treehouse Moderator 68,454 PointsThe line above the error line is indented to the same level as line 18. This starts a new statement. elif
cannot begin a statement.
if guess in already_guesses:
print ("You already guessed that number, try again")
already_guesses.append(guess) # <-- this indent closes the 'if' statement
number_guesses += 1
elif guess == secret_num: # <-- 'elif' cannot start a new statement
Styling tip: indent your comments to match the code at that point.