Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial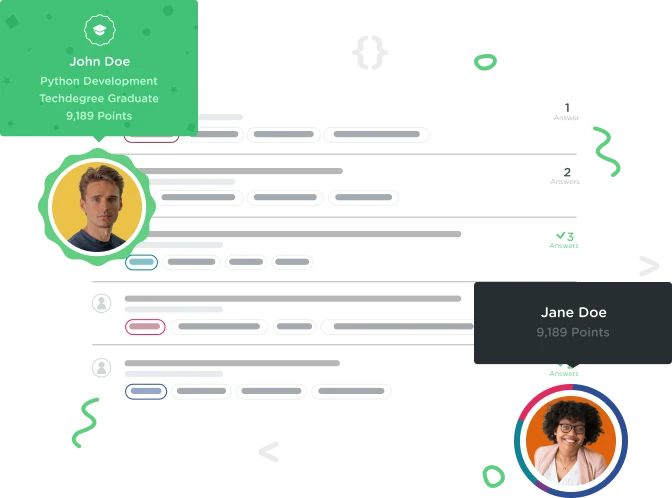

traolach trewhella
Courses Plus Student 835 Pointsokay for this 2 /part i dont understand the list of colors what is a list of colors and how do i remove it please help
i tried copy the colors it didint work how do i remove colors and agian what are colors?
states = [
'ACTIVE',
['red', 'green', 'blue'],
'CANCELLED',
'FINISHED',
5,
]
states.remove(5)
2 Answers
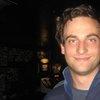
Alex Sell
19,355 PointsHi Traolach,
So a list in python is basically just a collection or grouping of items or elements. For example [1, 2, 3] is a list of numbers. ["dog", "cat", "mule"] is a list of animals. ["dog", 2, "s"] is a list of miscellaneous items. You can even have empty lists: []. In other programming languages, lists are often referred to as arrays.
To answer your question, ['red', 'green', 'blue'] would be the list of colors you are looking for. In fact, you can see that ['red', 'green', 'blue'] is a list that is inside another list called states.
When you call the remove method on states, you have to pass in the object you wish to remove from the list. So if I pass in the string 'ACTIVE', I am removing 'ACTIVE' from the states list. e.g. states.remove('ACTIVE')
If I want to remove the list ['red', 'green', 'blue '] from the states list, I would have to do something very similar.
Hope this helps!
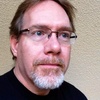
Chris Freeman
Treehouse Moderator 68,441 PointsYou can remove the list
in two ways:
states = [
'ACTIVE',
['red', 'green', 'blue'],
'CANCELLED',
'FINISHED',
5,
]
states.remove(5)
# remove using explicit list
states.remove(['red', 'green', 'blue'])
# OR using the index of the list within 'states'. The list is at index 1
states.remove(states[1])
# but this is the same as
states.pop(1)
# or as
del states[1]
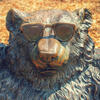
Ge Ode
Full Stack JavaScript Techdegree Student 9,002 PointsHey Chris, follow up question for you -
I gather that you can only remove 1 value at a time using the list.remove method, but why? I imagine that if we could input multiple values in a list.remove method, the list would have to be reindexed for each value removed. But is there a reason why loops (or methods i don't know) do this instead?
Thanks!
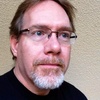
Chris Freeman
Treehouse Moderator 68,441 PointsThere is an inherent danger of looping over a list while also adding or removing elements due to the reindex (or mis-indexing) that occurs. Working around this usually means making a copy of the list to loop over but still make changes to the original list. I'm unaware of a builtin construct that would notice that an element of the iterable had been deleted and would then automatically skip to the correct next element.
Sometimes the limitations of a method, like remove()
, have to do with the resultant behavior instead of the input limitation.
For list.remove()
it raises an error if the item is not found:
list.remove(x)
Remove the first item from the list whose value is x. It is an error if there is no such item.
So if more than one removal item was provided as an argument, how should the method behave if first argument was present and removed but the second element wasn't?
What of the first argument was not present but the second argument was. Does the get error get raised before the second argument is removed?
Basically it best to keep the methods simple then wrap them in loops or list comprehensions to get the extended functionality.
If the removal is extensive then maybe conversion to sets may be called for so the set.difference()
method could be used.
Another consideration is whether you want to keep the original list intact and produce a new sublist or whether the original list needs to changed.