Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial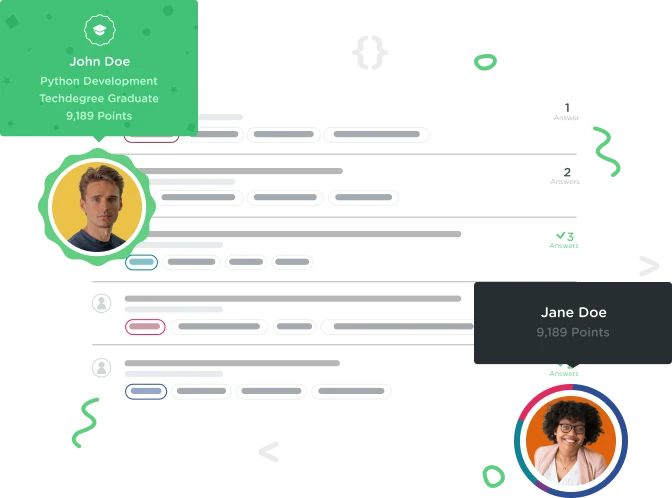

Henrique Costardi
4,883 PointsOn stage 4 out of 5 Im getting the correct results in my terminal but wont accept in the test. any ideas?
The dictionary will look something like:
{'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
Each key will be a Teacher and the value will be a list of courses.
Your code goes below here.
def num_teachers(arg): count = 0 for key in arg.keys(): count += 1 return count
def num_courses(arg): count = 0 courses = [] for value in arg.values(): courses.extend(value) for course in courses: count += 1 return count
def courses(arg): courses1 = [] for value in arg.values(): courses1.extend(value) return courses1
def most_courses(arg): for key in arg.keys(): temp_list = [] temp_list.extend(arg[key]) arg[key] = len(temp_list) max_count = max(arg.values()) for name, total in arg.items(): if total == max_count: top_teacher = name else: pass return top_teacher
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(arg):
count = 0
for key in arg.keys():
count +=1
return count
def num_courses(arg):
count = 0
courses = []
for value in arg.values():
courses.extend(value)
for course in courses:
count += 1
return count
def courses(arg):
courses1 = []
for value in arg.values():
courses1.extend(value)
return courses1
def most_courses(arg):
for key in arg.keys():
temp_list = []
temp_list.extend(arg[key])
arg[key] = len(temp_list)
max_count = max(arg.values())
for name, total in arg.items():
if total == max_count:
top_teacher = name
else:
pass
return top_teacher
1 Answer
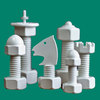
Steven Parker
231,007 PointsBe careful about assuming you are "getting the correct results in my terminal".
There are two things that make this determination difficult:
- If you have incorrectly interpreted the challenge instructions in your coding, you will quite likely be applying the same incorrect interpretation to the results.
- The challenge will apply multiple test sets to evaluate your function. Your own test set(s) may not be extensive enough to reveal possible issues in the code.
Now, regarding your code, here's a few hints:
- be sure to perform initializations before a loop
- you only need one loop for this function
- remember that each item in the dictionary will have a string and a list of classes
- you can't compare a collection (like a list) directly to a scalar value (like int)
- you can omit else entirely if all it does is pass