Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial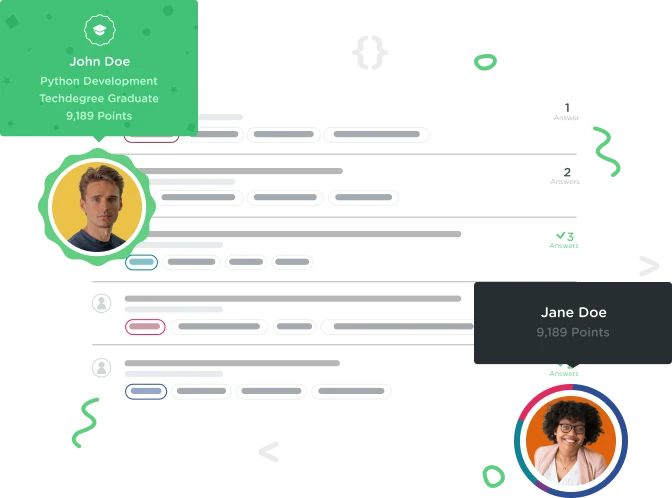
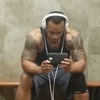
Isaac Barron
7,011 PointsOn task 3 of the final part of Python basics I can't figure out what I'm doing wrong. I know its something simple.
Challenge Task 3 of 3
Alright, last step but it's a big one. Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1. I know it's a lot, but I know you can do it!
ERROR => Bummer! Try again!
Error message isn't giving much to go off of and I copied and pasted this some code somewhere else and it worked fine
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
num = random.randint(1, 99)
if even_odd(num):
print('{} is even').format(num)
else:
print('{} is odd').format(num)
start -=1
3 Answers
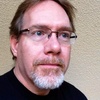
Chris Freeman
Treehouse Moderator 68,461 PointsThe error is in the syntax. format()
is a string method and must be used with a string. You have parens between the string and the method.
if even_odd(num):
print('{} is even'.format(num))
else:
print('{} is odd'.format(num))
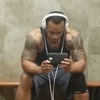
Isaac Barron
7,011 PointsO ok thanks. Very odd that it worked as expected elsewhere.
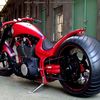
remslysambo
8,216 PointsThank you for your Q&A
Mohamed THIAM
1,091 PointsMohamed THIAM
1,091 PointsChris, I'm having a bit of difficulties with this one.
I can't seem to understand the logic behind the code.. I'm a bit confused by the meaning of these lines:
return not num % 2
and this section:
Could you translate them for me in 'English'?
Thank you and happy new year!
Mo.
Chris Freeman
Treehouse Moderator 68,461 PointsChris Freeman
Treehouse Moderator 68,461 PointsThere is a little bit of wizardry going on in
return not num % 2
num % 2
is modulo math that divides a number until there is only a remainder. using% 2
results in a0
for even numbers and a1
for odd numbers.If looking at the "truthiness" of this result, an even number would get
0
or False, and an odd number would get1
or True. This is the opposite of what we want.Using a
not
reverses the results sonot False
(for even numbers) becomesTrue
andnot True
(for odd numbers) becomesFalse
.Therefore,
return not num % 2
returnsTrue
for even numbers andFalse
for odd numbersIn the next section of code, the
while
loop is using the "truthiness" ofstart
to decide to loop. As long asstart
isn't0
("falsey") then the loop continues.Note that the helper function
even_odd()
would be better named asis_even()
so that theif
statement makes more sense as:One warning on using simple "truthiness" on a variable. If
start
got decremented by 2 (due to a bug) the value0
would be missed whenstart
jumped from1
to-1
. Negative numbers are "True" and thewhile
loop would run forever.Mohamed THIAM
1,091 PointsMohamed THIAM
1,091 PointsThanks for the explanation Chris!
Scott Halford
8,964 PointsScott Halford
8,964 PointsI am getting an error that states my "code took too long to run."
Chris Freeman
Treehouse Moderator 68,461 PointsChris Freeman
Treehouse Moderator 68,461 PointsScott, your indentation is off. The last five line need indented on stop:
Without the indent changes the only statement in the
while
loop. is thenum
assignment.start
never changes and the loop runs forever (or until the cpu cap is hit)Scott Halford
8,964 PointsScott Halford
8,964 PointsOops. Thanks Chris.