Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial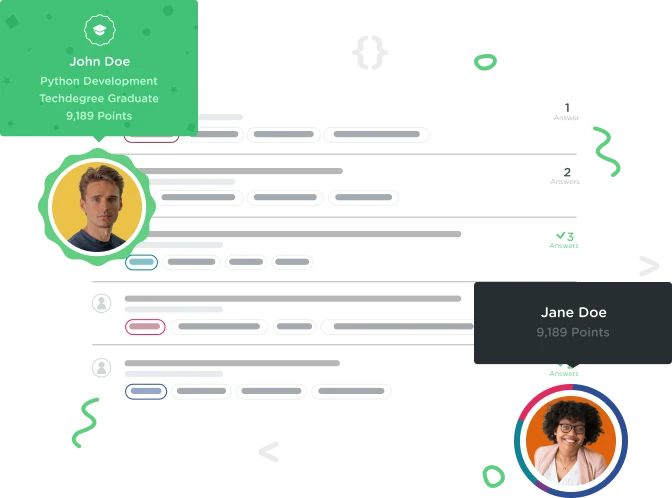

Jian Chen
4,938 PointsOnce a variable is declared to be "final", why can we still change it to something else in this situation?
since factLabel is final how its that factLabel.setText(fact); can still change it?
public class FunFactsActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare View variables and assign them the Views from layout file.
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = "Ostriches can run faster than horses.";
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
PLEASE HELP ME clarify this. I would appreciate any help.
2 Answers
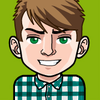
Harry James
14,780 PointsHey Jian!
We're never actually changing the value of the variable itself, it still is always referring to the layout element at R.id.factTextView
.
When we use setText(fact)
, we're calling this off the element itself. So, the thing to note here is that we're still calling this from R.id.factTextView
and that this element has a special methods to change its properties, one of these methods being the setText()
method, which changes the text property.
So, when we declare a variable as final
, we can never change what that variable refers to after it has been set. What we're doing in this example is we're running a method off this variable. We can change properties of our XML element but we can never change the element we're referring to.
Hopefully this should make sense to you now but, if there's still something that's not quite clear, let me know know and I'll try my best to explain it for you :)

Adrian Diaz
2,469 PointsI was really confused during the video but this explanation definitely helps. I'm still a little confused how changing an element's properties doesn't count as changing the element itself. What would be considered a real change? Changing the property name that we're referring to?
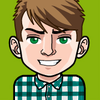
Harry James
14,780 PointsYes, basically, we're checking to see if the bit after the =
is any different.
When we use an XML element, it is actually in an int
format and you can see this in your R.java class (Do not modify this file!) which is found in app >> build >> generated >> source >> r >> debug >> com.yourpackage.name >> R.java.
If we change the pattern on the side, we're changing what the variable is equal to:
final EditText editText1 = (EditText) findViewById(R.id.editText1);
// The bit after the equals will be something like 0x01010126
final EditText editText2 = (EditText) findViewById(R.id.editText1);
// We're referring to something different here, a new instance, so it could be something like 0x01010127
Now, as long as we don't change the object we're referring to, we are fine to use the final
keyword.
It may seem a bit weird to think about it in this way as, you don't see any changes in your Java code when you reference a change on an XML element - there's no confirmation that it has changed anywhere.
A good way to think of this is with a TextView
- we can reference the object once, make it final
, and we will always be referring to that object. We can then change the text in the TextView but, note that this changes in the XML language - nothing ever changes in Java and so, this change is basically just passed on to XML where all the work happens. XML doesn't send anything back to Java saying "Hey! We've updated it, now you should change your reference", the reference will always remain the same.
Hopefully this should clarify it for you but, if there's anything else, give me a shout :)