Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial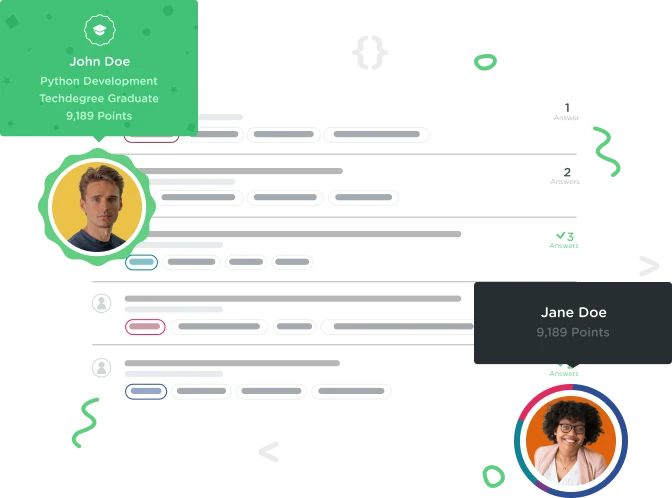
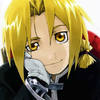
Gabriel D. Celery
13,810 PointsOne Solution for the extended Student Record Search Challange
Here is a possible solution to Dave's challenge. The way it works is that I created an empty array called "matchingSearchResults".
Then if the program finds a match it pushes the properties and values of the matching object into the array.
After this it checks whether the array is empty. If yes you get an alert, if not it prints out the data you requested.
Here is the student_report.js file
// Declaring the functions
var message = '';
var matchingSearchResults = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// Starting the search loop
while (true) {
var search = prompt("Type in the student's name you are looking for. If you want to stop, type in QUIT!")
if (search === null || search.toUpperCase() === "QUIT") {
break;
}
// If we find a matching name the matching object's properties and values are pushed into the matchingSearchResults array
for (var i = 0; i < students.length; i ++) {
if (search === students[i].name) {
matchingSearchResults.push(students[i]);
}
}
// If the matchingSearchResults array is empty we get an alert
if (matchingSearchResults.length < 1){
alert("We don't have any student named like that!")
}
// Otherwise we print out the array's content
for (var j = 0; j < matchingSearchResults.length; j ++) {
message += '<h2>Student: ' + matchingSearchResults[j].name + '</h2>';
message += '<p>Track: ' + matchingSearchResults[j].track + '</p>';
message += '<p>Points: ' + matchingSearchResults[j].points + '</p>';
message += '<p>Points: ' + matchingSearchResults[j].achievements + '</p>';
print(message);
}
// Resetting the array otherwise every time we would type a new name into the box it would print out the entire content of the array rather than working properly
matchingSearchResults = [];
}
Alex Flores
7,864 PointsAlex Flores
7,864 PointsReally liked your code. Simple and easy to read.