Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial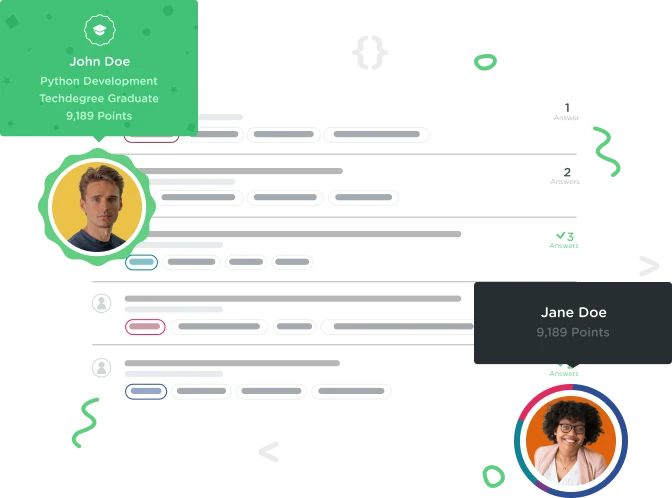

Mark Ryan Holanda
4,223 PointsOOP Code Challenge: Oops! It looks like Task 1 is no longer passing.
Hi im a bit lost with this code challenge.. here's the instruction:
Challenge task 3 of 3
Return an collection array of house objects whose public properties roof_color OR wall_color match the passed color parameter. Note: The local houses array contains an array of House objects, each of which has a public property for "roof_color" and "wall_color".
<?php
// add code below this comment
class Subdivision {
public $houses = array();
public function filterHouseColor($color)
{
$houses = [];
foreach ($this->houses as $house) {
if ($house[roof_color] == $color) || ($house[wall_color] == $color) {
$houses[] = $house;
}
} return $houses;
}
}
?>
the error is: Oops! It looks like Task 1 is no longer passing.
But the Task 1 is correct.. so not sure whats wrong with my code.. please help! thanks!
<?php
// add code below this comment
class Subdivision {
public $houses = array();
public function filterHouseColor($color)
{
$houses = [];
foreach ($this->houses as $house) {
if ($house[roof_color] == $color) || ($house[wall_color] == $color) {
$houses[] = $house;
}
} return $houses;
}
}
?>
2 Answers
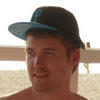
Algirdas Lalys
9,389 PointsHi Mark Ryan Holanda,
Well your foreach loop is good. but in your if statement you are checking $house as an array, but it's and object (it specifies in 1 Task "Add a new class named "Subdivision" with a public $houses property which will be a collection array of House OBJECTS."), so you should check objects property roof_color and wall_color. And you should create another array to store those checked $house objects, then you will be able to return them:). So it should be something like this.
<?php
// add code below this comment
class Subdivision
{
public $houses = array();
public function filterHouseColor($color)
{
// temporary array to store checked houses
$checkedHouseArray = array();
foreach($this->houses as $house) {
// check every house object propertys
if($house->roof_color == $color || $house->wall_color == $color) {
// roof_color OR wall_color property matches our $color argument, then we add it to our temporary array
$checkedHouseArray[] = $house;
}
}
// return array all checked houses
return $checkedHouseArray;
}
}
?>

Mark Ryan Holanda
4,223 PointsHi Algirdas Lalys,
Thank you so much for your response it works now! I was a little bit confused on calling the properties.. thanks for the clarification! Have a great day!