Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial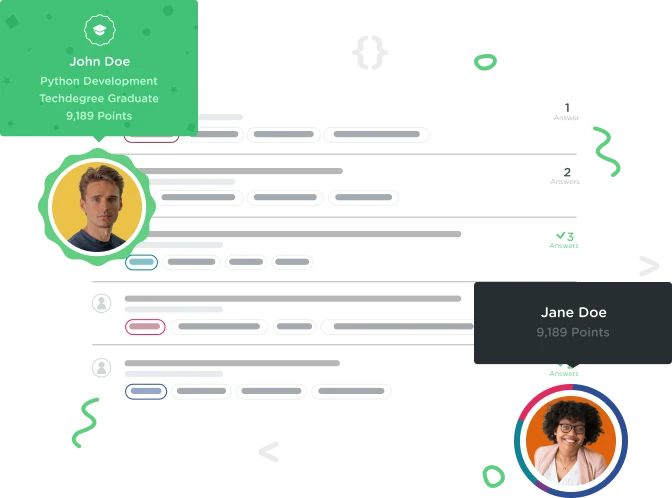
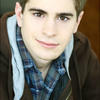
Dustin Kahn
4,194 PointsPart 1 Of Extra Challenge (JavaScript Loops, Arrays, Objects): What's wrong with my code?
I've looked over this code many times and I can't seem to figure out why when I add my final "else if" statement that my code no longer recognizes a correct student name input. Also, I'm new to Treehouse Forums. Could some instruct me on how to add screenshots of workspaces into a message? Thank you in advance for your help!
var message = ' '; var student; var search;
function print(message) { var outputDiv = document.getElementById('output'); outputDiv.innerHTML = message; }
function getStudentReport (student) { var report = '<h2>Student: ' + student.name + '</h2>'; report += '<p>Track: ' + student.track + '</p>'; report += '<p>Points: ' + student.points + '</p>'; report += '<p>Achievements: ' + student.achievements + '</p>'; return report; };
while (true) {
search = prompt ("Search student records: type a name [Jody] (or type 'quit' to end)");
if (search === null || search.toLowerCase() === "quit") {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message = getStudentReport (student);
print (message);
} else if (student.name.toLowerCase() !== search.toLowerCase() ) {
message = "<h2>No student name was found</h2>";
print (message);
}
}
};
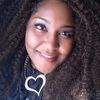
LaToya Legemah
12,600 PointsMay I ask for your HTML as well? I would like to run your code. Thanks
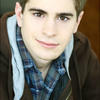
Dustin Kahn
4,194 Pointsvar students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
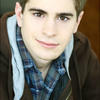
Dustin Kahn
4,194 Points<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Students</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>Students</h1>
<div id="output">
</div>
<script src="js/students.js"></script>
<script src="js/student_report.js"></script>
</body>
</html>
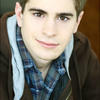
Dustin Kahn
4,194 PointsHere you go! Thank you so much!!!
2 Answers
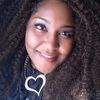
LaToya Legemah
12,600 PointsOk, I got it to work. It looks like you need a break in the if statement in the for loop
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message = getStudentReport (student);
print (message);
break;
} else if (student.name.toLowerCase() !== search.toLowerCase() ) {
message = "<h2>No student name was found</h2>";
print (message);
}
}
};
Your code goes through each element in the array. When you put in Dave you always got his information but not Jody's. It seemed to me that the code always hit the "else if" never returning the first print(message).
If anyone else cares to clarify please do :) Also I used https://jsfiddle.net/ to help run the code and alerts to help figure out what part of the code is running. That may help in the future. :)
Your entire code is below.
var message = '';
var student;
var search;
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
};
function getStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
};
while (true) {
search = prompt ("Search student records: type a name [Jody] (or type 'quit' to end)");
if (search === null || search.toLowerCase() === "quit") {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message = getStudentReport (student);
print (message);
break;
} else if (student.name.toLowerCase() !== search.toLowerCase() ) {
message = "<h2>No student name was found</h2>";
print (message);
}
}
};
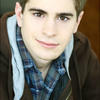
Dustin Kahn
4,194 PointsThank you! I'm still a bit confused as to why a "break" is needed. The loop shouldn't required it based on the parameters...or am I mistaken?
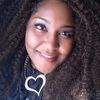
LaToya Legemah
12,600 PointsThere is an icon on the top right of the workspace. It looks like a box with a dot in the middle. Click that to take a snapshot of your workspace, then share the link.
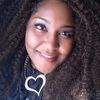
LaToya Legemah
12,600 PointsI am breaking because when the program finds what it needs, it needs to stop and display the information. So the code was reading:
WHILE TRUE
IF the search is not empty and the user does not in QUIT do the following:
FOR (LOOP )
IF the student name is equal to the student name
show the student information
ELSE IF the student name is not equal to the student name
show the no student name was found message
In your original code it just kept looping. The new code reads
WHILE TRUE
IF the search is not empty and the user does not in QUIT do the following:
FOR (LOOP )
IF the student name is equal to the student name
show the student information
BREAK go back to the top of the while loop
ELSE IF the student name is not equal to the student name
show the no student name was found message
After looking at the code, I realize it can be better. I changed the way the code is written to make it a bit more efficient. I took out the else since it's no longer needed.
while (true) {
search = prompt ("Search student records: type a name [Jody] (or type 'quit' to end)");
if (search === null || search.toLowerCase() === "quit" ) {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message = getStudentReport (student);
print (message);
break;
}
if (student.name.toLowerCase() !== search.toLowerCase() ) {
message = "<h2>No student name was found</h2>";
print (message);
}
}
};
Let me know if you have any more questions :)
Dustin Kahn
4,194 PointsDustin Kahn
4,194 Points