Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial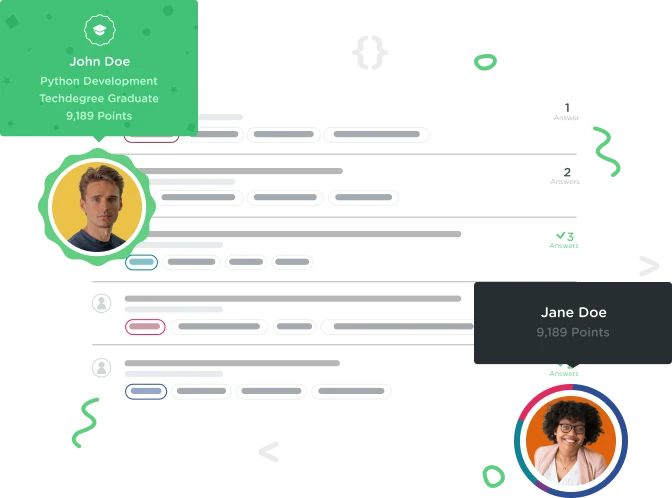
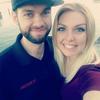
Unsubscribed User
4,131 PointsPassing a variable from the View to the Controller without using a form or link_to??
I'm trying to pass data from an API call I made in my View to the Controller to then save the data to the Database. This is my first Rails app so I'm a bit confused on how I can get these variables I've created from my API call data into my database. Thanks!
3 Answers
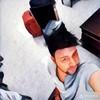
Ari Misha
19,323 PointsHiya Bryan! Whenever you create an instance variable using @ in your controller, its always available for you to use in views. But this is an API application ,right? I dont think you need views for it, right? Thats why its an API application. You could just respond with data directly with json from your controller. Here is an example:
# app/controller/api/v1/users_controller.rb
....
def index
users = User.sort('created_at DESC')
render json: users
end
....
The above example will generate a get request for the users#index and will return a json formatted data. You can try this out from your command line or terminal using cURL or you could use postman application or its chrome extension.
There are couple of gems out there that i think you should involve in your project. First one is jbuilder. Its really kickass for generating all kinds of json from hashes, arrays or even strings. Second gem you should install in your project is active_model_serializers. This sit on top of your API layer and converting 'em attributes into json behind the scenes. (btw jbuilder solves all your issues with views and similar stuff)
There are additional stuff i think you should implement in your API project. First one, HTTP authentication. You wouldnt want anyone to access your API layer right? Rather than implementing a conventional Auth , you must add HTTP auth which works around the concept auth_tokens. Second one is Rack-Cors. It handles all your cross-origin AJAX requests and take care of all kinds of requests whether is coming from Nodejs apps or Spring or PHP or even React. The last but not least gem is rack-attack. It provides additional security features like DDoS attacks, brute force, hammering, or even to monetize with paid usage limits.
This might seem like a lot but it takes your application to real world architecture and its a great practice or a project to show off around. Good Luck!
~ Ari
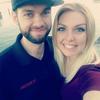
Unsubscribed User
4,131 PointsNo problem lol yes that is what I'm doing. I want to save 2 variables of data that I'm getting my from HTTParty call and save them to the database. I'm having a hard time finding out how online because I'm not using a form or link_to button to save them. Here's my code
<% headers = {
"Authorization" => "Basic "+Base64.strict_encode64(@apikey+":"+reduser+":"+redpass),
"Content-Type" => "application/json"
} %>
<% response = HTTParty.get(
"http://dev.api2.redtailtechnology.com/crm/v1/rest/authentication",
:headers => headers)%>
<% @userkey = response['UserKey'] %>
<% @redtailid = response['UserID'] %>
<% if response['Message'] == 'Success' %>
<% @userkey # I want to save these 2 variables to the %>
<% @redtailid # database once this call is made %>
<% end %>
<%= response['Message'] %>
<%end%>
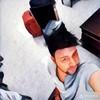
Ari Misha
19,323 PointsBryan Reed I think you nailed it. And you implemented everything. What was your question again? (:
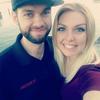
Unsubscribed User
4,131 PointsThe @userkey and @redtailid shown aren't saving to the database from the view here.
Here's my controller code:
def show
@apikey = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxx'
@userkey = User.find(set_user).userkey
@redtailid = User.find(set_user).redtailid
end
I'm missing whatever I need to actually tell the controller to save these variables to my database from my view.. :/
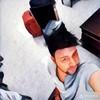
Ari Misha
19,323 PointsInteresting! I can think of couple of methods to implement this, at this moment. First one would be like saving the data before it hits your main action in the controller with using before_filter and .create() method. Use this one only when you're sure of the data's state, i mean it needs to be kinda static i.e, it wont keep changing and its sanitized, like images, right?
The second one would be something like below:
json_response = JSON.parse(your_response_object)
ApiCall.create(:artist_name => json_response["user"]["email"])
Does that make sense?
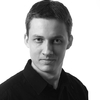
Maciej Czuchnowski
36,441 PointsIs there any reason to make that call from the view? This kind of logic should be moved to Ruby classes - controller or a service object.
Unsubscribed User
4,131 PointsUnsubscribed User
4,131 PointsHi Ari, this is a good response but it's not really what I was asking. I'm making an external API call to another app in my view to obtain data using HTTParty. I need to input that data into my database after the API call. I'm not making an API application.
Ari Misha
19,323 PointsAri Misha
19,323 PointsBryan Reed My bad Sorry haha. So you want your application to make an API call, right? Am i getting it this time, right?