Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial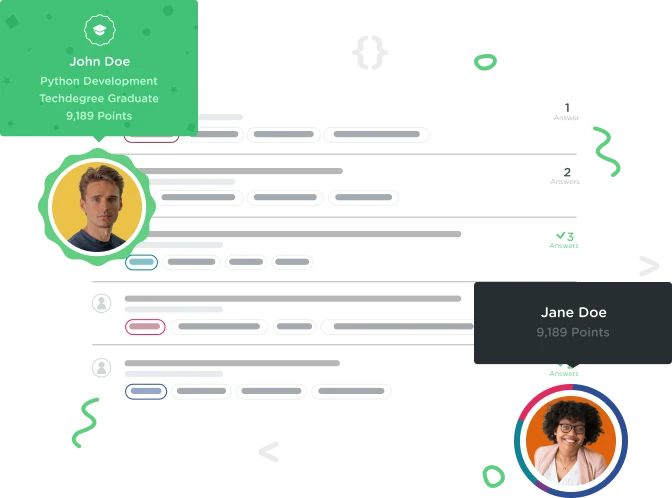

Andy Christie
1,361 PointsPlease can you help me with this python challenge
Write a function named members that takes two arguments, a dictionary and a list of keys. Return a count of how many of the items in the list are also keys in the dictionary.
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
def members():
my_list = ["app", "ora", "cab"]
my_dict = ("app": 1, "ora": 2, "cab": 3)
count = len(my_list[:2] == my_dict[::2]())
return count
5 Answers

jayj
2,515 PointsThere are a couple of things wrong with your code right now. Let's disect.
def members():
The first problem is that your function has no arguments. A function needs data to work your code on. In this case, we want to add two arguments to your parenthesis. Example: def sum_of_numbers(number1, number2):
my_list = ["app", "ora", "cab"]
my_dict = ("app": 1, "ora": 2, "cab": 3)
This is the data which you want to pass as arguments to your function. If they are in the function already, then your function can not call them! Make sure these are before you define your function.
Now, to actually solve the problem, my method was using a loop. My loop was to find each dictionary key
for a in my_dict:
And once I found a key, I checked if that key was in my list.
if a in my_list:
If the key is in my list, I then add +1 to a count variable, which I should have made at the beginning of my function.
Finally, return that count at the end. (Make sure you pay attention to white space and you return count outside of both loops.)
Good luck!

Jorge Dominguez
12,632 PointsRemember you need to pass information to your function.
def members(myDict, myKeys):
return what_ever_you_need
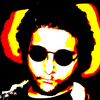
joewode
13,803 PointsThere is no room in your function to accept the arguments that the code challenge is trying to pass it. To pass arguments into a function you must include them in between the parentheses as you define the function. In this case you need to include room to accept the my_list and my_dict arguments, like so:
def members(my_list, my_dict):
This lets your function know that it is going to be passed some data. Think of these arguments like generic containers that will hold whatever data is passed to them. When you check your work with the code challenge it will try to pass data to your function through those arguments. If those arguments aren't there, it can't pass the data, and you fail right away.
After that you need to use a few if statements to compare the data in the two arguments your function is receiving. I hope this is specific enough to be of some help.
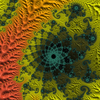
Wes Chumley
6,385 PointsWOW! This one took me a long while to decipher. Here's my solution(I'm sure there are many other ways):
def members(my_dict, my_list):
count = 0
for item in my_list:
try:
my_dict[item]
except:
count = count-1
count = count+1
print(count)
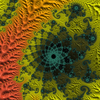
Wes Chumley
6,385 PointsThis should also work and seems like maybe more how it 'should' be written.
def members(my_dict, my_list):
count = 0
for item in my_list:
try:
my_dict[item]
count += 1
except:
count -= 0
print(count)

SCU ACM
32,149 PointsHere is what I came up with. Hopefully, it is the most efficient logic :)
def members( my_dict, my_list) : count = 0 for item in my_list: if item in my_dict.keys(): count +=1 return count
Andy Christie
1,361 PointsAndy Christie
1,361 PointsHi thankyou, I tried that but I did it with slicing would that not work or am I doing it wrong, I did my_list = ["app", "ora", "cab"] my_dict = {"app": 1, "ora": 2, "bug": 3}
def members(my_dict, my_list): count = () for key in (my_dict, my_list): if my_dict[:] == my_list[:]: count +1 return count
jayj
2,515 Pointsjayj
2,515 PointsUnfortunately you can not slice a dictionary because it is not in a permanant sequence. You never know what position each value will be in when you call it. This is why you use key's to find values.
So if I have a dictionary with:
I have no idea if its going to give me the name first, or the gender. Neither does Python! If we sliced, it could give back anything.