Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial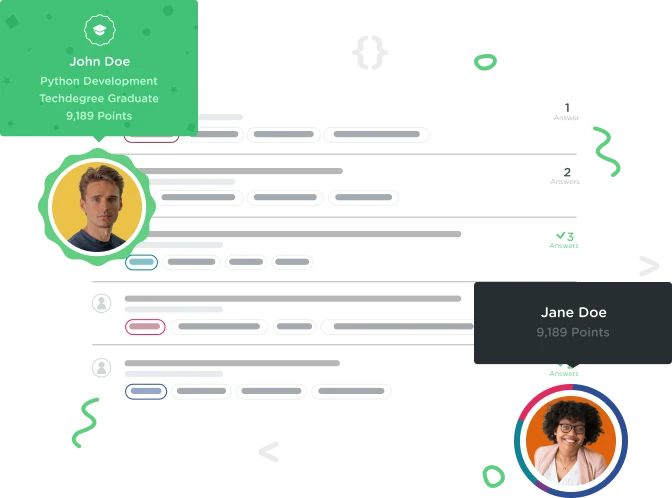

Jessica Orellanes
11,245 PointsPlease, check my code
var topNumber = parseInt(prompt("Tell me a number")); var bottomNumber = parseInt(prompt("Tell me another, but smaller")); var randomNumber = Math.floor(Math.random()*topNumber) + bottomNumber; document.write(randomNumber + " is a random number between " + bottomNumber +" and " + topNumber);
What do you think? It works? I'm bad at math. I don't get why he subtracts the bottomNumber.
3 Answers

Jacob Moyle
6,150 PointsHello Jessica,
Let me take a crack at breaking this code down for you while answering your questions.
"What do you think?"
- I recommend you look over markdown syntax. It'll really boost your ability to communicate your thoughts when it comes to code (and other areas of your text life!). It's very simple to pick up; here's a link to a cheatsheet someone put together.
"It works? "
- Yes! To an extent. There are some edge cases that create an unexpected outcome (due to the random numbers). For example, a high number of 4 with a low number of 2 has a chance of outputting 5. Which is not between 4 and 2.
"I'm bad at math."
- As someone who failed a lower level math class 6 times before passing, I totally get where you're coming from! Don't let your inner critic get the best of you.
"I don't get why he subtracts the bottomNumber."
I'm a little confused what you're asking here? There is no subtraction. I'm going to assume this is a typo and you meant "I don't get why he adds the bottomNumber."
Answer: The
bottomNumber
is added to the randomly generated number so that the ideal output is never equal to the lowest number input. If the random number was equal to the lowest number, the sentence output would be wrong. ("1 is a random number between 1 and 10")I'll add comments to your code below if this is confusing.
var topNumber = parseInt( prompt( "Tell me a number" ));
// I input 10
var bottomNumber = parseInt( prompt( "Tell me another, but smaller" ));
// I input 1
var randomNumber = Math.floor( Math.random() * topNumber ) + bottomNumber;
// Lets say Math.random() generates .1111111
// .1111111 * 10 = 1.111111
// Math.floor(1.111111) = 1
// 1 + 1 = 2
// var randomNumber = 2
document.write( randomNumber + " is a random number between " + bottomNumber + " and " + topNumber );
// Prints "2 is a random number between 1 and 10"
// Again, if you had not added the lowest number, the sentence would read "1 is a random number between 1 and 10"
If you made it this far, thank you for reading through my wall of text! I hope you find this answer helpful :D (if you did, please mark this as the best answer and I'll receive sweet points!)
Cheers,
Jacob

Jacob Moyle
6,150 PointsI'm glad you found it helpful! :D
A quick side note; I'm working through the careers program here on tree house. If you found my answer helpful I will get 1 point for an up-vote and/or 12 points if it's marked as the best answer. Just tossing it out there ;D
Rather than writing a lesser answer, I'm going to steal one from this Stack Overflow answer:
There are some examples on the Mozilla Developer Center page:
/**
* Returns a random number between min (inclusive) and max (exclusive)
*/
function getRandomArbitrary(min, max) {
return Math.random() * (max - min) + min;
}
/**
* Returns a random integer between min (inclusive) and max (inclusive)
* Using Math.round() will give you a non-uniform distribution!
*/
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
Here's the logic behind it. It's a simple rule of three:
Math.random() returns a Number between 0 (inclusive) and 1 (exclusive). So we have an interval like this:
[0 .................................... 1)
Now, we'd like a number between min (inclusive) and max (exclusive):
[0 .................................... 1)
[min .................................. max)
We can use the Math.random to get the correspondent in the [min, max) interval. But, first we should factor a little bit the problem by subtracting min from the second interval:
[0 .................................... 1)
[min - min ............................ max - min)
This gives:
[0 .................................... 1)
[0 .................................... max - min)
We may now apply Math.random and then calculate the correspondent. Let's choose a random number:
Math.random()
|
[0 .................................... 1)
[0 .................................... max - min)
|
x (what we need)
So, in order to find x, we would do:
x = Math.random() * (max - min);
Don't forget to add min back, so that we get a number in the [min, max) interval:
x = Math.random() * (max - min) + min;
That was the first function from MDC. The second one, returns an integer between min and max, both inclusive.
Now for getting integers, you could use round, ceil or floor.
You could use Math.round(Math.random() * (max - min)) + min, this however gives a non-even distribution. Both, min and max only have approximately half the chance to roll:
min...min+0.5...min+1...min+1.5 ... max-0.5....max
└───┬───┘└────────┬───────┘└───── ... ─────┘└───┬──┘ ← round()
min min+1 max
With max excluded from the interval, it has an even less chance to roll than min.
With Math.floor(Math.random() * (max - min +1)) + min you have a perfectly even distribution.
min.... min+1... min+2 ... max-1... max.... max+1 (is excluded from interval)
| | | | | |
└───┬───┘└───┬───┘└─── ... ┘└───┬───┘└───┬───┘ ← floor()
min min+1 max-1 max
You can't use ceil() and -1 in that equation because max now had a slightly less chance to roll, but you can roll the (unwanted) min-1 result too.

Jessica Orellanes
11,245 PointsThanks Jacob,
First of all: It really is helpful the markdown thing, i didn't know how to use it. Big thanks for pointing it out.
I also appreciated the detailed, organized analysis, i got a better understanding of what am i doing.
When i said "I don't get why he subtracts the bottomNumber.", I'm referring to the solution Dave (the teacher) implemented.
He did this:
var randomNumber = Math.floor(Math.random() * (topNumber - bottomNumber +1) + bottomNumber);
See? I don't get the difference.
Scott Walton
9,174 PointsScott Walton
9,174 PointsThank you for your explanation. I was struggling with this challenge and you made it crystal clear.