Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial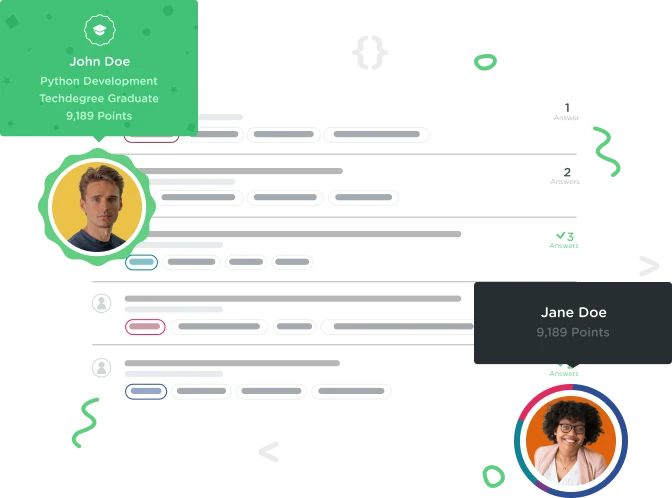

Braveo Yuriy
Courses Plus Student 5,763 PointsPlease, check my solution to see, if it is something to improve.
var students = [
{name: 'Steve', track: 'Web Design', achievements: 130, points: 1407},
{name: 'Gouldie', track: 'iOS Apps', achievements: 240, points: 11451},
{name: 'Bert', track: 'Ruby Developer', achievements: 40, points: 634},
{name: 'Steve', track: 'Python', achievements: 145, points: 1563},
{name: 'Long Bo', track: 'Full-Stack JS', achievements: 256, points: 14653}
];
var request;
var message = '';
var studentList = [];
// Make an array of just names of students
for ( var i = 0; i < students.length; i += 1 ) {
studentList.push(students[i].name);
}
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
message += '<p>Points: ' + student.points + '</p>';
return message;
}
while ( true ) {
request = prompt('Who you need?');
if ( request === 'quit' || request === null ) {
break;
}
// Clear the list before another search operation
message = '';
for (var i = 0; i < students.length; i += 1 ) {
if ( request === students[i].name ) {
getStudentReport(students[i]);
print(message);
}
// test, if there is no matches of names in studentList, then exit the loop immediately
else if ( studentList.indexOf(request) === -1) {
alert('No student found');
break;
}
}
}
3 Answers
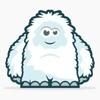
ywang04
6,762 Points@Braveo Yuriy, just found a defect. If you input "Bert" first and then input "Steve" twice. Only two Steve information are shown. However, "Bert" information is gone. I am also facing the same issue. :)

Braveo Yuriy
Courses Plus Student 5,763 PointsIn my code there is a twisted logic. Before entering a loop, I need to check, if my name is in the array of students, and only then make an information report.
if ( studentList.indexOf(request) > -1) {
for (var i = 0; i < students.length; i += 1 ) {
if ( request === students[i].name ) {
getStudentReport(students[i]);
print(message);
}
} else {
alert('No student found');
}

Tomasz Cysewski
2,736 Pointsyeah but after all, congrats for creating it.
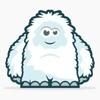
ywang04
6,762 PointsNoticed. The browser behaviour is changed a lot as you said. We have to use "message" as a global variable in the function. After cleaning it, the previous info will be gone.
Braveo Yuriy
Courses Plus Student 5,763 PointsBraveo Yuriy
Courses Plus Student 5,763 PointsI add this option on purpose, actually:). In my code there is a comment: // Clear the list before another search operation You can simply delete this line to see the whole list you input. In this course there was a small tip, said, that newer browsers do not show any information until the loop is done. We can see it only by pressing or typing 'quit'.