Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial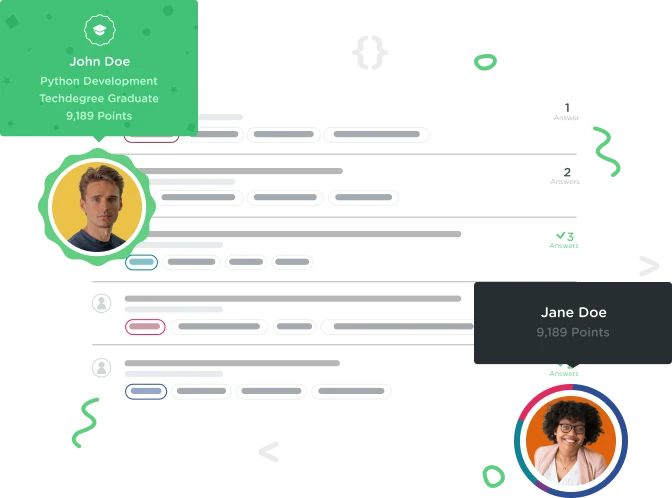
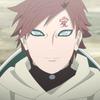
Prasanna kadel
Courses Plus Student 1,057 Pointsplease help
Great work! OK, let's create something a bit more refined. Create a new function named covers_all that takes a single set as an argument. Return the names of all of the courses, in a list, where all of the topics in the supplied set are covered. For example, covers_all({"conditions", "input"}) would return ["Python Basics", "Ruby Basics"]. Java Basics and PHP Basics would be exclude because they don't include both of those topics.
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(a):
l=[]
for keys,value in COURSES.items():
if value&a:
l.append(keys)
return l
1 Answer
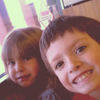
Jason Freitas
6,716 PointsThe key here is to use sets for the comparison, which I think is where you are heading with the "&" or intersection operator in your "if" statement. As you probably know the function argument "a" may include a collection of topics.
In the loop, you will need to convert the list of topics for each "key" into a set, set(COURSES[keys]). How about a statement to check that the length of the output of the intersection is greater than zero?
If the comparison is true, that means there are some common elements.
if len(set(a) & set(COURSES[keys])) > 0: l.append(keys)
Something like that should work...