Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial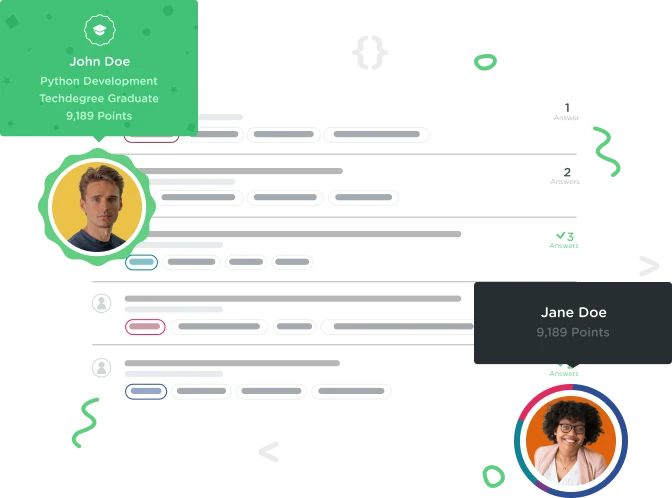

Dolapo Sekoni
Courses Plus Student 1,431 Pointsplease help
please help with this
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teachers):
count = 0
for teacher in teachers:
count += 1
return count
def num_courses(teachers):
count = 0
for course in teachers:
count += 1
return count
2 Answers
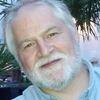
Jeff Muday
Treehouse Moderator 28,720 PointsAny time you are iterating on a dictionary, you need to use the items(), keys(), or values() methods of the dictionary to work with the "key/value" pairs.
Here's an example I typed in to demonstrate...
Python 3.6.4 (v3.6.4:d48eceb, Dec 19 2017, 06:04:45) [MSC v.1900 32 bit (Intel)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> musical_groups = {'Beatles': ['John','Paul','George','Ringo'],
'Van Halen':['David','Eddie','Alex','Wolfgang','Sammy']}
>>> # count the number of keys (groups)
>>> len(musical_groups.keys())
2
>>> # iterate over the keys (groups) and print out
>>> for group in musical_groups.keys():
... print("group name: ", group)
...
group name: Beatles
group name: Van Halen
>>> # iterate over the values (list of group members) and print out
>>> for members in musical_groups.values():
... print("members: ", members)
...
members: ['John', 'Paul', 'George', 'Ringo']
members: ['David', 'Eddie', 'Alex', 'Wolfgang', 'Sammy']
>>>
>>> # now, iterate on items (which allows us to get both the KEY and the VALUE) and print out
>>> for group, members in musical_groups.items():
... print("group: ",group," members: ",members)
...
group: Beatles members: ['John', 'Paul', 'George', 'Ringo']
group: Van Halen members: ['David', 'Eddie', 'Alex', 'Wolfgang', 'Sammy']
>>>
>>> # we can count the members of a specific group by referencing the value
>>> # note, it will throw an error if you don't supply a valid group
>>> def count_members(group):
... members = musical_groups[group]
... return len(members)
...
>>> count_members('Beatles')
4
>>> count_members('Van Halen')
5

Radovan Valachovic
Python Web Development Techdegree Graduate 20,368 PointsHello Dolapo,
What help you need with the code?
John Lack-Wilson
8,181 PointsJohn Lack-Wilson
8,181 PointsHi Dolapo, thanks for posting your code. Could you be more specific about what you need help with?