Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial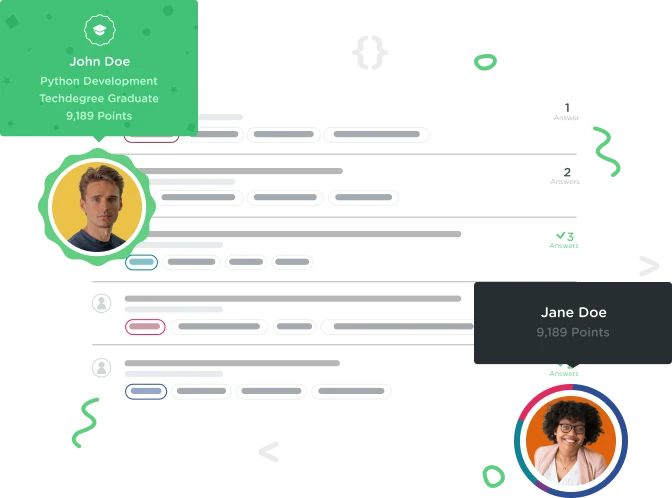

Gyan Yadav
458 PointsPlease help me
Check this code and help me
import random
start = 5
def even_odd():
while start!=0:
random_num = random.randint(1, 99)
new_num = random_num % 2
if new_num != 0:
print("{} is odd".format(new_num))
else:
print("{} is even".format(new_num))
start -=1
#return not num % 2
2 Answers
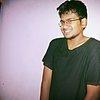
Vidhya Sagar
1,568 PointsWhen you define a function ,avoid the use of global variables.Define start inside the method . And also in your code the variable new_num will hold only the remainder after the division by 2 and not the random number generated by the compiler.I've altered these ,see if it satisfies you. CODE:
import random
def even_odd():
start = 5
while start!=0:
random_num = random.randint(1, 99)
new_num = random_num % 2
if new_num != 0:
print("{} is odd".format(random_num))
else:
print("{} is even".format(random_num))
start -=1
#return not num % 2
a=even_odd()
print(a)
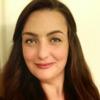
Jennifer Nordell
Treehouse TeacherHi there! The biggest problem here is that you've put everything inside the even_odd
function that's defined by Treehouse. This was not meant to be the case. In fact, Treehouse explicitly states that your code should go outside that function and in the global scope. The purpose of the even_odd
function supplied by Treehouse is to take the number you send to it and send back a value of True
if it's even and False
if it's odd. So this is how I did it:
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while(start):
random_num = random.randint(1, 99)
if even_odd(random_num):
print("{} is even".format(random_num))
else:
print("{} is odd".format(random_num))
start -= 1
As you can see the code starts with us setting the start
variable equal to 5. Then we run the while loop while start is truthy. Inside our while we create a random number. Then we take that random number and send it to the even_odd
function defined by Treehouse. If we get back a value of True for that number then we print out that the number was even. Otherwise, we print that the number was odd. At the end, we decrement our start variable by 1.
Hope this clarifies things! Good luck!