Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial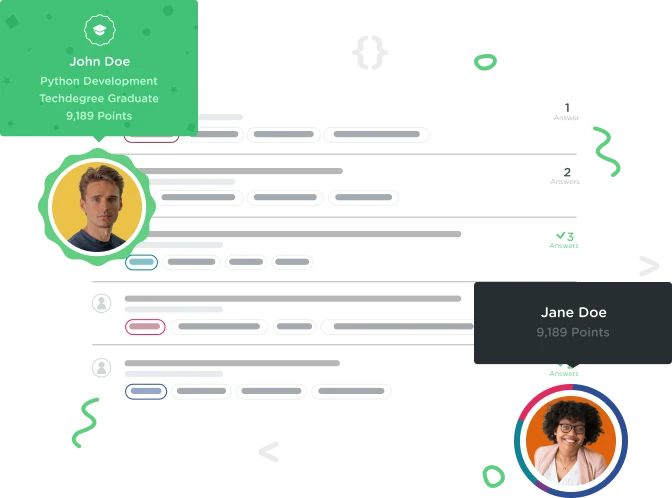

max lemelin
3,407 PointsPlease help with dictionaries 5 part question! I'm on part two
That one wasn't too bad, right? Let's try something a bit more challenging.
Create a new function named num_courses that will receive the same dictionary as its only argument.
The function should return the total number of courses for all of the teachers.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teachers):
return len(teachers)
def num_courses (teachers):
for x in teachers:
return x.keys(teachers)
10 Answers
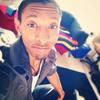
Caleb (Stephen) Gilbert
9,450 PointsI'm heavily stuck on this as well, it's becoming a wall that is frustrating me with the language. I have been all over google and other resources, either I am not understanding the question, or it's just worded in a way I am going about the problem wrong. been trying to look up and figure this answer out since this morning but to avail, and I feel like it wasn't explained too well, or I just missed it? I don't recall learning a method or way to complete this. From basics to collections I feel there is a whole lot I still do not understand. Or grasp about Python, is this really a beginners course? Even rewatching all the videos. So If someone can explain what is wrong and what is right, and explain the solution so I know what I am not getting.
def num_teachers(teacher_class): return len(teacher_class)
def num_courses(teacher_class): for values in teacher_class: return dict.values(teacher_class)
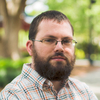
Kenneth Love
Treehouse Guest TeacherWhat's the combined length of all of the lists of courses?
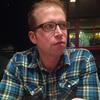
Benjamin Blois
4,530 PointsI got this to work:
def num_courses(teachers):
return sum(len(v) for v in teachers.values())

Gavin Broussard
1,844 Pointscan you or someone explain what exactly this does? what is the alternative to this solution? Are we assigning 'v' as the items in the teacher key? example: v(2) + v(2) = 4?
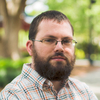
Kenneth Love
Treehouse Guest TeacherThis is taking advantage of Python's implicit generators. The part inside of sum()
, len(v) for v in teachers.values()
becomes a generator, which is kind of like a list and a for loop combined in one but it doesn't make a list (just go with it for now).
Yes, the v
is whatever is inside of an individual teacher's value (which is a list of courses), so len(v)
might return something like 5
or 2
. We'd end up with a generator full of values like (5, 2, 8, 10)
. And then sum()
adds all of those values up, giving something like 28
.
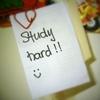
Quinton Rivera
5,177 PointsAmazing only one line of code.

Benjamin Harris
8,872 Pointsif you swap the values with items you get the same answer, only it fails the question?

Adolfo Luciano
Python Web Development Techdegree Student 972 Pointslist_of_values = []
for value in items.values():
list_of_values.append(len(value))
print(list_of_values) #i just used this to see the created list
return sum(list_of_values)
This is basically what the code above would look it if it was expanded

michael brown
5,484 PointsI think the part that is getting me caught up is the question it self. This returns the number of characters in the course names combined. I used a string I made as an example:
teachers = {'teacher 1': 'course 1', 'teacher 2': 'course 2', 'teacher 3': 'course 3', 'teacher 4': 'course 2' }
(The duplicate course was so we could do a comparison and not count the course twice).
The question itself states the total number of courses which in my example above is 3 (not counting the duplicate) or 4 if you wish to count it. However the answer I'm getting back is 32, using the above string, which is the number of characters in the combined values rather then the number of courses.
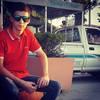
Robert Stepanyan
2,297 Pointsdef num_courses(dictionary):
return sum(map(len,dictionary.values()))

f4u57
6,743 PointsTook quite a bit but I finally got it.
def num_courses(my_dict):
num = 0
for value in my_dict.values():
num += len(value)
return num
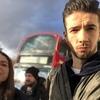
Jan Durcak
12,662 Pointsdef num_courses(a): n=0 for v in a: n += len(a[v]) return n
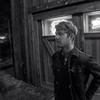
Justin Houghton
4,560 PointsFor anyone getting to this post and confused about Benjamin Blois's challenge solution, I just found a really good blog post by Kenneth Love that is EXTREMELY helpful in explaining what's going on in his solution.

Obaa Cobby
9,337 Pointsdef num_teachers(teachers): return len(teachers.keys())
def num_courses(teachers): return sum(map(len, teachers.values()))
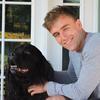
Justin Milner
7,831 Pointsdef num_courses(teachers): result=[] for teacher in teachers.values(): courses = (len(teachers.values())) result.append(courses) return(sum(result))
Can anyone help me understand where I'm doing wrong here?

Terry Felder
1,738 Pointsdef num_courses(a_dict):
new = []
for key,value in a_dict.items():
for values in a_dict[key]:
new.append(values)
return len(new)
so the problem with this challenge is asking to count the numbers of courses a teacher has right?? so my fuction does that just fine.....only if the value is a list! so lets say in the example {'Kenneth Love': ['Python Basics', 'Python collections']}....if Kenneth decided to teach just 'python basics'. then the dictionary key/value pair is now in stead, {'Kenneth Love': 'Python basics'} so now when you look for the length or sum or iterate through the values...you will iterate through each letter and space now....
im am almost 100% sure if we fix this problem in the function it will pass.....any ideas or help im all ears but like i said im sure we are all on the right track we are just missing how to count the value if it is not a list datatype..

Anthony Stephens
Full Stack JavaScript Techdegree Student 3,328 Pointsdef value_count(dict):
x=0
for name in dict :
x += len(dict[name])
return x
I don't understand why this wont work for me. It does in fact return an int of the proper amount of classes being taught by all professors.