Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial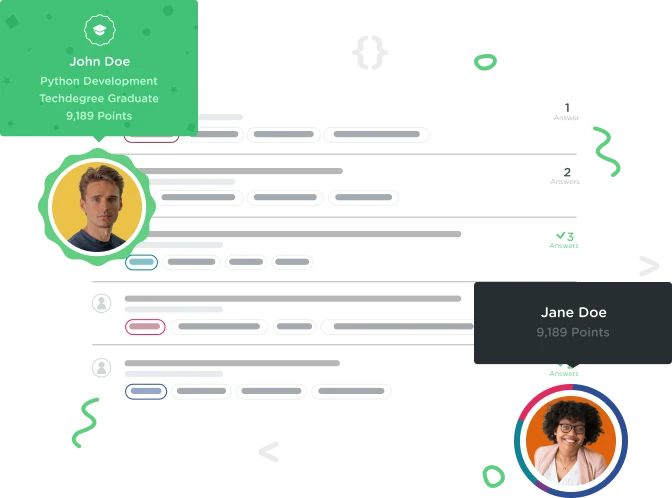

Fikreyesus Desta
4,009 PointsPlease let me know what my mistake is.
Stuck here
var counter = 0;
while (counter < 10 ) {
var randNum = randomNumber (6);
document.write (randNum + ' ');
counter += 1;
}
3 Answers
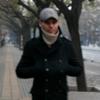
Matthew Main
7,566 PointsIt looks like in line three you'd like to declare a variable as a random number between 1 and 6. Is that right?
If so, to create a random integer between 1 and any number, use:
Math.ceil(Math.random() * anyNumber )
Arriving at random integers is a bit convoluted in JavaScript. "Math.random()" chooses a random decimal number between 0 and 1. Multiplying that decimal number by "any number"βin your case, 6βcreates a random decimal number between 0 and 6. "Math.ceil" rounds that decimal number up to the integer, giving you a random integer between 1 and 6.
So if your goal is to print 10 random numbers between 1 and 6, try rolling the dice like this:
var counter = 0;
while ( counter < 10 ) {
var randNum = Math.ceil(Math.random() * 6 );
document.write (randNum + ' ');
counter += 1;
}

Daniel Newman
Courses Plus Student 10,715 PointsProblem of your code is here also:
while( ... ) {
document.write (randNum + ' ');
}
In this code you are erasing content of your html page with new data. You should use additional variable to store whole data and then, after loop, write it on your page.
For example:
var htmlData = '';
while( ... ) {
htmlData = htmlData + newData;
}
document.write(htmlData);
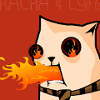
Brian Romine
9,518 PointsDo you have randomNumber defined as a function? you need to use Math.random() at some point to get a random number...