Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial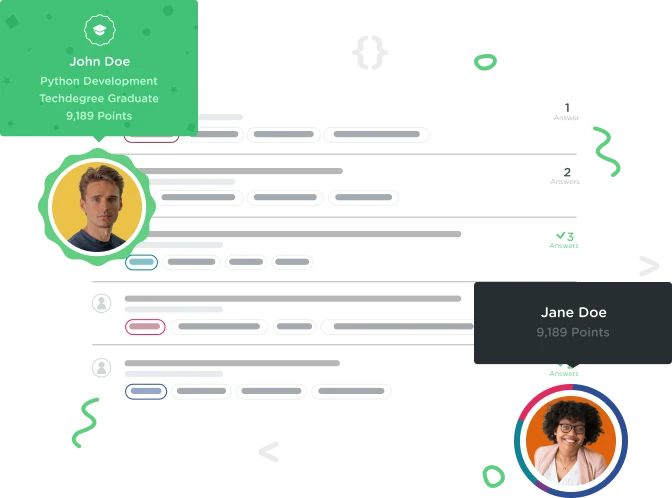
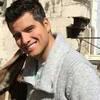
Javier MARQUEZ
11,877 PointsPlease take a look; In Firefox everything works, but somehow I always get 'undefined' at the top of the results
Thanks a lot for all your help, I seem to have nailed this challenge but I always get the 'undefined' word at the beggining of the results, and when using chrome the results are printed on the screen only after hitting 'esc' or quitting.
var message = '';
var student;
var search;
var found;
var searchResults = [];
//Printing inside the div with the output id
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//Returning all information for all students
function allstudents(listStudents){
var allStudentlist;
for (var i = 0; i < listStudents.length; i += 1) {
student = listStudents[i];
allStudentlist += '<h2>Student: ' + student.name + '</h2>';
allStudentlist += '<p>Track: ' + student.track + '</p>';
allStudentlist += '<p>Points: ' + student.points + '</p>';
allStudentlist += '<p>Achievements: ' + student.achievements + '</p>';
}
return allStudentlist;
}
//Returns information for one student only
function getStudentReport ( student ){
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
//Creates a n array of results
function resultsArray ( array ) {
searchResults = []; //Reinitilaizing outside the loop
for (var i = 0; i < array.length; i += 1) {
student = array[i];
if (student.name.toLowerCase() === search.toLowerCase() ){
found = true;
searchResults.push(student);
}
}
return searchResults;
}
while (true) {
search = prompt("Let's search for an student, type 'all' for all students and 'quit' to exit");
//exits if quit or null
if ( search === null || search.toLowerCase() === 'quit' ){
break;
} if (search.toLowerCase() === 'all' ){ //Searching for all students
found = true;
message = allstudents(students);
print(message);
break;
}
resultsArray(students); //calling a function to create a temporary array with the results
if (typeof searchResults !== 'undefined' && searchResults.length > 0) { //if the results are there print the message
message = allstudents(searchResults);
print(message);
}
if (!found ) {
alert("There is no student with the name " + search + ".");
}
}
1 Answer
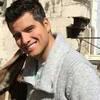
Javier MARQUEZ
11,877 PointsYou sir are the real MVP.
Thanks a lot, very clear answer. It works now.
Keen Learner
6,126 PointsKeen Learner
6,126 PointsThe problem is really tiny and is probably one of the common issues faced in javascript.
To quote MDN's description of undefined - undefined is a property of the global object, i.e. it is a variable in global scope. The initial value of undefined is the primitive value undefined. A variable that has not been assigned a value is of type undefined. A method or statement also returns undefined if the variable that is being evaluated does not have an assigned value.
Now having understood this, we observe that variable allStudentlist hasn't been initialized or assigned a value (line 1) before appending (with the += operator) more data to it (line 3).
Simply initializing it on declaration will resolve your issue.
var allStudentlist = '';
Hope this helps.