Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial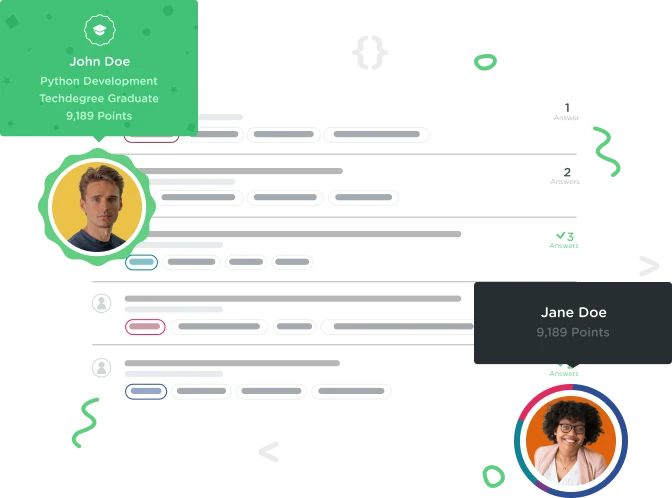

Jeremy Juengel
Courses Plus Student 5,286 PointsPossible solution to multiple entries of the same or similar names.
While not a perfect solution, as it has its own shortfalls, it does answer the question on how to get around multiple student entries with the same name.
I used the string.search('search for this') method. This will return any entry that contains the information you are searching for. I used it like this:
function searchStudent(searchName)
{
for (var i = 0; i < students.length; i++)
{
student = students[i];
if ( !( ( ( student.Name.toLowerCase() ).search(searchName) ) ) )
{
message += '<h2>' + student.Name + '</h2>';
message += '<p> Track: ' + student.Track + '</p>';
message += '<p> Points: ' + student.Points + '</p>';
message += '<p> Achv: ' + student.Achievements + '</p>';
}
}
print(message);
}
*** Note the "!" used before the 2nd parentheses in the IF statement. I found that if I did not "NOT" this statement, it would return everything BUT what i searched for. Since i couldn't figure out why that was happening, i just inverted the results. ***
The advantage of using the ".toLowerCase()" method is that if the search is cased correctly, it will still return the value. In other words, "Jody", "jody", and "JODY" will still yield the same results.
Anyway, that is how i solved the entries with the same name challenge!