Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial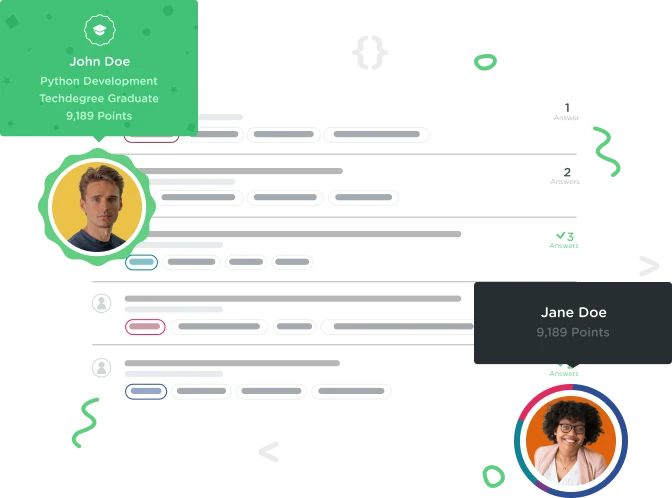
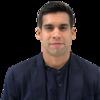
Johnny Garces
Courses Plus Student 8,551 PointsPrint function displays only one student instead of all students' names. Why is that?
Here's my problem- I can't get the print function to display all student names.
However I'm able to console log all student names. How can I get the function print all names?
Here's my code. I think the problem may lie in the "for loop".
any suggestion is greatly appreciated!
var students = [
{
name: 'Bob',
track: ['Javascript', 'iOS', 'Web Design'],
achievements: 3,
points: 120
},
{
name: 'Jane',
track: ['Android', 'iOS', 'Ruby on Rails'],
achievements: 3,
points: 110
},
{
name: 'Alex',
track: ['PHP', 'iOS', 'Web Design', 'CSS'],
achievements: 4,
points: 150
},
{
name: 'Rose',
track: ['Java'],
achievements: 1,
points: 30
},
{
name: 'Mia',
track: ['Javascript', 'iOS', 'Android', 'Ruby on Rails', 'Perl'],
achievements: 5,
points: 140
},
];
//print function//
function print(message){
var div = document.getElementById('output');
div.innerHTML = message;
return message;
}
for(var i=0; i<students.length; i++){
var student = students[i];
var studentName = students[i].name;
console.log(studentName);
message = '<p>Student: '+studentName + '</p>';
}
print(message);
3 Answers
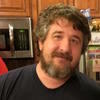
Michael Hall
Courses Plus Student 30,909 PointsYour message is only set once. So each time the function goes through, it creates a new message. So you are only getting the last name. Build the message with +=. Also, you should create the message variable outside the function, you can set it to an empty string or just leave it blank.
var students = [
{
name: 'Bob',
track: ['Javascript', 'iOS', 'Web Design'],
achievements: 3,
points: 120
},
{
name: 'Jane',
track: ['Android', 'iOS', 'Ruby on Rails'],
achievements: 3,
points: 110
},
{
name: 'Alex',
track: ['PHP', 'iOS', 'Web Design', 'CSS'],
achievements: 4,
points: 150
},
{
name: 'Rose',
track: ['Java'],
achievements: 1,
points: 30
},
{
name: 'Mia',
track: ['Javascript', 'iOS', 'Android', 'Ruby on Rails', 'Perl'],
achievements: 5,
points: 140
},
];
//print function//
var message = '';
function print(message){
var div = document.getElementById('output');
div.innerHTML = message;
return message;
}
for(var i=0; i<students.length; i++){
var student = students[i];
var studentName = students[i].name;
console.log(studentName);
message += '<p>Student: '+studentName + '</p>';
}
print(message);
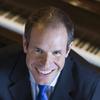
Sam Shiffman
7,466 PointsHi Johnny, I encountered this same problem too. This print function will run the first iteration (when i = 0) and that's all that will appear on the page. For each loop you have to += to the message. Then AFTER the for loop is finished you call the print(message) command. Give me another minute and I will show you the modified code in a Comment.
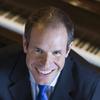
Sam Shiffman
7,466 PointsJohnny, I added a new line var message; to declare the variable message. Notice that in the last command in the for loop I changed the = to +=.
```var message; // Declare message variable
function print(message){ var div = document.getElementById('output'); div.innerHTML = message; return message; }
for(var i=0; i<students.length; i++){
var student = students[i]; var studentName = students[i].name; console.log(studentName); message += '<p>Student: '+studentName + '</p>'; // Append student name to message
}
print(message);
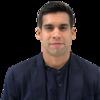
Johnny Garces
Courses Plus Student 8,551 Pointsthx sam! it's strange that I could get all the names with console log, but with print i had to iterate over the message by doing message+=
this could be a js ineffeciency.
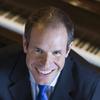
Sam Shiffman
7,466 PointsSPOILER ALERT This comment provides a partial solution to a Coding Challenge later on in the course concerned here. It's not the same as the instructor's solution, but it works
Johnny, I am still relatively new to JavaScript and I have been wondering the very same thing. When I was solving the 'quiz' challenge in this course I hacked out various attempts to print lists to ol and li elements. The only way I could get them to work was to build a message variable piece by piece and print the message after the entire html structure was complete, like so:
var correct = [];
var wrong = [];
var question;
var answer;
var response;
var reportMessage = "";
function print(message, elementId) {
var outputElement = document.getElementById(elementId);
outputElement.innerHTML = message;
}
function listQuestions(list, rightWrong) {
reportMessage += "<h2>You got these " + list.length + " questions " + rightWrong + "</h2>";
reportMessage += "<ol>";
for (var i = 0; i < list.length; i++) {
reportMessage += "<li>" + list[i] + "</li>";
}
reportMessage += "</ol>";
}
listQuestions(correct, "correct");
listQuestions(wrong, "wrong");
print(reportMessage, "output");
I'm guessing that either the getElementById() function or the innerHTML() function will only access the html element one time after the page loads, so you need to populate your 'message' with EVERYTHING and call the print() function once. (Notice my own modified print() function, which takes a 2nd argument so you can select the html element you want to write to.)
Johnny Garces
Courses Plus Student 8,551 PointsJohnny Garces
Courses Plus Student 8,551 Pointsthx for the answer, michael. this worked. however, i'm still curious as to how console log was able to output all the names simultaneously.
Michael Hall
Courses Plus Student 30,909 PointsMichael Hall
Courses Plus Student 30,909 Pointsconsole.log is getting called each time through the function. So each time it's given a new name to log. The reason this didn't work with message was because of the difference between '=' and '+='. '+=' takes the variable and adds to it. '=' ignores whatever was previously in the variable and assigns the new value.