Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial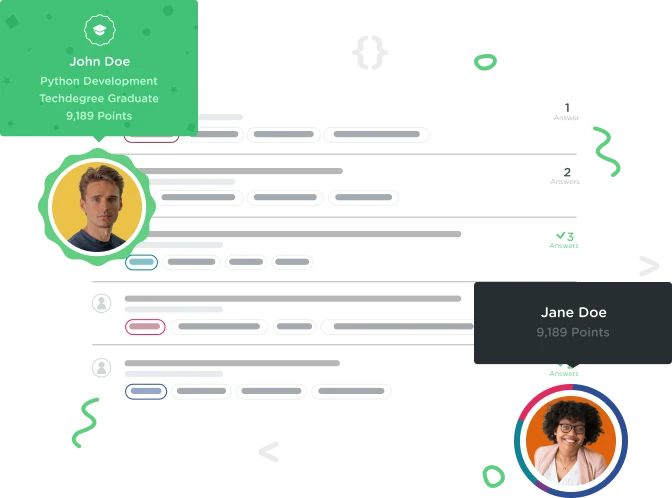
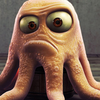
Laurence Foley
16,695 PointsProblem with student record while loop
In my while loop I first ask the user for input and then store that data in a variable, then I have a few if/else statements that will print out a student record to the browser depending on what name the user types. This works fine the first time the loop runs and will print out the correct student record but when you try and print out a second record it will print out that student record plus the previous record.
<script type="text/javascript" src="student_records.js"></script>
<script type="text/javascript">
var search;
var message = '';
var student;
function print(message) {
document.write(message);
}
function printStudent(number) {
student = students[number];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievments: ' + student.achievments + '</p>';
print(message);
}
while (search !== 'QUIT') {
var search = prompt().toUpperCase();
if (search === students[0].name.toUpperCase()) {
printStudent(0);
} else if (search === students[1].name.toUpperCase()) {
printStudent(1);
} else if (search === students[2].name.toUpperCase()) {
printStudent(2);
} else if (search === students[3].name.toUpperCase()) {
printStudent(3);
}
}
</script>```
2 Answers
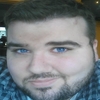
Marcus Parsons
15,719 PointsAh, I see what's happening. Your message
variable is in the global context, and every time the printStudent()
function launches, the message
variable is being added to, making each previous call to message appear again stacked on top of the last call made and all previous calls. You can solve this by adding message = '';
after the print(message);
line in the printStudent()
function so that message will be cleared of all previous calls.
I also got rid of the print function; it causes problems with Safari and is much slower than just using document.write() by itself. Also, there was a spelling error on the property "achievements" in the printStudent()
function:
var search;
var message = '';
var student;
function printStudent(number) {
student = students[number];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievments: ' + student.achievements + '</p>';
document.write(message);
message = '';
}
while (search !== 'QUIT') {
var search = prompt("Enter a student name, or type 'quit' to quit.").toUpperCase();
//A much more modular, dynamic approach that will allow you to use as many students as you wish in your array
//If you have 100 students in your data, you don't want to have to do 100 if/else-if statements to accommodate that
//This approach solves that dilemma
for (var i = 0; i < students.length; i += 1) {
if (search === students[i].name.toUpperCase()) {
printStudent(i);
}
}
}
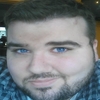
Marcus Parsons
15,719 PointsHey Laurence,
That's the way document.write()
works. document.write()
(on its very first call) erases any content currently on the page. All subsequent calls to document.write()
result in appending data to the document.
By the way, you can make your code much more efficient and modular by utilizing a loop instead of an if/else/else-if structure. Also, it is much faster to just document.write()
instead of a custom print()
function. You can see a test case of that here at this JS performance test: http://jsperf.com/custom-functions-vs-no-functions
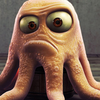
Laurence Foley
16,695 PointsHi Marcus,
Thanks for the reply. I don't think I explained clearly what I am confused about. What I don't understand is when the program runs and the user is prompted to type a name say Joe for example the program will print out:
Student: Joe.
after that the user is prompted again and say the user this time types Bob, the document will show this:
Student: Joe.
Student: Joe.
Student: bob.
Why is Joe being duplicated?
Laurence Foley
16,695 PointsLaurence Foley
16,695 PointsThanks alot Marcus.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou are very welcome, Laurence!