Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial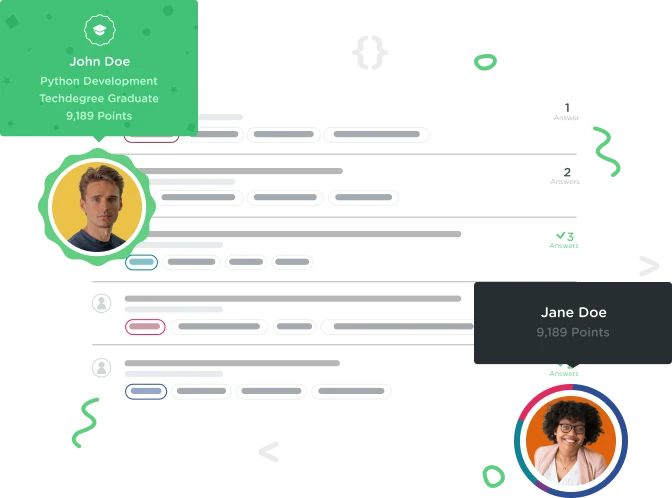

none ofyourbusiness
2,407 PointsProgram not keeping track of correct answers?
The Quiz Challenge program is not keeping track of correct answers. Not sure what I'm doing wrong.
/*
Quiz Application
*/
// Quiz begins, no answers correct
var correct = 0;
// Asking five questions
var answer1 = prompt("Name a programming language that is also a gem.");
if (answer1.toUpperCase === "RUBY") {
correct += 1;
}
var answer2 = prompt("Name a programming language that is also a snake.");
if (answer2.toUpperCase === "PYTHON") {
correct += 1;
}
var answer3 = prompt("What programming language do you use to style web pages?");
if (answer3.toUpperCase === "CSS") {
correct += 1;
}
var answer4 = prompt("What prograaming language do you use to structure web pages?");
if (answer4.toUpperCase === "HTML") {
correct += 1;
}
var answer5 = prompt("What programming language do you use to add interactivity to a web page?");
if (answer5.toUpperCase === "JAVASCRIPT") {
correct += 1;
}
// OUTPUT RESULTS
document.write("<p> You got " + correct + " out of five questions correct. </p>");
// OUTPUT RANK
if (correct === 5) {
document.write("<p><strong> You earned a gold crown!</strong></p>");
} else if (correct >=3 ) {
document.write ("<p><strong> You earned a silver crown! </strong></p>");
} else if (correct >=1 ) {
document.write ("<p><strong> You earned a bronze crown! </strong></p>");
} else {
document.write ("<p><strong> You earned no crown. Try again! </strong></p>");
}

none ofyourbusiness
2,407 PointsThanks Mike!
4 Answers
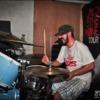
Mike Costa
Courses Plus Student 26,362 PointsThe response coming back from the prompt would be whatever you type into the prompt. So if you type in "Denver" for the first answer, answer1.toUpperCase() will really be "DENVER". Your conditional statement is not checking that case. Change all the conditionals to uppercase words, (DENVER, BASKETBALL, JAYHAWK, ect) and you should be good. :)

Kate Petry
Courses Plus Student 9,432 PointsI am having the exact same problem - the quiz is not keeping track of the correct answers. I do not see my error. I have tried to compare my code to how Dave has set his up but I don't see any major discrepancies. The only thing I noticed in Dave's code was that after answer1.toUppercase there was the parenthesis () but for the other answer toUppercase the parentheses were not included. I have tried both and still am not having any luck. So far I only tried to get the label for gold crown and b/c I was unable to get the correct # to show with that I did not complete the else ifs statements for the remaining ranks. Could that be my problem. Any suggestions would be most appreciated.
//quiz begins, no answers correct
var correctGuess = 0;
//ask questions
var answer1 = prompt('What city do the Denver Broncos play in?');
if (answer1.toUpperCase() === "Denver") {
correctGuess += 1;
}
var answer2 = prompt('What is the mascot of KU?');
if (answer2.toUpperCase() === "Jayhawk") {
correctGuess +=1;
}
var answer3 = prompt('What sport includes a traveling violation?');
if (answer3.toUpperCase() === "Basketball") {
correctGuess += 1 ;
}
var answer4 = prompt('What is your husbands name?');
if (answer4.toUpperCase() === "Thad") {
correctGuess += 1;
}
var answer5 = prompt('What is the name of your current favorite TV show?'); {
if (answer5.toUpperCase() === "Castle") {
correctGuess += 1;
}
}
//output results
document.write("<p>You got " + correctGuess + " out of 5 questions correct.</p>");
//output rank
if ( correctGuess === 5) {
document.write("<p><strong>You earned a gold crown!</strong></p>");
}

Kate Petry
Courses Plus Student 9,432 PointsMike - thanks so much that worked, but I'm not sure I understand why. I thought my converting answer1 to upperCase allowed for the answer to be written as either Denver, denver or DENVER? So if i set answer1.toUpperCase() does that mean whatever I set it to needs to be listed as all capitals?
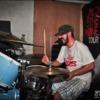
Mike Costa
Courses Plus Student 26,362 PointsWhen you use the toUpperCase function, the return value will be all capital letters.
lets take this as an example:
var answer1 = prompt('What city do the Denver Broncos play in?');
The prompt comes up and you can write anything in that prompt box, which means the variable "answer1" will be what you entered. So if you wrote "dEnVeR" in the prompt box, answer1 will be equal to "dEnVeR". When you call toUpperCase() on answer1, it converts that string to "DENVER". The conditional with the way you had it
if (answer1.toUpperCase() === "Denver")
is saying
if ("DENVER" === "Denver")
which is not equal.
So in order to properly test that condition, you'd need to account for what you think the return value of answer1.toUpperCase() would be. So if the correct answer you want someone to put in the prompt box is "Denver", you would use
if (answer1.toUpperCase() === "DENVER")
the reason being is that "DENVER" is the correct answer, and no matter which way the user types it into the prompt box, ("denVER", "DeNvEr", "denveR", ect), you're forcing the users answer to be in all capital letters with toUpperCase() to match your correct answer.

Kate Petry
Courses Plus Student 9,432 PointsThanks Mike. This explanation was perfect and just what I needed to make sense of things. Thank your for the well thought out response!
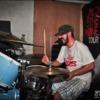
Mike Costa
Courses Plus Student 26,362 PointsGlad i can help! :)
Mike Costa
Courses Plus Student 26,362 PointsMike Costa
Courses Plus Student 26,362 PointstoUpperCase is a function. You'll have to call it like this
if (answer1.toUpperCase() === "RUBY") { correct += 1; }