Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial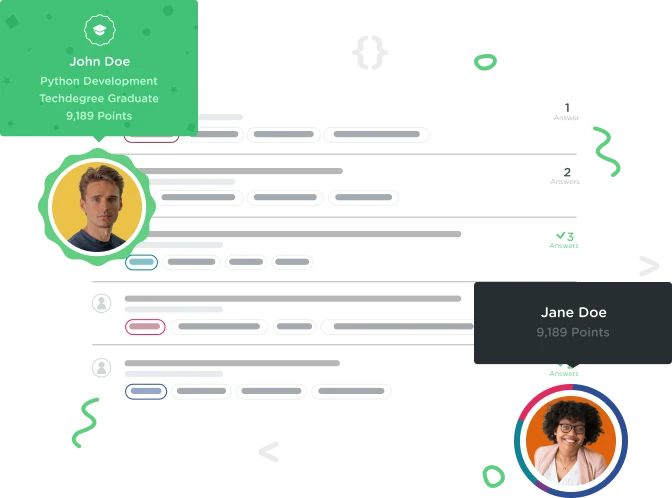

Thea Van Kessel
2,058 PointsPrompt isn't popping up
When I follow along with Dave on "The Random Challenge Solution" I can never get the prompts to pop up when I preview it. I've reviewed the code over and over and it seems to look just like his but it just doesn't work. Also when I go to the JavaScript console it says "Uncaught SyntaxError: Unexpected String" and this error is on line 6.
Here is my code.
var input1 = prompt("Please type a starting number"); var bottomNumber = parseInt(input1); var input = prompt("Please type a number"); var topNumber = parseInt(input); var randomNumber = Math.floor(Math.random() * (topNumber - bottomNumber +1)) + bottomNumber; var message = "<p>" + randomNumber + " is a number between " + bottomNumber + "and " + topNumber ".</p>"; document.write(message);
Line six is: var message = "<p>" + randomNumber + " is a number between " + bottomNumber + "and " + topNumber ".</p>";
1 Answer
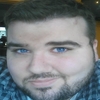
Marcus Parsons
15,719 PointsHi Thea,
The reason why the prompt will not show up is because there is a missing + operator in the message
variable right after topNumber
. A side note, you don't have to put an empty string when concatenating with strings and numbers. Here's the fixed code, Thea! :)
var input1 = prompt("Please type a starting number");
var bottomNumber = parseInt(input1);
var input = prompt("Please type a number");
var topNumber = parseInt(input);
var randomNumber = Math.floor(Math.random() * (topNumber - bottomNumber + 1)) + bottomNumber;
var message = randomNumber + " is a number between " + bottomNumber + " and " + topNumber + ".";
document.write(message);
You can also make this code shorter by parsing the input from the prompt right away:
var bottomNumber = parseInt(prompt("Please type a starting number"));
var topNumber = parseInt(prompt("Please type a number"));
var randomNumber = Math.floor(Math.random() * (topNumber - bottomNumber + 1)) + bottomNumber;
var message = randomNumber + " is a number between " + bottomNumber + " and " + topNumber + ".";
document.write(message);
Thea Van Kessel
2,058 PointsThea Van Kessel
2,058 PointsI even copy and pasted your corrected code to the text editor and the prompt box still won't pop up. It also still says that there is unexpected string on line six and when I delete line six completely I can get both prompt boxes to show up.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHmm, I know the code is correct because I tested in the console before posting it, but I don't know what your original code looks like. Are you working on this in Workspaces?
Thea Van Kessel
2,058 PointsThea Van Kessel
2,058 PointsThank you! I'm not sure what the problem was but it's working now after closing and reopening it a few times. Thank you for your help.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAh, excellent! I'm glad to have helped you. Happy Coding, Thea! =]