Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial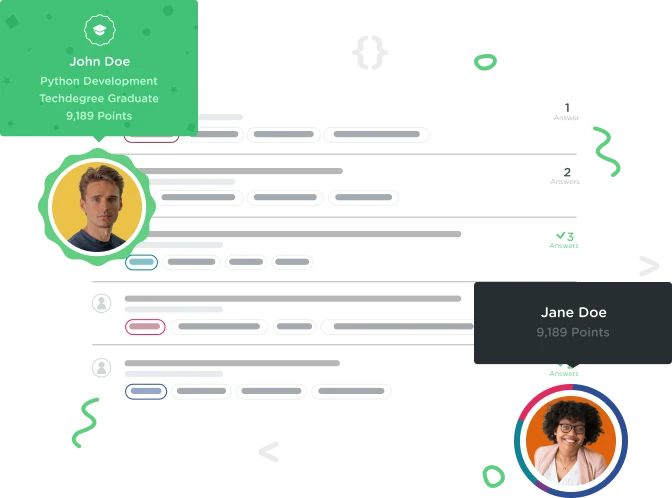
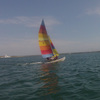
Wiley Conte
9,618 Points.push issues inside a .each loop
Hi all thanks for taking the time to help if you are able. Desired output is [[direction, north], [direction, south], [direction, east]] . An array of arrays. Here is my test code that I want to run:
sentence = "north south east"
words = sentence.split
array_sentence = []
words.each do |word|
if word.include?('north' || 'east' || 'west' || 'south')
array_sentence.push(['direction', word])
end
end
puts array_sentence
From my testing, it seems the .push line is causing the loop to run through only once, and the output is [direction, north] when it should have all three items from my sentence. Thank you in advance!
2 Answers

andren
28,558 PointsWhen you are trying to debug an issue (especially when looping), an easy way to get information is to place a bunch of puts
statements in your code to get an idea of what is happening while your code runs.
Like this for example:
sentence = "north south east"
words = sentence.split
array_sentence = []
words.each do |word|
puts "The word is: " + word
puts "Result of check is: " + word.include?('north' || 'east' || 'west' || 'south').to_s
if word.include?('north' || 'east' || 'west' || 'south')
array_sentence.push(['direction', word])
end
end
puts array_sentence
If you run that code the result will be this:
The word is: north
Result of check is: true
The word is: south
Result of check is: false
The word is: east
Result of check is: false
So you can see that the loop is indeed running the correct number of times, however the check you have written only works when "north" is passed in.
The reason for this is that you cannot pass multiple strings to the include
method, that's not how the ||
operator works. If you wanted to use ||
and include
to check multiple strings then you would have to call the method multiple times.
Like this:
sentence = "north south east"
words = sentence.split
array_sentence = []
words.each do |word|
if word.include?('north') || word.include?('east') || word.include?('west') || word.include?('south')
array_sentence.push(['direction', word])
end
end
puts array_sentence
That would work, but there are more efficient ways of doing this type of check. One pretty simple one would be to place the strings you are looking for in an array and then call include
to check if the word
is included in the array.
Like this:
sentence = "north south east"
words = sentence.split
array_sentence = []
words.each do |word|
if ["north", "east", "west", "south"].include?(word)
array_sentence.push(['direction', word])
end
end
puts array_sentence
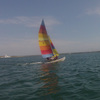
Wiley Conte
9,618 PointsThank you - very helpful!