Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial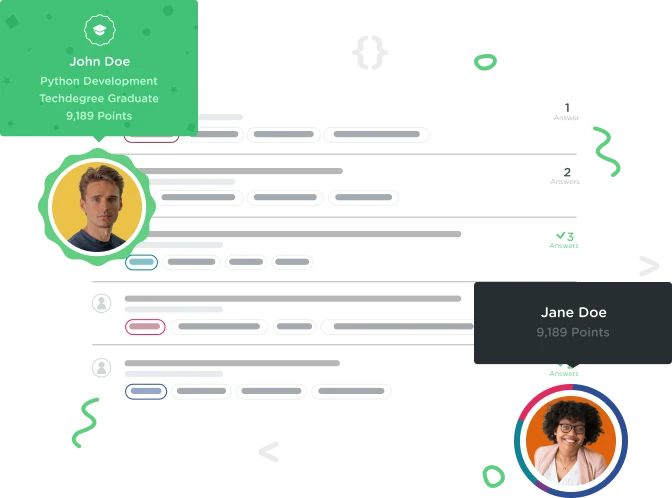

Ozhan Saat
Courses Plus Student 4,002 PointsPush notification for estimote beacons
Hi all,
I am working on an app that uses Estimote beacons and so far I've been following the docs at http://developer.estimote.com/android/tutorial/part-2-background-monitoring/.
At the moment the push notification gets it's values from two hard coded strings but I want to change that to text from two edit text fields. I created the Edit text fields in the layout and made a getter class but I can't seem to use the text in the notification. The notification comes back blank.
Below is my code.
'''public class MainActivity extends AppCompatActivity {
private EditText eventTitle;
private EditText message;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final String title = eventTitle.getText().toString();
final String messageBody = message.getText().toString();
if (title.length() == 0) {
eventTitle.requestFocus();
eventTitle.setError("Field cannot be empty");
} else if (message.length() ==0) {
message.requestFocus();
message.setError("Field cannot be empty");
} else {
Toast.makeText(MainActivity.this, "Reminder Successful", Toast.LENGTH_LONG).show();
}
}
});
eventTitle = (EditText) findViewById(R.id.eventTitle);
message = (EditText) findViewById(R.id.message); '''
'''public class Reminders {
public String getEventTitle() {
return eventTitle;
}
public String getMessage() {
return message;
}
String eventTitle;
String message;
} '''
'''public class MyApplication extends Application {
private BeaconManager beaconManager;
@Override
public void onCreate() {
super.onCreate();
Reminders reminders = new Reminders();
final String title = reminders.getEventTitle();
final String message = reminders.getMessage();
beaconManager = new BeaconManager(getApplicationContext());
beaconManager.setMonitoringListener(new BeaconManager.MonitoringListener() {
@Override
public void onEnteredRegion(Region region, List<Beacon> list) {
showNotification(
title, message
);
}
@Override
public void onExitedRegion(Region region) {
}
});
beaconManager.connect(new BeaconManager.ServiceReadyCallback() {
@Override
public void onServiceReady() {
beaconManager.startMonitoring(new Region("monitoring region", UUID.fromString("B9407F30-F5F8-466E-AFF9-25556B57FE6D"),
2982, 61870));
}
});
}
public void showNotification(String title, String message) {
Intent notifyIntent = new Intent(this, MainActivity.class);
notifyIntent.setFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP);
PendingIntent pendingIntent = PendingIntent.getActivities(this, 0, new Intent[] { notifyIntent }, PendingIntent
.FLAG_UPDATE_CURRENT);
Notification notification = new Notification.Builder(this)
.setSmallIcon(android.R.drawable.ic_dialog_info)
.setContentTitle(title)
.setContentText(message)
.setAutoCancel(true)
.setContentIntent(pendingIntent)
.build();
notification.defaults |= Notification.DEFAULT_SOUND;
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.notify(1, notification);
}
'''
I'd appreciate any help on this. Thanks
Ozhan Saat
Courses Plus Student 4,002 PointsOzhan Saat
Courses Plus Student 4,002 PointsThis is for anyone who might have a similar issue. I spent too long trying to work this out. When I finally did, I felt a little stupid for taking so long because the answer was quite simple. It's probably not the best way to do it but it works so I'll take it.
First, from MyApplication I deleted
''' Reminders reminders = new Reminders(); final String title = reminders.getEventTitle(); final String message = reminders.getMessage(); '''
and added
''' private String title;
'''
Then, in MainActivity, I added this to onCreate
''' eventTitle = (EditText) findViewById(R.id.eventTitle); message = (EditText) findViewById(R.id.message);
'''
That's all it took. I spent so long trying to solve this when it should have taken minutes. It was because I was overlooking some key concepts of OOP programming. I'm looking forward to stressing about the next step in this very simple app.