Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial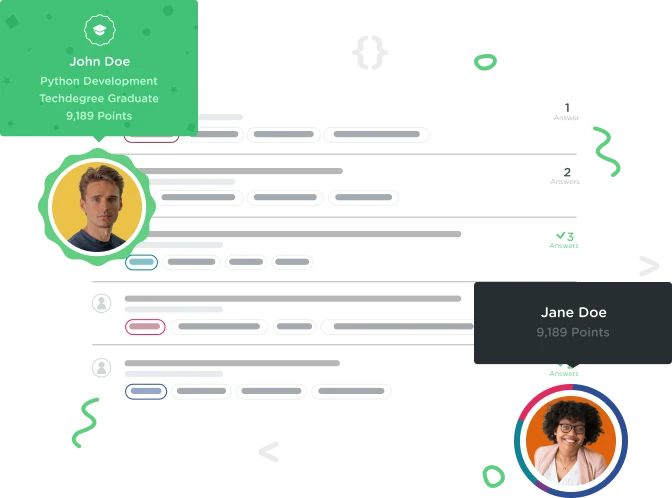

Shadow Skillz
3,020 PointsPython collection Challenge Task 2 of 5 ?
Not sure how to go about returning the sum of all classes. I can create one variable that returns the amount of one teacher but not to sure how to create to variable's that holds the classes so I can add them up. Anybody's help would be greatly appreciated.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dic):
return len(dic)
def num_courses(my_dict):
teachers_list = {}
for teacher in my_dict:
teachers_list[teacher] = len(my_dict[teacher])
for T in my_dict:
teachers_list[T] = len(my_dict[teacher])
return len(teachers_list )
2 Answers
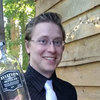
Evan Demaris
64,262 PointsHi Christian,
One of the ways to solve task 2 would be to use a single loop which counts the length of each teacher's array of classes. As long as we don't have teachers who both teach the same class, that should adequately solve the task.
def num_courses(my_dict):
count = 0
for teacher in my_dict:
count += len(my_dict[teacher])
return count
Hope that helps!
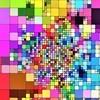
james south
Front End Web Development Techdegree Graduate 33,271 Pointsright now your for loops are redundant. one way to reach the values of each key in the dict so you can count them would be to nest the second for loop (indent it so it is in the block of the first loop) rather than put it under the first loop. the first loop is like you have, looping through the keys. the second loop must loop through the values associated with each key. you know how to get those, so then you just append the values, which is your second loop variable, to your list and return its length.

Shadow Skillz
3,020 PointsThanks for the help. I don't think you can append to a dictionary. can you give me a visual aid for this challenge
Shadow Skillz
3,020 PointsShadow Skillz
3,020 PointsEvan thank you so much for the help bro. For the life of me, I couldn't figure out the problem. It goes to show my coding perception is still quite juvenile. Once again thanks mill.