Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial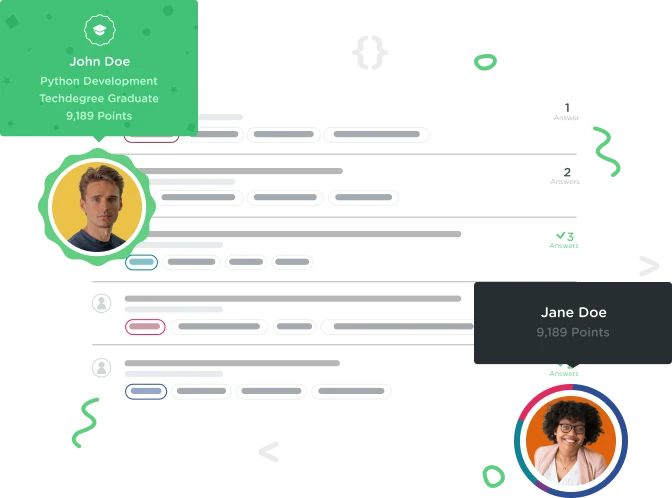

Brian Willeford
1,830 PointsPython Collections Challenge "Bummer! Didn't get all of the expected output from string_factory()" and have no idea why
I have been investigating the issue on other asked questions and tried to solve this, but I'm getting the error "Bummer! Didn't get all of the expected output from string_factory()" when checking it. I don't know what's going on with it... Any help would be appreciated!
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
dict_list = [
{'name': 'Brian', 'food': 'pizza'},
{'name': 'Jimmy', 'food': 'dirt'},
{'name': 'Snake', 'food': 'mice'},
{'name': 'Roger', 'food': 'Booze'}
]
string = "Hi, I'm {name} and I like to eat {food}"
def string_factory(dict_list):
new_list = []
for item in dict_list:
new_list.append(string.format(**item))
return new_list
2 Answers
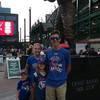
AJ Salmon
5,675 PointsHey Brian!
At first glance I thought I saw the issue: there was no exclamation mark at the end of your string, where the original template used an exclamation mark. This would, in fact, prevent you from passing the challenge, but after fixing that and running it, it still wouldn't pass. I honestly am not sure why. Then, I left the original template string in the code, and left everything else the same as you had it, save for changing 'string' to 'template' when appending the list. What do you know, it worked like a charm. I'm sorry I don't have a definitive reason that it wouldn't pass for you, haha. Here's the code that passed it:
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dict_list):
new_list = []
for item in dict_list:
new_list.append(template.format(**item))
return new_list
Side note: leaving the actual string untouched, you can change the variable name of template to string, or whatever you want for that matter, and it passes as well. You can also leave the dict_list in there, even thought it's not needed, and it passes, as long as you use the original string, which to my eye has no difference to yours (other than the exclamation mark that I added.) Now I'm a bit perplexed, I'll let you know if I figure it out, haha.
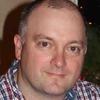
jonlunsford
15,480 PointsBrian:
This looks like solid code to me, so I'm not sure why it's not letting you through. Sometimes the code challenge machine is a little temperamental! I would try removing your dict_list = [ ... ] and try again. It could be the challenge doesn't know what this additional dictionary variable is.