Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial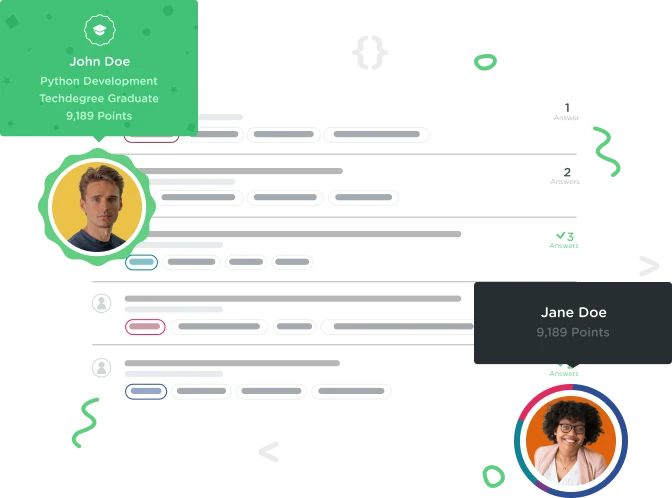

thomas2017
1,342 PointsPython Collections: Removing Items from a List Challenge Task 2
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.insert(0, messy_list.pop(3))
for x in messy_list.copy():
if isinstance(x, (bool, str, list)):
messy_list.remove(x)
Hello, can someone please tell me why this isn't working. In another post on the forum about this page that states that I shouldn't mess with the list directly as it cause the order of the index to get mess up. So I did it with a copy of the list but that didn't seem to fix it.
3 Answers
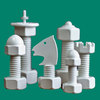
Steven Parker
241,956 PointsList objects don't have a "copy" method. Perhaps you're thinking of dictionaries.
But you can make a copy using a slice with no parameters. If you do that instead, your code should pass the challenge:
for x in messy_list[:]:

thomas2017
1,342 PointsI'm not sure if if it does or not.
I just saw someone else post the answer.
https://teamtreehouse.com/community/python-collections-removing-items-from-a-list-challenge-task-2-2
-Answered by Chris Freeman "Using your third code attempt, It can pass the challenge if you make a copy of messy_list for the for loop. This way, the deletions do not alter the indexes of the copy."
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.insert(0, messy_list.pop(3))
for item in messy_list.copy(): # <-- iterate over a copy of messy_list
if type(item) != int:
print(item)
del messy_list[messy_list.index(item)]
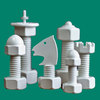
Steven Parker
241,956 PointsThat code also seems to be trying to use a "copy" method on a list. I would not expect that to work, either.
But I added a suggestion to my original answer.

Bob Pickles
1,363 PointsI didn't think about copying it. I'm gonna to back and try it. I ended up doing it with a list comprehension (although it doesn't use remove, it just creates a new list with only the ints)
Adding here for completeness: messy_list = [x for x in messy_list if type(x) is int
Also, from what I understand (and i'd like an actual python dev to chime in on this). According to the pep8 standard, you should use is not instead of != against singletons like "None" (which i assume in this case int is)
Steven Parker
241,956 PointsSteven Parker
241,956 PointsOdd, I did find "copy" listed in the documentation. But when I tried it in my Python, I got "AttributeError: 'list' object has no attribute 'copy'". Maybe I just have a old version and this is something new.