Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial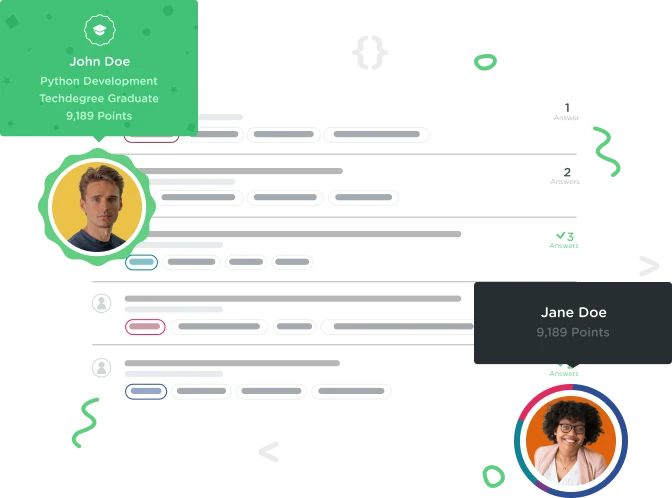

Gilbert Craig
2,102 PointsPython - disemvowel : .replace works in workspaces but again gets the Bummer response. Any help appreciated
I am stumped with why this fails the check
vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word):
# convert argument to list
for char in word:
if char in vowels_list:
word = word.replace(char, '') # for each char in word in vowels list, remove it.
else:
pass
print(word)
get_word = input("What is the word ?:" )
disemvowel(get_word)
8 Answers

Ryan S
27,276 PointsHi Gilbert,
Your logic is good, but there are two reasons why it's not passing the challenge.
The first is that the challenge asks you to return "word", not print.
The second is that you've introduced some extra code after the function that the challenge did not ask for. The code checker will run the code on its own and pass in its own arguments, there is no need to call the function yourself or ask for user input.
If you fix these two things then it should pass.
Hope this helps.

dojewqveaq
11,393 PointsHi Gilbert!
Since strings are immutable. You have to turn it into a list before you do anything with it. The method list() takes care of that. You can assign the new list to a variable, I called it letters_in_word in the code below. Than you can manipulate this list, remove items with the remove() method, and then assign word to the new variable you created. Lastly, you have to return word, and not print it. Check out how I did it:
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
def disemvowel(word):
letters_in_word = list(word)
for letter in word:
if letter in vowels:
letters_in_word.remove(letter)
word = ''.join(letters_in_word)
return word

Gilbert Craig
2,102 PointsHi Ryan/Eric, Many thanks for the responses. I applied both your recommendations but still failing with "getting unexpected letters"

Ryan S
27,276 PointsHey Gilbert,
That is strange. If I copy and paste your code into the challenge (and modify those 2 things), it passes for me:
vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word):
# convert argument to list
for char in word:
if char in vowels_list:
word = word.replace(char, '') # for each char in word in vowels list, remove it.
else:
pass
return word

Gilbert Craig
2,102 PointsMy code is now .. vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word): word_list = list(word) # convert argument to list for char in word_list: if char in vowels_list: word_list.remove(char) # for each char in word in vowels list, remove it.
word = ''.join(word_list) # convert remaining list to word
return(word)

Gilbert Craig
2,102 Points... apologies, new at this ... dont know how to add code to an answer yet

Ryan S
27,276 PointsTake a look at the Markdown Cheatsheet (linked under the text box when submitting answers/comments).
Surround your code with 3 backticks (not apostrophes), and type the name of the programming language after the first 3.
Example:
```python
def disemvowel(word): return word
```
Will show up like:
def disemvowel(word):
return word
Also, to keep the conversations organized, I'd recommend using "Add comment" (when appropriate) instead of responding with Answers for every post. It helps other people follow the conversation by keeping things logical.

Gilbert Craig
2,102 PointsHere is my current stripped down code which still gets the "try again" message ''' vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word): word_list = list(word) for char in word_list: if char in vowels_list: word_list.remove(char)
word = ''.join(word_list)
return word
'''

Gilbert Craig
2,102 PointsThanks Ryan ``` vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word): word_list = list(word) for char in word_list: if char in vowels_list: word_list.remove(char)
word = ''.join(word_list)
return word

Gilbert Craig
2,102 Points.. giving up now .. batsticks have defeated me .. thanks for your help though

Gilbert Craig
2,102 Pointslast try
vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word):
word_list = list(word)
for char in word_list:
if char in vowels_list:
word_list.remove(char)
word = ''.join(word_list)
return word

Ryan S
27,276 PointsNice!
The issue with this code is that you are modifying the list (removing vowels) while you are looping through it. So when you remove a letter, the index position will shift and if there are 2 vowels in a row, you will skip over the second one.
To fix this, you can loop through "word", but modify "word_list":
vowels_list = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
def disemvowel(word):
word_list = list(word)
for char in word:
if char in vowels_list:
word_list.remove(char)
word = ''.join(word_list)
return word
Also, just to put this out there, your code that you posted in your original question worked fine. It was an interesting way of solving it. The only thing was that it printed instead of returned "word", and you had extra user input and a function call that was interfering with the code checker. But other than that, your logic worked and it passes the challenge.

Gilbert Craig
2,102 PointsGentlemen, I resorted to using a conditional loop based on the length of the argument.
'Congrats' displayed
many thanks for your assistance