Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial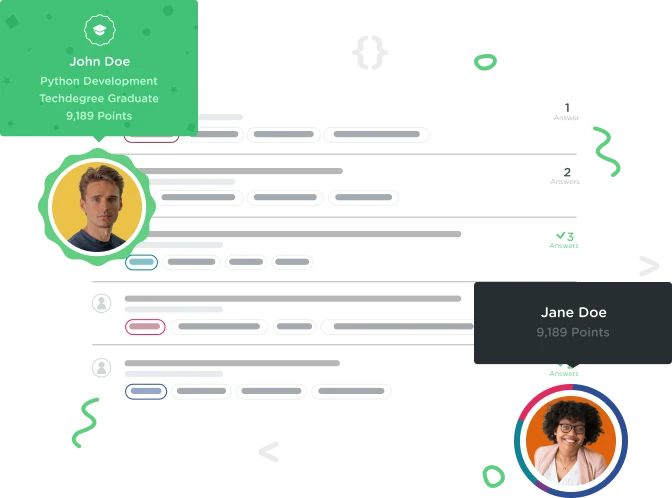
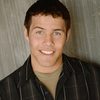
Kyler Smith
10,110 PointsPython function not returning correctly
I'm using IDLE to preview the function I have wrote and in the IDE I am using it will return the correct answer. Any ideas?
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(x):
count = 0
try:
int(x)
returnVal = x * x
except TypeError:
listX = list(x)
for val in listX:
count += 1
returnVal = x * count
except ValueError:
listX = list(x)
for val in listX:
count += 1
returnVal = x * count
return returnVal
3 Answers
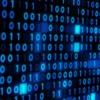
Alexander Davison
65,469 PointsTry this:
def squared(a):
try:
return int(a) ** 2
except ValueError:
return a * len(a)
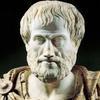
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsHey Kyler,
the challenge wants you to return the argument multiplied by it's length, if it cannot be converted to an integer. If you pass in "tim", the length of the string is three, so you should multiply it by that and return "timtimtim". Currently you convert it into a list which then only has the element "tim" and a length of one, while the actual argument, the string, has a length of three.
Try this instead:
except:
return x * len(x)
Kind Regards, Florian
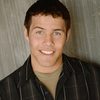
Kyler Smith
10,110 PointsThanks but I altered my code to match your way of doing it as so:
def squared(inputValue): try: int(inputValue) returnVal = inputValue ** 2 except: returnVal = inputValue * len(inputValue)
return returnVal
Still same issue, 'squared' did not return the right answer
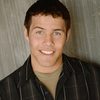
Kyler Smith
10,110 PointsSorry i thought it would format better when I posted it. I will fix soon
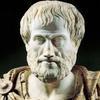
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsSo this is your code now:
def squared(inputValue):
try:
int(inputValue) returnVal = inputValue ** 2
except:
returnVal = inputValue * len(inputValue)
return returnVal
Why do you put 'int(inputValue)' in front of the variable 'returnVal'? It seems you are mixing it up with Java or another type safe language now.
Your code adjusted:
def squared(inputValue):
try:
x = int(inputValue)
returnVal = x ** 2
except:
returnVal = inputValue * len(inputValue)
return returnVal
Kind Regards, Florian