Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial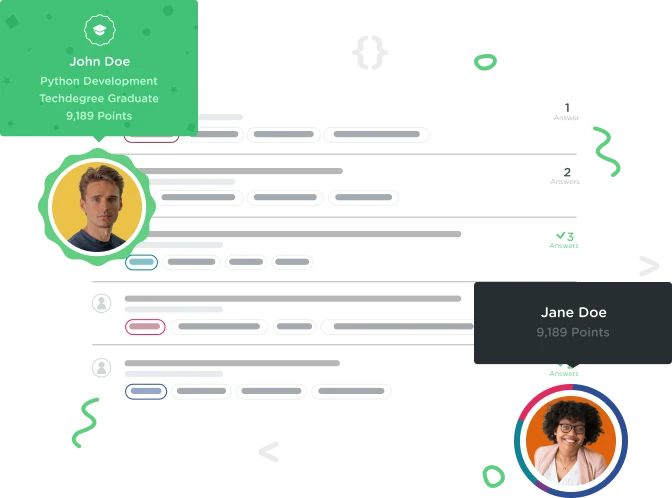

shunjianlee
1,045 Points[Question: How to grey out and disable the button in android studio?]
Good day everyone, i am currently doing a office clerk check in apps. I am struggling to finding the solution how to disable grey out "check in" button after press once and only press "check out" button then "check in" button will return to normal color.
Main activity java:
package ibm.css_example;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.webkit.WebChromeClient;
import android.webkit.WebView;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
int flag=1;
Button button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
WebView webView = (WebView)findViewById(R.id.webView);
webView.getSettings().setJavaScriptEnabled(true);
webView.setWebChromeClient(new WebChromeClient());
webView.loadUrl("file:///android_asset/logo_example_web/index.html");
Button button = (Button)findViewById(R.id.button2);
button.setEnabled(false);
button.setBackgroundResource(R.drawable.);
}
@Override
public void onClick(View v)
{
if(flag)
{
button.setEnabled(false);
Log.d("ins", "called");
}
flag=0;
}
}
activity main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="ibm.css_example.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:id="@+id/textView" />
<WebView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/webView"
android:layout_below="@+id/textView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginLeft="25dp"
android:layout_marginStart="25dp"
android:layout_marginTop="319dp" />
<Button
android:text="@string/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/textView"
android:layout_toLeftOf="@+id/button"
android:layout_toStartOf="@+id/checkin"
android:layout_marginTop="73dp"
android:id="@+id/button2"
/>
<Button
android:text="Check Out"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/checkout"
android:layout_alignBaseline="@+id/button2"
android:layout_alignBottom="@+id/button2"
android:layout_toRightOf="@+id/button"
android:layout_toEndOf="@+id/button" />
</RelativeLayout>
1 Answer

J.D. Sandifer
18,813 PointsHi, shunjianlee. It looks like there are a few things to clean up, but I think the main issue is using an int for your flag variable. Try using a boolean instead. Start with it true and set it to false after the button is pressed.
It also looks like you're starting with the button not enabled:
Button button = (Button)findViewById(R.id.button2);
button.setEnabled(false); // probably want this to be setting it to true
In addition, I highly recommend you think a little more about good names for your variables. Names like "button" and "flag" make it easy to forget what they refer to when you get lots of code going. ("button" in your code refers to a button with the id "button2". Seems super confusing...)
Try more descriptive names like checkIn and checkInEnabled (for the flag). Your second button has the id "checkout" - use more descriptive id's and names like that for everything. It will help you keep things straight and help anyone else looking at your code!