Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial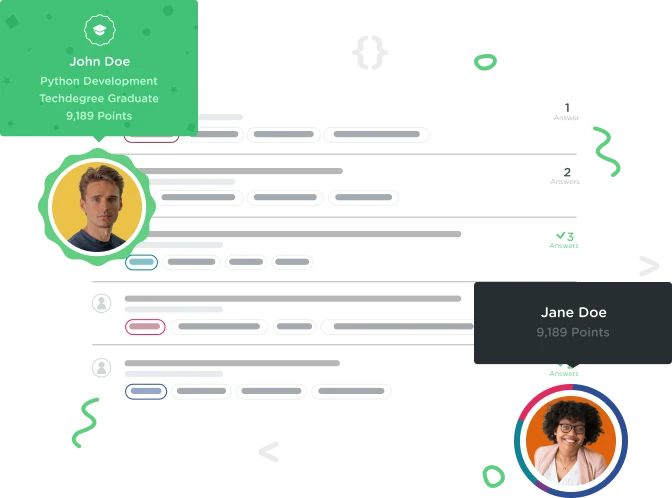

Jonathon Lumpkin
3,806 PointsQuiz answers not formatting correctly
My solution to the quiz...
var questions = [
['How many feet are in a mile?', 5280],
['How many pounds are in a ton?', 2000],
['How many planets are in our solar system?', 9]
];
var answers;
var answer;
var correctAnswer = 0;
var incorrectAnswer = 0;
var successfulTries = [];
var unsuccessfulTries = [];
for (var i = 0; i < questions.length; i ++) {
var response = prompt(questions[i][0]);
answer = questions[i][1];
if (response === answer) {
alert('Correct!');
correctAnswer += 1;
successfulTries.push(questions[i]);
} else {
alert('That\'s not right!');
incorrectAnswer += 1;
unsuccessfulTries.push(questions[i]);
}
}
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function printResults(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i ++) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
html = "You got " + correctAnswer + " question(s) right.";
html += '<h2>You got these questions correct:</h2>';
html += printResults(successfulTries);
html += '<h2>You got these questions wrong:</h2>';
html += printResults(unsuccessfulTries);
print(html);
The problem I'm having is that when it prints the results, the questions show up as "Question?,Answer." There are no spaces, and I can't figure out where that comma is coming from.
2 Answers
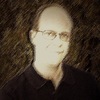
Jason Anders
Treehouse Moderator 145,860 PointsHey Jonathon,
It's printing no spaces and a comma, because you are pushing both the question and the answer into your new array, so when you print it, the program is printing out the array. Just add the [0] to your push and it will only print out the questions that are right or wrong.
for (var i = 0; i < questions.length; i ++) {
var response = prompt(questions[i][0]);
answer = questions[i][1];
if (response === answer) {
alert('Correct!');
correctAnswer += 1;
successfulTries.push(questions[i][0]); //added [0]
} else {
alert('That\'s not right!');
incorrectAnswer += 1;
unsuccessfulTries.push(questions[i][0]); //added [0]
}
}
Also, I noticed that I was getting all the questions wrong. You need to add parseInt to your conditional statement. By default, any prompt will return a string. Because you are using === you are checking for "strict equality" and a string never equals a number. You could change to a "lenient equality" (==), but it's usually safer to use ===. In this case, you just need to change your input string to a number, because your answers are numbers.
if (parseInt(response) === answer) { //added parseInt() to change string response to a number
alert('Correct!');
correctAnswer += 1;
successfulTries.push(questions[i])
}
Hope that all makes sense and helps out.
Keep Coding! :)

Jonathon Lumpkin
3,806 PointsAh, thanks. I couldn't figure out if there was something formatwise I was supposed to be adding or what.
I was planning to add the parseInt, I just hadn't put it in yet, since I know all the answers are integers it hadn't been a problem yet :) I'll add that in now.