Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial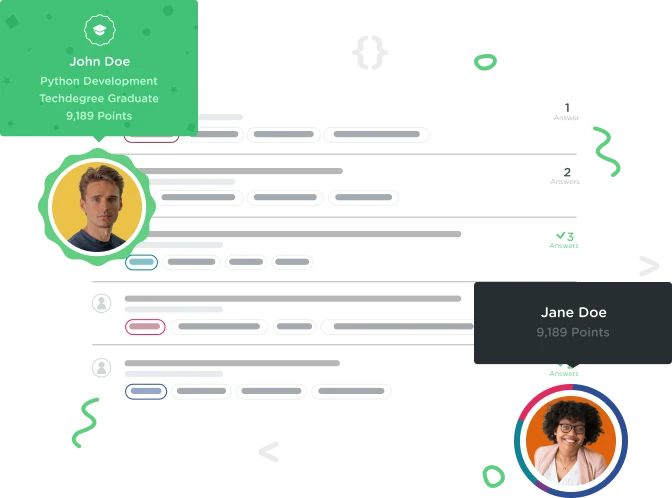

Patrick Rice
11,017 PointsQuiz challenge code feedback
The first program I have written in JavaScript practicing everything I have learned up to this point. When I tested my code it works fine, just looking for any feedback regarding format, technique, opportunities, or best practices. Thanks
/*
This quiz will ask questions about standard
timeframes regarding days, weeks, hours, minutes, seconds.
*/
// Explain the basics of the quiz
var quizStart = alert("The following quiz is 5 questions. Each question you
get right is worth 1 point, for a total of 5 points. Are you ready to play? Click OK!");
var score = 0;
/* prompt user for answer, convert answer to integer, add 1 point to score
for correct answers, and leave score the same for incorrect answers. Display score.
*/
var days = prompt("How many days are there in a week?");
var daysInt = parseInt(days);
if ( daysInt === 7 ) {
score += 1
alert("That is correct! Score = " + score);
} else {
alert("Sorry, that is incorrect! Score = " + score);
}
var weeks = prompt("How many weeks are in a year?");
var weeksInt = parseInt(weeks);
if ( weeksInt === 52 ) {
score += 1
alert("That is correct! Score = " + score);
} else {
alert("Sorry, that is incorrect! Score = " + score);
}
var hours = prompt("How many hours are in a day?");
var hoursInt = parseInt(hours);
if ( hoursInt === 24 ) {
score += 1
alert("That is correct! Score = " + score);
} else {
alert("Sorry, that is incorrect! Score = " + score);
}
var minutes = prompt("How many minutes are in an hour?");
var minutesInt = parseInt(minutes);
if ( minutesInt === 60 ) {
score += 1
alert("That is correct! Score = " + score);
} else {
alert("Sorry, that is incorrect! Score = " + score);
}
var seconds = prompt("How many seconds in a minutes?");
var secondsInt = parseInt(seconds);
if ( secondsInt === 60 ) {
score += 1
alert("That is correct! Score = " + score);
} else {
alert("Sorry, that is incorrect! Score = " + score);
}
// determine rank of the player
var trophy
if ( score === 5 ) {
trophy = "Gold Crown";
} else if ( score > 2 && score < 5 ) {
trophy = "Silver Crown";
} else if ( score > 0 && score < 3 ) {
trophy = "Bronze Crown";
} else {
trophy = "None!";
}
// provide final message showing score and rank
var gameOver = alert("You have finished the quiz. Your final score is " + score + ". Achievement: " + trophy);
1 Answer
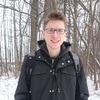
Mike Rose
21,228 PointsHi Patrick,
Looks good. I have a couple suggestions, if you like.
When using parseInt
it's always good to specify a radix as a second argument. You can read about them here:
https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/parseInt
Also, if you wanted to reduce the amount of code, you might consider putting the questions into a function. If you haven't covered them yet, it might seem a bit confusing, but essentially it would be something like this..
// this function takes three arguments (interval, unitOfTime, answer)
// and uses them throughout the block of code, kinda like variables.
function askQuestion(interval, unitOfTime, answer) {
var input = prompt('How many ' + interval + ' are in a ' + unitOfTime + '?');
var inputInt= parseInt(input, 10); // using radix of 10 here
if ( inputInt === answer ) {
score += 1
alert("That is correct! Score = " + score);
} else {
alert("Sorry, that is incorrect! Score = " + score);
}
}
askQuestion('seconds', 'minute', 60); // here we pass in three arguments and 'call' the function.
askQuestion('days', 'week', 7); // here we call it again with three different arguments
askQuestion('weeks', 'year', 52); // etc..
Patrick Rice
11,017 PointsPatrick Rice
11,017 PointsNice! Thanks for your feedback. Yes - haven't got there yet (looking forward to it) but your code makes sense. I will check out the resource you linked to.