Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial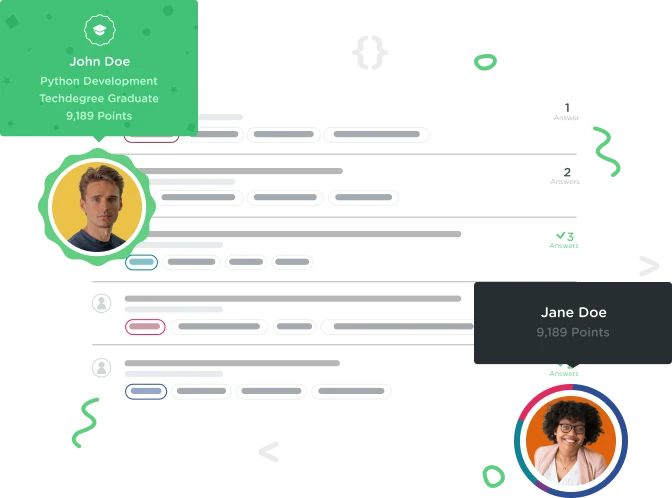

Aryan Monga
4,061 PointsQuiz question!
How do i do and what do i do? This is getting messy! ;(
import random
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
def random_item(a):
2 Answers
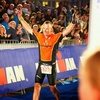
Steve Hunter
57,712 PointsHi Aryan,
Here, you need to create a new function that takes an iterable as a parameter. Let's assume that's a string because that's easy to visualize. And we'll use "TREEHOUSE" as the challenge uses that as an example. However, that string is passed into the function - let's get that set up first:
def random_item(iterable):
You've correctly identified that we need to import random
so let's add that too:
import random
def random_item(iterable):
That's where you made it to. We're going to use random
to pick a number that's between zero and the length of the iterable passed in. Let's create a store for that value and use the len()
method to get the number we need. Pass the iterable into the len()
method - it'll return the number of characters in the string, in our case.:
import random
def random_item(iterable):
length = len(iterable)
What we want to do now is to generate a random number between zero (the first index element of the iterable) and the length of the iterable, minus one (because we started at zero). We'll store that in another variable - I called it index
. The random.randint()
method generates a number for us. It takes two parameters, the lower & upper bounds. We know those already; zero and length - 1
. That gives us:
import random
def random_item(iterable):
length = len(iterable)
index = random.randint(0, length - 1)
We then want to return the character at index
of iterable
. Use square brackets:
import random
def random_item(iterable):
length = len(iterable)
index = random.randint(0, length - 1)
return iterable[index]
We could tidy that up a bit and not have the index
variable; just return the expression inside the square brackets:
import random
def random_item(iterable):
length = len(iterable)
return iterable[random.randint(0, length - 1)]
I hope that helps, and makes sense.
Steve.

Keenan Johnson
13,841 PointsYou're off to a good start with the code you have! From there, use the random.randint() function with the given from the text parameters (0 and the length of the parameter - 1) and storing that result in a variable. Use that variable to access the item at that position.
Aryan Monga
4,061 PointsAryan Monga
4,061 PointsPlease give me some instructions!