Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial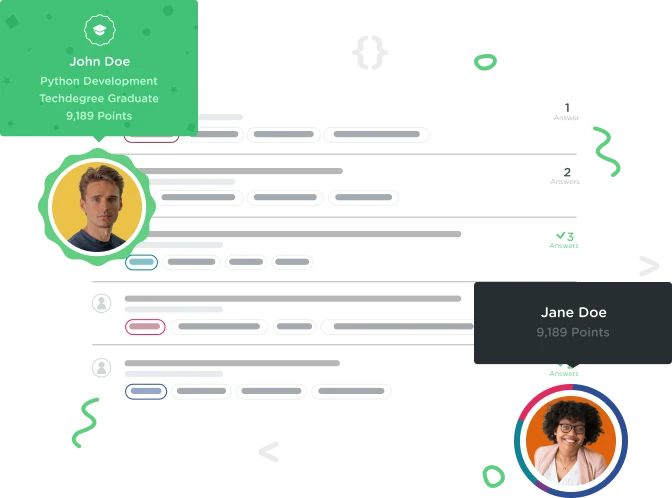
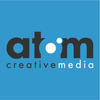
Alex Connor
2,809 PointsRandom Number Challenge - My Solution
This took me a while, as math isn't my strong suit. I had to look up a couple of things that haven't yet been covered in these lessons, but I figure that's sort of the point as well, right?
Anyway. I opted for the extra challenge to input 2 numbers.
var firstNumber = prompt("Give us a number...");
var secondNumber = prompt("And another...");
firstNumber = parseInt(firstNumber);
secondNumber = parseInt(secondNumber);
I wasn't sure if it was important to first figure out which is the higher number, otherwise we might end up with negative values. So I found Math.min
and Math.max
.
//which is lower?
var lowestNumber = Math.min(firstNumber,secondNumber);
//which is higher?
var highestNumber = Math.max(firstNumber,secondNumber);
To test this was working, I used console.log
.
console.log("Lowest number is: " + lowestNumber);
console.log("Highest number is: " + highestNumber);
This was where it got tricky for me. I basically had to look this up, as I couldn't brain it with my own brain.
var randomNumber = Math.floor(Math.random() * (highestNumber - lowestNumber + 1 )) + lowestNumber;
So, basically, generate the random number using Math.random
, and multiply that by the highest number minus the lowest number + 1. Then add the lowest number, to ensure that the final result doesn't go under the lowest given value.
So it would look like this:
random Number * (high - low + 1) + low
or, as a simple example...
0.5 * (100 - 50 + 1) + 50
further broken down as...
0.5 * 51 + 50 = 75.5
Then Math.floor
that sucker, giving us 75.
Interestingly, I had to store this result in its own variable (randomNumber
), since if I placed the math statement directly in the alert it was treated as a string, appending onto the end of the alert message.
alert("Here's a number between " + lowestNumber + " and " + highestNumber + ": " + randomNumber);
Not sure why this is, but it was probably a good thing anyway as it helped keep my code a bit more manageable and readable.
1 Answer
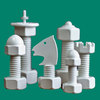
Steven Parker
231,275 PointsUsing "max" and "min" was a nice touch, most folks just ask specifically for a low number and then a higher one.
Your string issue might have been caused by putting quotes around your expression. If that's not it, and you'd like help figuring it out, show the actual code that was involved so we can take a look.